Question
In Assignment 19 I created a simple ToDo list that would hold structs that contained information about each item that would be in the list..
In Assignment 19 I created a simple ToDo list that would hold structs that contained information about each item that would be in the list.. For this assignment I need to take assingment 19 and create a class out of it.
The class should be called ToDoList. The definition for the ToDoList should be in the header file. The variables that make up the data section should be put in the private area of the class. The prototypes for the interface functions should be in the public area of the class.
Data Section
You can easily identify the variables that make up the data section from the fact that all of the functions use these variables. You should remember from the lecture material on classes that the data section should be the private section of the class. You should also note that if a variable should not have direct access to it then it should be in the private section. What I mean here is that if a person can directly access a variable from a function outside of the class and if modifying this variable can cause unknown problems to the operation of your class then it should be in the private section of the class.
Prototype Functions
All of the function prototypes should also go in the class. If a function is an interface into the class then it should go in the public section. For this assignment all functions are interfaces so all prototypes should go in the public section of the class.
In the previous step you prototyped all of the functions and put them in the public section of the class definition. The bodies for these functions should go in ToDoList.cpp. Don't forget to use the scope resolution operator to relate the function bodies to the prototypes in the class definition.
Here are the classes from assingment 19.
main.cpp
#include
#include "ToDo.h"
using namespace std;
int main()
{
cout << "Enter a number" << endl;
int num;
cin >> num;
int n = 1;
for (int i = num; i > 0; i)
{
n *= i;
}
cout << num << "! is " << n << endl;
return 0;
}
void printMenu()
{
cout << "1. Add Todo By Struct" << endl;
cout << "2. Add Todo By description" << endl;
cout << "3. Get Next Todo " << endl;
}
ToDo.cpp
#include
#include "ToDo.h"
using namespace std;
MyToDo ToDoList[MAXLIST];
int head = 0, tail = -1;
bool addToList(const MyToDo &td)
{
if(head < MAXLIST )
{
ToDoList[head] = td;
head++;
return true;
}
return false;
}
bool addToList(string description, string date, int priority)
{
MyToDo td;
td.description = description;
td.dueDate = date;
td.priority = priority;
return addToList(td);
}
bool getNextItem(MyToDo &td)
{
if(tail >= 0)
{
if(tail >= MAXLIST)
tail = 0;
td = ToDoList[tail];
tail++;
return true;
}
return false;
}
bool getNextItem(string &description, string &date, int &priority)
{
MyToDo tmp;
bool status = getNextItem(tmp);
if(status == true)
{
description = tmp.description;
date = tmp.dueDate;
priority = tmp.priority;
}
return status;
}
bool getByPriority(MyToDo list[], int priority)
{
if(tail < 0)
return false;
int count = 0;
for(int i = 0 ; i < head; i++)
{
if(ToDoList[i].priority == priority)
{
list[count] = ToDoList[i];
count++;
}
}
if(count > 0)
return true;
return false;
}
void printToDo()
{
for(int i = 0; i < head; i++)
{
cout << "Description: " << mtd[i].description << endl;
cout << "Due Date: " << mtd[i].dueDate << endl;
cout << "Priority : " << mtd[i].priority << endl;
}
}
ToDo.h
#ifndef TODO
#define TODO
#include
using std::string;
const int MAXLIST = 10;
struct MyToDo
{
string description;
string dueDate;
int priority;
};
bool addToList(const MyToDo &td);
bool addToList(string description, string date, int priority);
bool getNextItem(MyToDo &td);
bool getNextItem(string &description, string &date, int &priority);
bool getByPriority(MyToDo list[], int priority);
void printToDo();
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
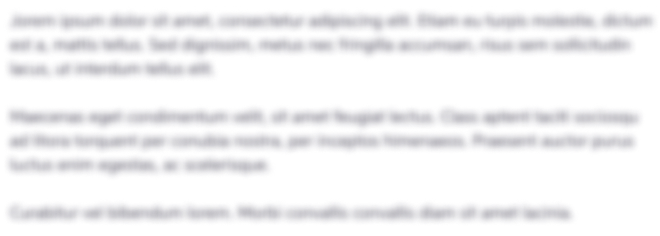
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started