Question
In C# Add a RemoveAll method to OurStack class. It removes all occurrences of a value from a stack. Use the Equals method and not
In C#
Add a RemoveAll method to OurStack class. It removes all occurrences of a value from a stack. Use the Equals method and not ==.
Example: Stack = a, b, a, c, d, a, e Remove('a') Stack = b, c, d, e
OurStack Class:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
class OurStack { private class Node { public T Data { get; set; } public Node Next { get; set; } public Node(T d = default(T), Node node = null) { Data = d; Next = node; } }
private Node top;
public OurStack() { top = null; }
public void Clear() { top = null; }
public bool IsEmpty() { return top == null; }
public void Push(T value) { top = new Node(value, top); }
public T Pop() { if (IsEmpty() == true) throw new ApplicationException("Error: can't pop an empty stack"); T removedData = top.Data; top = top.Next; return removedData; }
public T Peek() { if (IsEmpty() == true) throw new ApplicationException("Error: can't peek at an empty stack"); return top.Data; } public int Count { get { int count = 0; Node pTmp = top; while (pTmp != null) { count++; pTmp = pTmp.Next; } return count; } }
public override string ToString() { if (IsEmpty() == true) return string.Empty;
StringBuilder returnString = new StringBuilder(); Node pTmp = top; while (pTmp != null) { if (returnString.Length > 0) returnString.Append(":"); returnString.Append(pTmp.Data); pTmp = pTmp.Next; } return returnString.ToString(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
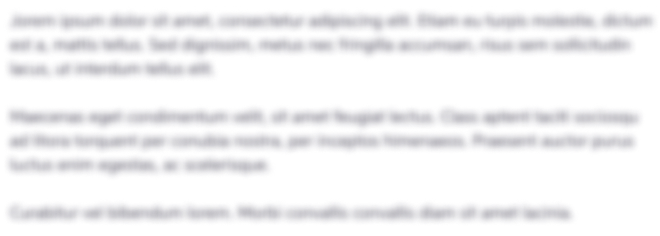
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started