Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In C++ I need the following taken care of... Criteria are as follows... Use menu Driven Program. Correctly Define the classes, their member variables and
In C++ I need the following taken care of...
Criteria are as follows...
Use menu Driven Program.
Correctly Define the classes, their member variables and accessors/mutators.
Correctly implemented required functions.
Program executes without error and the output is correctly and neatly formatted.
Below Is Sample Output...
Description: Write a menu-driven program that allows a user to show Uber Driver Requirements, Uber Vehicle Requirements, as well as take a test to see if the User Qualifies to be an Uber Driver. Requirements: 1. Use a menu-driven program that implements the following commands: a. Display Uber Vehicle Requirements b. Display Uber Driver Requirements c. See if you Qualify to be a driver d. Exit the program 2. Create the following Classes and their member variables. Also ensure that you create Accessors(getters) and Mutators (setters) a. Uber Vehicle.h i. int vehicleNumberOfDoors; ii. int vehicle Year; iii. string vehicleMake; iv. string vehicleModel; v. string in State VehiclePlates; vi. string is VehicleMarketTaxiOrSalvage; vii. string has Vehicle PassedInspection; viii. string isVehicleRegistered; b. UberDriver.h i. int ageOfdriver; ii. int yearsOfdriving Experiance; iii. string is VehicleEnsured ToDriver; iv. string is BackgroundCheckClear; v. string is DriverRecordClear; vi. string driver Has Smartphone; 3. Implement the following functions (at minimum, you can add as many as you see fit) a. showMenu(); // Show Menu i. Displays the menu ii. Inputs the user's selection iii. Validates the user's selection b. showUber Vehicle Requirements (); // Show Current Uber Vehicle Requirements i. This method creates an instance of the Uber Vehicle class and makes a call to an overloaded constructor to initialize the object with Uber's Vehicle Requirements Example: Uber Vehicle Uber Vehicle(4, 2001, "Toyota", "Camery", "Yes", "No", "Yes", "Yes"); Where each parameter in the constructor corresponds with the class member variables. c. showUberDriver Requirements 0; // Show Current Uber Driver Requirements i. Tthis method creates an instance of the Uber Driver class and makes a call to an overloaded constructor to initialize the object with Uber's Driver Requirements Example: UberDriver UberDriver(21, 3, "Yes", "Yes", "Yes", "Yes"); Where each parameter in the constructor corresponds with the class member variables. d.setUserData(); i. This method creates an instance of both the Uber Driver and Vehicle class, then asks a series of questions to set the object's mutators (setters) with the appropriate values. ii. Make sure to validate user's input. e. display Users Data(UberDriver, Uber Vehicle); i. This method should be called and passed two arguments UberDriver and UberVehicle. Both of these objects should hold user's data from the previous method. The sole purpose of this method is to display User's answers neatly formatted (see below screen shots) 4. Main program uses a while loop with embedded switch statement to call appropriate functions based on menu choice. Sample Output: **** MAIN MENU******* 1. Display Uber Vehicle Requirements 2. Display Uber Driver Requirements 3. See if you Qualify to be a driver 4. Exit the program Enter your choice (1-4): 1 Uber Vehicle Requirements | Vehicle Number of Doors: | Vehicle Year: | Vehicle Make: Vehicle Model: Does Vehicle have in state license plates: Is the vehicle market Taxi or Salvage: | Has vehicle passed Uber inspection: | Is the vehicle currentely registred: 2001 Toyota Camery Yes No Yes Yes * * * * MAIN MENU * * * * * * 1. Display Uber Vehicle Requirements 2. Display Uber Driver Requirements 3. See if you Qualify to be a driver 4. Exit the program Enter