Question
Improve the card program. You can now specify the number of players plus the number of cards to draw for each player. Your JAVA program
Improve the card program. You can now specify the number of players plus the number of cards to draw for each player. Your JAVA program will then draw that many cards for that many players, then display the suits and values of all the cards drawn. Assume you are drawing without replacement, which means that once a card is drawn it cannot be drawn again. There are 52 cards in a deck. A deck consists of four suits (Diamonds, Hearts, Clubs and Spades). Each suit contains 13 values (Ace, 2, 3, 4, 5, 6, 7, 8, 9, 10, Jack, Queen and King).
-Create a method that accepts two integer parameters and has a 2-dimensional String array (or String[][]) return type. The method will randomly draw cards from a simulated deck and add them to a string array that is returned after cards are drawn
. -Create a method that accepts one String array (or String[][]) parameter and has a void return type. The method will perform the System.out.println() function on all elements of the String array. It will also indicate which cards belong to which players like in the example run. -The main method of the program will receive and validate user input. If the input is valid, it will then call both methods.
-User input will be the parameter for the method 1 call -The returned 2d String array from method 1 will be the parameter for the method 2 call -You may choose to use either the console or the Java dialog boxes for data input and output.
-If the number of cards to draw is zero or a negative or if the total number of cards would exceed 52, your program should report the error and exit the program: you can do this with System.exit(0)
Example Run
Players?
2
cards?
26
Player 1 is holding:
Eight of Clubs
Four of Spades
Eight of Hearts
King of Spades
Six of Diamonds
Four of Hearts
Two of Spades
Jack of Diamonds
King of Hearts
Two of Diamonds
Five of Hearts
Three of Spades
Five of Clubs
Nine of Spades
Two of Hearts
Seven of Hearts
Six of Spades
Seven of Diamonds
Three of Clubs
Four of Diamonds
Queen of Spades
Queen of Clubs
Five of Spades
Ace of Hearts
Five of Diamonds
Jack of Hearts
Player 2 is holding:
Eight of Diamonds
Ace of Spades
Nine of Clubs
Three of Diamonds
Eight of Spades
Ace of Clubs
Seven of Clubs
King of Diamonds
Jack of Spades
Ten of Clubs
Nine of Hearts
Jack of Clubs
Ten of Diamonds
Queen of Diamonds
Four of Clubs
Three of Hearts
Seven of Spades
Ten of Spades
King of Clubs
Queen of Hearts
Six of Clubs
Ten of Hearts
Six of Hearts
Two of Clubs
Nine of Diamonds
Ace of Diamonds
THIS IS AN ADD-ON TO A PREVIOUS ASSIGNMENT. I ALREADY HAVE THE CODE, I JUST WANT TO INTEGRATE THIS INTO THE EXISTING CODE:
import java.util.*;
class Card {
// String rank, suit;
public Card(String r, String s) {
rank = r;
suit = s;
}
public String toString() {
return rank + " of " + suit;
}
}
public class Cards {
public Card[] cards;
public static final String[] suits = {"Diamonds", "Hearts", "Clubs", "Spades"};
public static final String[] ranks = {"Ace", "2", "3", "4", "5", "6", "7", "8","9", "10", "Jack", "Queen", "King"};
public Cards() {
cards = new Card[52]; //DECLARE K
int k = 0;
for(int i=0; i<4; i++) {
for(int j=0; j<13; j++) {
cards[k ++] = new Card(ranks[j], suits[i]);
}
}
}
public String[] selectCards(int n) {
String[] c = new String[n]; //GENERATE RANDOM NUMBER Random rand = new Random();
for(int i=0; i int select; select = rand.nextInt(52); c[i] = cards[select].toString(); } return c; } public void displayDrawnCards(String[] c) { for(int i=0; i System.out.println((i+1) + ") " + c[i]); } } //MAIN METHOD public static void main(String[] args) { Cards c = new Cards(); Scanner sc = new Scanner(System.in); // ASK USER FOR NUMBER OF CARDS System.out.print("Number of cards : "); int n = sc.nextInt(); //SHOW ERROR/INVALID IF USER INPUTS NEGATIVE NUMBERS if(n <= 0 ) { System.out.println("Invalid"); System.exit(0); } c.displayDrawnCards(c.selectCards(n)); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
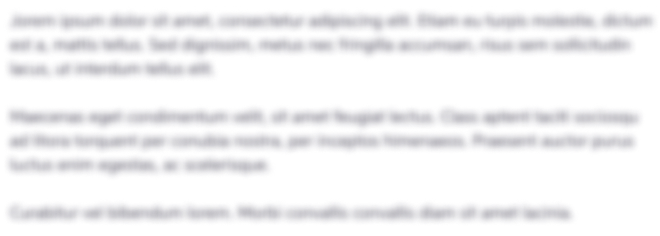
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started