Question
In C++ For this assignment, we will be creating classes that contain shapes described by a series of points. Points will be described by the
In C++
For this assignment, we will be creating classes that contain shapes described by a series of points. Points will be described by the Point structure:
struct Point {
double x, y;
};
Using the point structure, create a class called "Shape". Shape can contain an array or vector of Points. Use the following class definition for the shape class:
class Shape{ private: vector points; public: void addPoint(Point); Point getPoint(int); int getNumPoints(void); };
b. This shape class only contains member function prototypes. You will need to define the member functions themselves. The functions should behave as follows:
* addPoint should push a new point struct to the vector of points * getPoint should retrieve a point at the specified index. Be sure to check for boundary conditions! * getNumPoints should return the current vector length
c. Three files are provided containing a series of points in the format "x, y". Each file has a new point on each new line. When launching your program, add a file name as a command line parameter. The program should open the file, read all the points contained within, then initialize a Shape object containing the points. If no filename is passed to the function, print an error message and exit.
Pointset1.txt | Pointset2.txt | Pointset3.txt |
0,2 2,0 0,2 2,2 | 0.0 2,0 1,1 | 0,0 2,0 0,4 2,4 |
d. Each file contains points which form either a square, triangle, and rectangle. Create a derived class for each shape which uses the "Shape" class as a base. Add a virtual "area" and "perimeter" function to the base the class and implementation for each of these in the derived square, triangle, and rectangle classes. Feel free to add any additional data member objects for these shapes as you find helpful (such as a side length for squares, length and width for rectangles.) Create an overloaded constructor which uses 3 points as input for the triangle class, and 4 points for the square and rectangle.
e. When reading in a set of points from the file, write some code which determines which shape the set of points should belong to (yes, squares are types of rectangles, but if you read points which form a square, use the square class). When the proper shape is determined, initialze that class using those points.
Once you have completed this, call the area and perimeter member fuctions of your classes and print these values to the terminal.
f. Also, Add functionality so that multiple files can be passed as input parameters. Initialize each set of points within the proper shape class. Create an array of Shape pointers which point to each derived class and utilize the virtual area and perimeter functions to retrive the area and perimeter for each shape.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
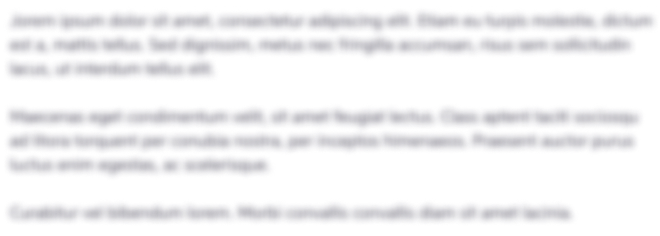
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started