your choice (1-4): 2 Uber Driver Requirements Uber Driver Requirements | Minimum Age of Driver: | Years of Driving Experiance: | Is Vehicle Ensured to the Driver: Has background been cleared: | Has Driver Record been Cleared: | Does Driver have a Smart Phone: Yes Yes Yes Yes * * * * MAIN MENU * * * * * 1. Display Uber Vehicle Requirements 2. Display Uber Driver Requirements 3. See if you Quality to be a driver 4. Exit the program #2 3. See if you Qualify to be a driver 14. Exit the program Enter your choice (1-4): 3 Let's see if you Qualify to be a driver. Answer Each question to see if you Qualify to be an Uber Driver: Please Enter Your current Age: 24 Please Enter Your Years of driving Experiance: Is the vehicle Ensured to the Driver (Enter Yes/No): Yes Can the driver Pass a Background Check (Enter Yes/No): Yes Does the driver has a clear Driving Record (Enter Yes/No): NO Does the driver has a Smart Phone (Enter Yes/No): ves Please Enter Your Vehicles Make: Honda Please Enter Your Vehicles Model: Pilot Please Enter Your Vehicle's Year: 2014 Please Enter Your Vehicle's Number of Doors: Does Vehicle have in state license plates: (Enter Yes/No) Yes Is the vehicle marked Taxi or Salvage: (Enter Yes/No) NO Has the vehicle passed Uber inspection: (Enter Yes/No) Yes Is the vehicle currentely registred: (Enter Yes/No) Yes . Has the vehicle passed Uber inspection: (Enter Yes/No) Yes Is the vehicle currentely registred: (Enter Yes/No) Yes User's Application Data Questions | User's Answer | 24 Minimum Age of Driver: Years of Driving Experiance: Is Vehicle Ensured to the Driver: Has background been Cleared: Has Driver Record been cleared: Does Driver have a Smart Phone: Yes Yes NO Yes Vehicle Number of Doors: Vehicle Year: Vehicle Make: Vehicle Model: Does Vehicle have in state license plates: Is the vehicle market Taxi or Salvage: Has vehicle passed Uber inspection: Is the vehicle currentely registred: 2014 Honda Pilot Yes NO Yes Yes **** MAIN MENU 1. Display Uber Vehicle Requirements 2. Display Uber Driver Requirements 3. See if you Qualify to be a driver 4. Exit the program Enter your choice (1-4): Description: Write a menu-driven program that allows a user to show Uber Driver Requirements, Uber Vehicle Requirements, as well as take a test to see if the User Qualifies to be an Uber Driver. Requirements: 1. Use a menu-driven program that implements the following commands: a. Display Uber Vehicle Requirements b. Display Uber Driver Requirements c. See if you Qualify to be a driver d. Exit the program 2. Create the following Classes and their member variables. Also ensure that you create Accessors(getters) and Mutators (setters) a. Uber Vehicle.h i. int vehicleNumberOfDoors; ii. int vehicle Year; iii. string vehicleMake; iv. string vehicleModel; v. string in State VehiclePlates; vi. string is VehicleMarketTaxiOrSalvage; vii. string has Vehicle PassedInspection; viii. string isVehicleRegistered; b. UberDriver.h i. int ageOfdriver; ii. int yearsOfdriving Experiance; iii. string is VehicleEnsured ToDriver; iv. string is BackgroundCheckClear; v. string is DriverRecordClear; vi. string driver Has Smartphone; 3. Implement the following functions (at minimum, you can add as many as you see fit) a. showMenu(); // Show Menu i. Displays the menu ii. Inputs the user's selection iii. Validates the user's selection b. showUber Vehicle Requirements (); // Show Current Uber Vehicle Requirements i. This method creates an instance of the Uber Vehicle class and makes a call to an overloaded constructor to initialize the object with Uber's Vehicle Requirements Example: Uber Vehicle Uber Vehicle(4, 2001, "Toyota", "Camery", "Yes", "No", "Yes", "Yes"); Where each parameter in the constructor corresponds with the class member variables. c. showUberDriver Requirements 0; // Show Current Uber Driver Requirements i. Tthis method creates an