Question
In C++: Goals: Learn how to apply the 3-tier program structure, i.e. CEO, manager and regular employee, to our projects. Learn how to use a
In C++:
Goals:
- Learn how to apply the 3-tier program structure, i.e. CEO, manager and regular employee, to our projects.
- Learn how to use a loop statement to interact with the system user. When the user enters a command, the system responds to the command in a proper way. Should the loop statement be a for loop, while or do-while statement?
- Learn how to initialize data in constructors and release memory in destructor.
- This HW will prepare you for the coming HWs which demonstrates Polymorphism of OOP by using Virtual Functions.
General requirement:
Each .h or .cpp file has to be separated. The five files should be zipped together and submitted.
Project requirements:
Design and implement a system which simulates the speed dial phone system with the following requirements:
- The input file, named phonenumbers.txt, provides at most 999 phone numbers and their corresponding owner names. The three columns in the file are (1) 10-digit phone number, (2) first name, and (3) last name. (We can assume that there is no error in the file.)
- After the system read the data from the input file, the user may interact with the system by entering the following commands:
Command | Action |
Q or q | Quit |
D or d | Display the entire listing in three columns: phone number, first name and last name |
R or r | For survey purpose the system can randomly pick up a phone number in the listing and dial. Only the phone number and its corresponding user last name and first name are displayed. |
An integer | If the integer is in the range, the phone number and its corresponding user last name and first name are displayed. If the number is out of range, a message saying Not available is prompted. |
- How does the use of the Manager class avoid memory leak? Write your answer on the top of the main.cpp file.
Design and implementation:
- The structure resembles the typical industry 3-tier program structure. The three logical entities, i.e. main, PhoneMgr class and Phone class, resemble the three level of a corporate hierarchy, i.e. CEO, manager and other employees. A CEO does not talk to lower-level employees directly. Instead, he talks to the managers and decide what to get done on a higher level, the managers conduct project managements, organize the tasks and works with the engineers to get all the tasks done. (This suggests that we should have three physical .cpp files, i.e. main.cpp, PhoneMgr.cpp, and Phone.cpp, in the system.)
- An array containing Phone pointers, not Phone objects, will be created to simulate the speed-dial system. It is obvious that PhoneMgr class must own this array. The array must be initialized with NULL or populated with values once a PhoneMgr object is constructed. A good place to complete these works is in PhoneMgr constructor. The problem is that if the array is a local variable of the constructor, then how can other PhoneMgr functions, e.g. Display(), access it? Since the array has to be shared by all member functions of the class during the entire life span of the application, we must declare it as a data member of the class. By doing so, the array is available during the entire life span of the application.
- The functions atoi() and c_str() in string class might have to be used for converting C++ strings to integers. You must know which .h file should be included in order to use these functions.
phonenumbers.txt
9254567823 Ava Smith 9257734571 Donald Trump 9252222223 Hillary Clinton 9254683338 Bill Clinton 9251234478 David Jackson 9259889090 Mary Comey 9254327844 Michael Johnson 9253432234 Maria Peters 9259988898 Ester Zhang 9258728187 Noah Lee 9254567423 Olivia Smith 9251234571 Sophia Jackson 9259889791 Emma Comey 9254327848 Esther Johnson 9253435555 Lonnie Lynn
The skeleton code
//Main.cpp
/// How does the use of the Manager class avoid memory leak? /// Write your answer here. #include #include "PhoneMgr.h" #include /// For using atoi() #include #include #include using namespace std; int main() { string input; PhoneMgr pm;
/// TO DO: Get the record count of the phone system
do { cout << "Enter D for listing, R for random choice, Q for quit, or an index number." << endl; cin >> input; if (input == "D" || input == "d") pm.Display(); else if (input == "R" || input == "r") pm.Random(); else if (input == "Q" || input == "q") { } else { /// TO DO: Convert the string to an int and check if is in the range. /// If it is, call Dial() function of PhoneMgr class. /// You may have to pass the converted int to the function. } }while(input != "Q" && input != "q"); return 0; } |
PhoneMgr.h
#ifndef PHONEMGR_H #define PHONEMGR_H #include "Phone.h" const int SIZE = 999; class PhoneMgr { private: Phone * arrPhone[SIZE] = {nullptr}; // Manager owns the main data structure int recordCount = 0; public: PhoneMgr(); ~PhoneMgr(); int getRecordCount() const; void Display(); void Random(); void Dial(); }; #endif // PhoneMgr_H |
//PhoneMgr.cpp
#include "PhoneMgr.h" #include // for using cin and cout #include // for using atoi() #include // for processing input files using namespace std; PhoneMgr::PhoneMgr() { fstream inputFile; long long number; string firstName, lastName, temp; string fileName = "phonenumbers.txt"; inputFile.open(fileName); /// The array is already initialized with nullptr. /// TO DO: /// Populate the array by "newing" phone objects and save their pointers /// in the array, and get the total record count of the input file. /// Close input file to avoid memory leak.
} PhoneMgr::~PhoneMgr() { /// TO DO: /// Delete all pointers in the array to avoid memory leak. } void PhoneMgr::Display() { /// TO DO: /// Display all phone numbers, first names and last names in three columns. } void PhoneMgr::Random() { /// TO DO: /// Randomly display a phone number, its corresponding first name and last /// name in three columns } void PhoneMgr::Dial() { /// TO DO: /// Get a number i from the user and display the phone number at the ith /// position, its corresponding first name and last name in three columns /// To the user, the first position is 1, not 0. /// You may have to add a parameter to the function. } int PhoneMgr::getRecordCount() const { return recordCount; } |
//Phone.h
#ifndef PHONE_H #define PHONE_H #include #include #include "stdlib.h" using namespace std; class Phone { private: long long phoneNumber; string firstName; string lastName; public: Phone(); ~Phone(); void setFirstName(string); void setLastName(string); void setPhoneNumber(long long); string getFirstName() const; string getLastName() const; long long getPhoneNumber() const; }; #endif // PHONE_H |
//Phone.cpp
#include "Phone.h" #include using namespace std; Phone::Phone() { } Phone::~Phone() { } void Phone::setFirstName(string f) { firstName = f; } void Phone::setLastName(string l) { lastName = l; } string Phone::getFirstName() const { return firstName; } string Phone::getLastName() const { return lastName; } void Phone::setPhoneNumber(long long s) { phoneNumber = s; } long long Phone::getPhoneNumber() const { return phoneNumber; } |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
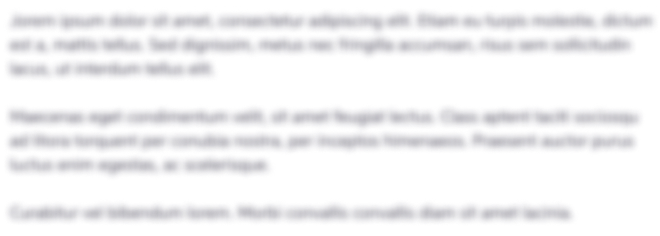
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started