Question
In C++ code, complete the program below labeled: Practice Problem 2. It must use the starting code provided at the bottom and have comments explaining
In C++ code, complete the program below labeled: Practice Problem 2. It must use the starting code provided at the bottom and have comments explaining what is happening in the program.
Practice Problem 1. Redefine CDAccount from Display 10.1 so that it is a class rather than a structure. Use the same member variables as in Display 10.1 but make them private. Include member functions for each of the following: one to return the initial balance, one to return the balance at maturity, one to return the interest rate, and one to return the term. Include a constructor that sets all of the member variables to any specified values, as well as a default constructor. Embed your class definition in a test program.
Practice Problem 2. Redo your definition of the class CDAccount from Practice Program 1 so that it has the same interface but a different implementation. The new implementation is in many ways similar to the second implementation for the class BankAccount given in Display 10.7. Your new implementation for the class CDAccount will record the balance as two values of type int: one for the dollars and one for the cents. The member variable for the interest rate will store the interest rate as a fraction rather than as a percentage. For example, an interest rate of 4.3% will be stored as the value 0.043 of type double. Store the term in the same way as in Display 10.1.
Display 10.1 A Structure Definition (part 1 of 2)
1 //Program to demonstrate the CDAccount structure type.
2 #include
3 using namespace std;
4 //Structure for a bank certificate of deposit:
5 struct CDAccount
6 {
7 double balance;
8 double interest_rate;
9 int term; //months until maturity
10 };
11
12
13 void get_data(CDAccount& the_account);
14 //Postcondition: the_account.balance and the_account.interest_rate
15 //have been given values that the user entered at the keyboard.
16
17
18 int main( )
19 {
20 CDAccount account;
21 get_data(account);
22
23 double rate_fraction, interest;
24 rate_fraction = account.interest_rate / 100.0;
25 interest = account.balance * rate_fraction * (account.term / 12.0);
26 account.balance = account.balance + interest;
27
28 cout.setf(ios::fixed);
29 cout.setf(ios::showpoint);
30 cout.precision(2);
31 cout << "When your CD matures in "
32 << account.term << " months, "
33 << "it will have a balance of $"
34 << account.balance << endl;
35 return 0;
36 }
37
38 //Uses iostream:
39 void get_data(CDAccount& the_account)
40 {
41 cout << "Enter account balance: $";
42 cin >> the_account.balance;
43 cout << "Enter account interest rate: ";
44 cin >> the_account.interest_rate;
45 cout << "Enter the number of months until maturity "
46 << "(must be 12 or fewer months): ";
47 cin >> the_account.term;
48 }
(continued)
Display 10.1 A Structure Definition (part 2 of 2)
Sample Dialogue
Enter account balance: $100.00
Enter account interest rate: 10.0
Enter the number of months until maturity
(must be 12 or fewer months): 6
When your CD matures in 6 months,
it will have a balance of $105.00
Display 10.7 Alternative BankAccount Class Implementation (part 1 of 4)
1 //Demonstrates an alternative implementation of the class BankAccount.
2 #include
3 #include
4 using namespace std; .
5 //Class for a bank account:
6 class BankAccount
7 {
8 public:
9 BankAccount(int dollars, int cents, double rate);
10 //Initializes the account balance to $dollars.cents and
11 //initializes the interest rate to rate percent.
12 BankAccount(int dollars, double rate);
13 //Initializes the account balance to $dollars.00 and
14 //initializes the interest rate to rate percent.
15 BankAccount( );
16 //Initializes the account balance to $0.00 and the
17 //interest rate to 0.0%.
18 void set(int dollars, int cents, double rate);
19 //Postcondition: The account balance has been set to $dollars.cents;
20 //The interest rate has been set to rate percent.
21 void set(int dollars, double rate);
22 //Postcondition: The account balance has been set to $dollars.00.
23 //The interest rate has been set to rate percent.
24 void update( );
25 //Postcondition: One year of simple interest has been
26 //added to the account balance.
27 double get_balance( );
28 //Returns the current account balance.
29 double get_rate( );
30 //Returns the current account interest rate as a percentage.
31 void output(ostream& outs);
32 //Precondition: If outs is a file output stream, then
33 //outs has already been connected to a file.
34 //Postcondition: Account balance and interest rate
35 //have been written to the stream outs.
36 private:
37 int dollars_part;
38 int cents_part;
39 double interest_rate;
40 //Expressed as a fraction, for example, 0.057 for 5.7%
(continued)
Notice that the public members of
BankAccount look and behave
exactly the same as in Display 10.6
Display 10.7 Alternative BankAccount Class Implementation (part 2 of 4)
41 double fraction(double percent);
42 //Converts a percentage to a fraction. For example, fraction(50.3)
43 //returns 0.503.
44 double percent(double fraction_value);
45 //Converts a fraction to a percentage. For example, percent(0.503)
46 //returns 50.3.