instance of the Uber Driver class and makes a call to an overloaded constructor to initialize the object with Uber's Driver Requirements Example: UberDriver UberDriver(21, 3, "Yes", "Yes", "Yes", "Yes"); Where each parameter in the constructor corresponds with the class member variables. d.setUserData(); i. This method creates an instance of both the Uber Driver and Vehicle class, then asks a series of questions to set the object's mutators (setters) with the appropriate values. ii. Make sure to validate user's input. e. display Users Data(UberDriver, Uber Vehicle); i. This method should be called and passed two arguments UberDriver and UberVehicle. Both of these objects should hold user's data from the previous method. The sole purpose of this method is to display User's answers neatly formatted (see below screen shots) 4. Main program uses a while loop with embedded switch statement to call appropriate functions based on menu choice. Sample Output: **** MAIN MENU******* 1. Display Uber Vehicle Requirements 2. Display Uber Driver Requirements 3. See if you Qualify to be a driver 4. Exit the program Enter your choice (1-4): 1 Uber Vehicle Requirements | Vehicle Number of Doors: | Vehicle Year: | Vehicle Make: Vehicle Model: Does Vehicle have in state license plates: Is the vehicle market Taxi or Salvage: | Has vehicle passed Uber inspection: | Is the vehicle currentely registred: 2001 Toyota Camery Yes No Yes Yes * * * * MAIN MENU * * * * * * 1. Display Uber Vehicle Requirements 2. Display Uber Driver Requirements 3. See if you Qualify to be a driver 4. Exit the program Enter your choice (1-4): 2 Uber Driver Requirements Uber Driver Requirements | Minimum Age of Driver: | Years of Driving Experiance: | Is Vehicle Ensured to the Driver: Has background been cleared: | Has Driver Record been Cleared: | Does Driver have a Smart Phone: Yes Yes Yes Yes * * * * MAIN MENU * * * * * 1. Display Uber Vehicle Requirements 2. Display Uber Driver Requirements 3. See if you Quality to be a driver 4. Exit the program #2 3. See if you Qualify to be a driver 14. Exit the program Enter your choice (1-4): 3 Let's see if you Qualify to be a driver. Answer Each question to see if you Qualify to be an Uber Driver: Please Enter Your current Age: 24 Please Enter Your Years of driving Experiance: Is the vehicle Ensured to the Driver (Enter Yes/No): Yes Can the driver Pass a Background Check (Enter Yes/No): Yes Does the driver has a clear Driving Record (Enter Yes/No): NO Does the driver has a Smart Phone (Enter Yes/No): ves Please Enter Your Vehicles Make: Honda Please Enter Your Vehicles Model: Pilot Please Enter Your Vehicle's Year: 2014 Please Enter Your Vehicle's Number of Doors: Does Vehicle have in state license plates: (Enter Yes/No) Yes Is the vehicle marked Taxi or Salvage: (Enter Yes/No) NO Has the vehicle passed Uber inspection: (Enter Yes/No) Yes Is the vehicle currentely registred: (Enter Yes/No) Yes . Has the vehicle passed Uber inspection: (Enter Yes/No) Yes Is the vehicle currentely registred: (Enter Yes/No) Yes User's Application Data Questions | User's Answer | 24 Minimum Age of Driver: Years of Driving Experiance: Is Vehicle Ensured to the Driver: Has background been Cleared: Has Driver Record been cleared: Does Driver have a Smart Phone: Yes Yes NO Yes Vehicle Number of Doors: Vehicle Year: Vehicle Make: Vehicle Model: Does Vehicle have in state license plates: Is the vehicle market Taxi or Salvage: Has vehicle passed Uber inspection: Is the vehicle currentely registred: 2014 Honda Pilot Yes NO Yes Yes **** MAIN MENU 1. Display Uber Vehicle Requirements 2. Display Uber Driver Requirements 3. See if you Qualify to be a driver 4. Exit the program Enter your choice (1-4)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
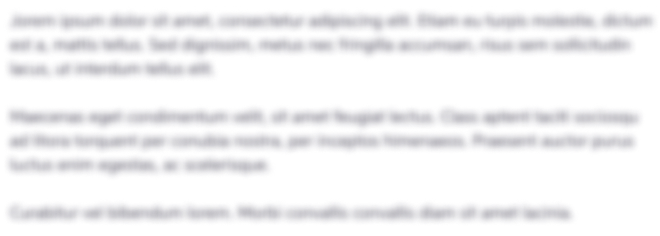
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started