Question
In C++, insert, delete and create a constructor and destructor for a BST with a linked list attached to each node.Tree nodes will contain a
In C++, insert, delete and create a constructor and destructor for a BST with a linked list attached to each node.Tree nodes will contain a letter of the alphabet and a linked list. The linked list will be an alphabetically sorted list of movies which start with that letter.
/****************************************************************/ /* MovieTree Definition */ /****************************************************************/ /* LEAVE THIS FILE AS IS! DO NOT MODIFY ANYTHING! =] */ /****************************************************************/
#ifndef MOVIETREE_HPP #define MOVIETREE_HPP
#include
/* Linked List node structure that will be stored at each node in the BST*/ struct LLMovieNode { int ranking; // Rank of the movie std::string title; // Title of the movie int year; // Release year float rating; // Movies rating struct LLMovieNode* next; // Pointer to the next node
LLMovieNode(){} // default constructor
// Parametrized constructor LLMovieNode(int r, std::string t, int y, float q) : ranking(r), title(t), year(y), rating(q), next(NULL) {} };
/* Node struct that will be stored in the MovieTree BST */
struct TreeNode { LLMovieNode* head = NULL; // Pointer to the head node of a LL char titleChar; // Starting character of the titles stored in the linked list TreeNode *parent = NULL; // Pointer to its parent node in BST TreeNode *leftChild = NULL; // Pointer to its leftChild in BST TreeNode *rightChild = NULL; // Pointer to its rightChild in BST };
/* Class for storing and manipulating the TreeNode's of BST*/
class MovieTree { public: // Check writeup for detailed function descriptions MovieTree(); ~MovieTree(); void printMovieInventory(); void addMovie(int ranking, std::string title, int year, float rating); void deleteMovie(std::string title);
private: TreeNode *root; };
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
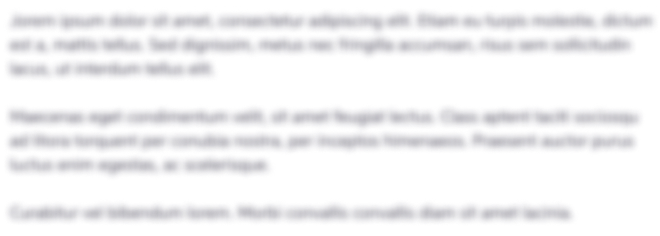
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started