47 };
48 int main( )
49 {
50 BankAccount account1(100, 2.3), account2;
51
52 cout << "account1 initialized as follows: ";
53 account1.output(cout);
54 cout << "account2 initialized as follows: ";
55 account2.output(cout);
56
57 account1 = BankAccount(999, 99, 5.5);
58 cout << "account1 reset to the following: ";
59 account1.output(cout);
60 return 0;
61 }
62 BankAccount::BankAccount(int dollars, int cents, double rate)
63 {
64 if ((dollars < 0) || (cents < 0) || (rate < 0))
65 {
66 cout << "Illegal values for money or interest rate. ";
67 exit(1);
68 }
69 dollars_part = dollars;
70 cents_part = cents;
71 interest_rate = fraction(rate);
72 }
73 BankAccount::BankAccount(int dollars, double rate)
74 {
75 if ((dollars < 0) || (rate < 0))
76 {
77 cout << "Illegal values for money or interest rate. ";
78 exit(1);
79 }
80 dollars_part = dollars;
81 cents_part = 0;
82 interest_rate = fraction(rate);
83 }
84 BankAccount::BankAccount( ) : dollars_part(0), cents_part(0), interest_rate(0.0)
85
(continued)
New
Since the body of main is
identical to that in Display 10.6 ,
the screen output is also identical
to that in Display 10.6
In the old implementation of this ADT, the private
member function fraction was used in the
definition of update. In this implementation,
fraction is instead used in the definition of
constructors and in the set function.
Display 10.7 Alternative BankAccount Class Implementation (part 3 of 4)
86 {
87 //Body intentionally empty.
88 }
89 double BankAccount::fraction(double percent_value)
90 {
91 return (percent_value/100.0);
92 }
93 //Uses cmath:
94 void BankAccount::update( )
95 {
96 double balance = get_balance( );
97 balance = balance + interest_rate * balance;
98 dollars_part = static_cast<int>(floor(balance));
99 cents_part = static_cast<int>(floor((balance - dollars_part)*100));
100 }
101 double BankAccount::get_balance( )
102 {
103 return (dollars_part + 0.01 * cents_part);
104 }
105 double BankAccount::percent(double fraction_value)
106 {
107 return (fraction_value * 100);
108 }
109 double BankAccount::get_rate( )
110 {
111 return percent(interest_rate);
112 }
113 //Uses iostream:
114 void BankAccount::output(ostream& outs)
115 {
116 outs.setf(ios::fixed);
117 outs.setf(ios::showpoint);
118 outs.precision(2);
119 outs << "Account balance $" << get_balance( ) << endl;
120 outs << "Interest rate "<< get_rate( ) << "%" << endl;
121 }
122 void BankAccount::set(int dollars, int cents, double rate)
123 {
124 if ((dollars < 0) || (cents < 0) || (rate < 0))
125 {
126 cout << "Illegal values for money or interest rate. ";
127 exit(1);
128 }
(continued)
Display 10.7 Alternative BankAccount Class Implementation (part 4 of 4)
129 dollars_part = dollars;
130 cents_part = cents;
131 interest_rate = fraction(rate);
132 }
133 void BankAccount::set(int dollars, double rate)
134 {
135 if ((dollars < 0) || (rate < 0))
136 {
137 cout << "Illegal values for money or interest rate. ";
138 exit(1);
139 }
140 dollars_part = dollars;
141 interest_rate = fraction(rate);
142 }
Starting Code:
#include
class CDAccount { public: CDAccount(); CDAccount(double new_balance, double new_interest_rate, int new_term); double get_initial_balance() const; double get_balance_at_maturity() const; double get_interest_rate() const; int get_term() const; void input(istream& in); void output(ostream& out); private: int dollar; int cent; double interest_rate; int term; // months until maturity };
int main() { // You implement the test code here // notice that user will only enter balance as a double value // You may implement it as a menu oriented testing program // which be able to test constructors, methods of CDAccount class }
// Implement the class definition here. Notice that the interfaces are the // same as in Programming Project 1. However, the implementation will be // different since the private information balance is stored differently // for example, the input function will only read the balance, interest rate // and term from the user. So it should be modified as following void CDAccount::input(istream& in) { double balance; in >> balance; // get initial balance from user // convert balance to dollars and cents since this is how balance stored dollar = (int) balance; // dollar is the interger part of balace cent = (int) ((balance - dollar)*100); // cent is the fraction part * 100 in >> interest_rate; // get interest rate from user in >> term; // get term from user }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
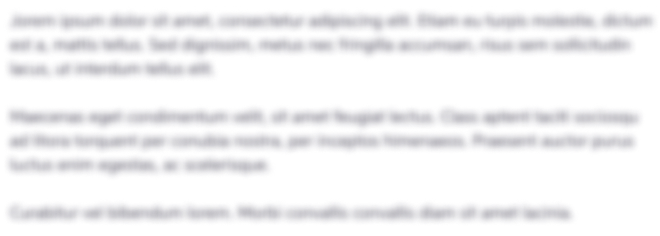
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started