Answered step by step
Verified Expert Solution
Question
1 Approved Answer
in c++ please. Final Grade in this course is a combination of Assignments average, Midterm and Final Exam scores. They are weighted differently. Let's write
in c++ please.
Final Grade in this course is a combination of Assignments average, Midterm and Final Exam scores. They are weighted differently. Let's write a program to calculate the Final Calculated Score and the Letter Grade for a student in this course. In this assignment, you will be wriling a program to calculate the final numerical score and the letter grade for a student in our class. You will read Assignment scores, and exam scores and calculate the final score and lefter grade based on the weights of Assignments' scores and Exams' scores. The purpose of this assignment is to modularize your program. Afler completing this assignment you will be able to: - Write functions to modularize your program and reduce redundancy - Write functions that take parameters by value and return values - Write void functions that take nothing and return nothing - Do dala validation for bad input dala for numbers - Use the correct exit condition to exit out of a loop Task Before you get started: Check out Sample Assignment A01 - Aloorithmic Design document Check out Sample Assignment A01 - SampleA01.cR Open the Algorithmic Design Document, make a copy, and follow the steps to create your algorithm. You must express your algorithm as pseudocode. You will write a program to read the number of assignments from the user. Then read the assignment scores, the midterm and final exam scores. Calculate the average assignment scores and then calculate the final numeric score using the assignments average, the midterm and final exam scores. The assignments are weighted at 60%, the midterm and final exams are weighted at 20% each. The final score will be calculated out of 4 and the letter grade will be based on the following table. Check the Sample Run and do the calculations on paper and make sure you get the right answers before you write the code. Wrile functions to do fhe following. Each function should do ONLY what it is supposed to do, and no more unless specified. Ater writing each function, call the function in main 0 to test it before writing the next function. 1. Welcome message (void function) 2. A function called int readint (string prompt) ; This function should be used any fime you read any integers from the user. Use this function to read the number of Assignments from the user. 3. A function called void readScore (atring prompt, donble Enum) ; to read all the scores from the user. Any time you read a score you must call this function. The function must do data validation to make sure it is a numeric value, and that it is within the range of 0 to 4 inclusive. 4. A function called double assignAverage (int numAssigns) ; to read the Assignment scores from the user. This function must call readScore every time you read an Assignment score. You must use a for loop to do this. Then calculate and return the average score. 5. A function called void getInput (double EmidtermScore, double SfinalExamScore) ; that calls the readScore () function and reads the 2 exam scores. 6. A function called double calcFina1score (double asaignAvg, double midterm, double final) ; that takes the Assignment average score, the midterm score and the final exam score, and calculates the final numerical score based on the appropriate weights for assignments and exams. The assignments are weighted at 60%, the midterm and final exams are weighted at 20% each. 7. A function called void caleLetterGrade (double finalscore, char 61etter); that takes the finaIScore by value and a character for the letter grade by reference. Print the letter grade in main (). 8. Your program must have function prototypes. Place the prototypes for your functions globally, after your \#fincludes. All functions must be implemented after main(). You may not use a while true loop and break statements in the loop. Your program must check for valid input data. For example, it should not accept negative numbers for scores and ail scores must be between 0 and 4 inclusive. Use loops to repeat until you get valid data from the user. Check out the sampleA.1. readint function that can be used to check for valid data. You can add the negative number check criteria to that function. Try not to have any redundart code (repeated code) in your program. That is the purpose of functions. All averages are displayed as with one digit precision. Use constants for all weights 0.6,0.2 elc for 60% and 20% Do not use arrays or any vectors for this program. Use only the concepts and functions we have learned so far. teria for Success Look at the sample run below. Determine the function prototypes you will need for the various options (your list may include other options): welcome () - void function, no parameters You must have these functions: readrnt (), readScore (), agsignaverage (), calcFinalscore (), calcLetterGrade (), and main () with the appropriate parameters and return types mentioned above under Task. Test your program using the following sample runs, making sure you get the same output when using the given inputs (in blue): Welcome to my Fina1 Grade Calculator! Pleage enter the following information and I will calculate pour Final Numerical Grade and Letter Grade for you! The number of agsignments nust be between 0 and 10 . A11 scores entered must be between 0 and 4. Fnter the number of assignments (o to 10 ) =6 Enter score 1: 3.4 Znter score 2: 4 Bnter score 3: 2.5 Bnter score 4=3.3 Enter score 5: 3.1 Fnter score 6:2.5 Enter your midtera exan score: 3.5 Enter your final exam gcore: 4 Your Einal Numerie score is 3.4 Your Final Grade is A Thank you for using my Grade Calculator! Welcome to my Final Grade Calculator: Please enter the following information and I will calculate your Final Numerical Grade and Letter Gzade for youl The number of assignments nust be between 0 and 10 . A11 scores entered must be between 0 and 4 . Enter the number of assignments (0 to 10):3 Enter score 1: 3 Bnter score 2: 4 Enter score 3: 2.5 Enter your midterm exam score: 2.5 Bntex your final exem scoxe: 2 Youx Final Numeric score is 2.8 Your Einal Grade in B Thank you for uging my Grade Calculatorl Welcome to my Final Grade Calculator1 please enter the following information and I wil1 calculate your Fina1 Numerical Grade and Letter Grade for you! The number of assignments must be between 0 and 10 . A11 scores entered must be between 0 and 4 . Bnter the number of assignments (0 to 10):12 Illegal Valuel please try again!l Bnter the number of agrignments (0 to 10):5 Bnter score 1: 3.4 Bnter score 2: 4 znter score 3:2.5 Bnter score 4=5.5 Illegal Score! please try again! Bnter score 4=3.5 Bnter score 5:3.1 Enter your midterm exam gcore: 3.5 Enter your final exam score: 4 Your Einal Numeric score is 3.5 Your Final Grade is A Thank you for using my Grade Calculatorll Youx Final Numeric score is 2.8 Your Einal Grade in B Thank you for uging my Grade Calculatorl Welcome to my Final Grade Calculator1 please enter the following information and I wil1 calculate your Fina1 Numerical Grade and Letter Grade for you! The number of assignments must be between 0 and 10 . A11 scores entered must be between 0 and 4 . Bnter the number of assignments (0 to 10):12 Illegal Valuel please try again!l Bnter the number of agrignments (0 to 10):5 Bnter score 1: 3.4 Bnter score 2: 4 znter score 3:2.5 Bnter score 4=5.5 Illegal Score! please try again! Bnter score 4=3.5 Bnter score 5:3.1 Enter your midterm exam gcore: 3.5 Enter your final exam score: 4 Your Einal Numeric score is 3.5 Your Final Grade is A Thank you for using my Grade Calculatorll







Step by Step Solution
There are 3 Steps involved in it
Step: 1
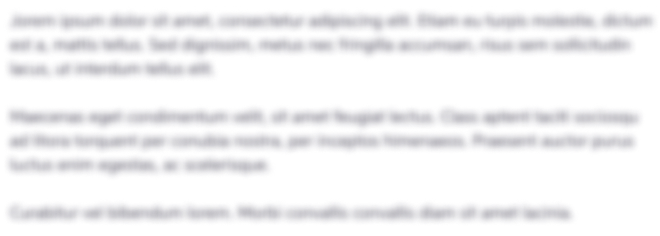
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started