In C++ please. It's a long question so if anyone can do their best. It would be greatly appreciated! Thank You! Create a simple movie
In C++ please. It's a long question so if anyone can do their best. It would be greatly appreciated! Thank You!
Create a simple movie database. You will populate your database by reading in information from a file whose name will be entered by the user. Each line in the file has the following format: Movie title, Gross Total (in billions), Director, Release Date, Runtime (in minutes) For example: Skyfall, 1.109, Sam Mendes, 11/9/12, 143 Each line in the file will need to be stored in an instance of a struct called Movie. The Movie struct will contain the following: a string representing the movie title, a double representing the gross total for the movie, a string for the directors name, a string for the release date, and an int for the movies runtime. This means that whatever you read in from the file will need to be parsed (and possibly converted). Every line in the file will be used to create a Movie object, which will be stored in a dynamicallyallocated array of Movie objects. For example, if my file has 10 movies, each line in the file will be used to populate a Movie object, which will then occupy a single index in my dynamicallyallocated array of 10 elements. This array is effectively acting as your database. Once your database has been populated appropriately, the user will be continually prompted to enter a movie title to search for. When the user enters a movie title, the program should search through the database for the movie title. If it is found, the movies information will be displayed; if it is not found, an appropriate error message will be displayed. The search should NOT be case sensitive. For example, if the database contains the movie Skyfall and the user inputs skYfaLL, the program should still be able to find the movie. After the movies information has been displayed (assuming the movie has been found), ask the user if they would like to save the movie. If they chose to do so, the movie should be added to a new, separate file called favorites.txt. Movies saved to the favorites file should have the same format as they do in the original file. For example, if the user chooses to save Skyfall, the favorites.txt file should have a line containing: Skyfall, 1.109, Sam Mendes, 11/9/12, 143 When the user chooses to exit the program, all saved movies from the favorites.txt file will be displayed
To recap, the basic flow of your program should be: 1. Populate your dynamically-allocated array of Movie objects by reading in information from the file 2. Ask the user to enter a movie title and perform a noncase-sensitive search a. If the movie is found, display the movies information and ask the user if they would like to save the movie; if they choose yes, save the movie to favorites.txt b. If the movie is not found, display an error message 3. Ask the user if they would like to exit a. If they choose not to exit, prompt them for a new movie title and repeat the process b. If they want to exit, display all movies in favorites.txt Program Requirements: Your program must have at least the following functions:
1. int numberOfLines(ifstream&); This function accepts an input file and returns an integer representing the number of lines in the file
2. void populateMovieFromFile(ifstream&, Movie&); This function accepts an input file and a Movie object. Inside the function, a single line should be read in from the current read position of the input file. Once the line has been read in, it will be parsed and stored in the Movie object that was passed to the function. Notice that for a single call of populateMovieFromFile, only a single Movie object is populated. This means you will need to call it multiple times!
3. void displayMovie(const Movie&); This function accepts a Movie object passed by constant reference and displays the contents of that object in a formatted fashion (see sample run).
4. Movie* createDatabase(int&); As the name suggests, this function will be responsible for creating your movie database (which is just a dynamically-allocated array of Movie objects). The integer argument passed by reference to the function represents the number of movies in the database. It will not be initialized when you call the function, meaning its value will be set inside the body of the function. This function should: First, prompt the user to enter an input file name. If the name is invalid, display an error and continue to prompt them until a valid file name has been entered Determine the number of lines in the file using the numberOfLines function and create your dynamically-allocated array of Movie objects Loop through your newly-created array and store a movie in each index. This is where you will want to call your handy populateMovieFromFile function Populate the reference argument and return a pointer to the array of Movie objects
5. bool caseInsensitiveCmp(string, string); This function accepts two strings and performs a noncase-sensitive comparison on the two. If the two strings are the same, the function should return true; otherwise, it should return false. For example, if I pass the strings skyfall and SKYFALL to this function, it should return true.
6. void findMovie(Movie*, int); This function accepts a pointer to an array of Movie objects (your database), and the number of Movies (which is the same thing as the number of elements in the array). The function should: First, prompt the user to enter a movie title to search for Search through the array of Movie objects for the movie title If the movie was found, display the movie and ask the user if they would like to save the movie; if they choose yes, the movie will be saved to favorites.txt If the movie was not found, display an error message Notice that this function only prompts the user for a single movie and only performs a single search. This means you will be using it in a loop!
7. void saveToFile(const Movie&); This function accepts a Movie object, passed by constant reference. It should save the Movie object to the favorites.txt file. If the file does not exist, create it. If the file does exist, add the Movie object to the end of the file. Remember that you need to store the Movie object in the format: Movie title, Gross Total (in billions), Director, Release Date, Runtime (in minutes)
8. bool promptToContinue(); This function will determine whether or not the user wants to continue using the program. First, ask the user if they would like to exit the program and prompt them to either enter Y or N. If Y or y is entered, the function will return false (the user no longer wishes to continue); otherwise, return true.
9. void displayFavorites(); This function will display all saved favorite movies by displaying all the information in the favorites.txt file. If there are no saved movies, display an error; otherwise, simply display all saved movies. Any user input, with the exception of the prompt to enter the file name, should be caseinsensitive. For example, if you prompt the user to enter Y or N, an input of y should be the same as Y.
Use the following data to create your file for testing Skyfall, 1.109, Sam Mendes, 11/9/12, 143 Avengers: Age of Ultron, 1.405, Joss Whedon, 5/1/15, 141 Harry Potter and the Deathly Hallows - Part 2, 1.342, David Yates, 7/15/11, 130 Furious 7, 1.516, James Wan, 4/1/15, 137 Rogue One, 1.056, Gareth Edwards, 12/16/16, 133 Minions, 1.159, Pierre Coffin & Kyle Blada, 7/10/15, 91 The Avengers, 1.519, Joss Whedon, 5/4/12, 143 Finding Dory, 1.029, Andrew Stanton, 6/17/16, 97 Iron Man 3, 1.215, Shane Black, 5/3/13, 130 Pirates of the Caribbean: Dead Man's Chest, 1.066, Gore Verbinski, 7/7/06, 150 Avatar, 2.788, James Cameron, 12/18/09, 161 Pirates of the Caribbean: On Stranger Tides, 1.045, Rob Marshall, 5/20/11, 137 Captain America: Civil War, 1.153, Anthony Russo & Joe Russo, 5/6/16, 147 Star Wars: The Force Awakens, 2.068, J. J. Abrams, 12/18/15, 136 Jurassic World, 1.672, Colin Trevorrow, 6/12/15, 124 Transformers: Dark of the Moon, 1.124, Michael Bay, 6/29/11, 154 Beauty and the Beast, 1.233, Bill Condon, 3/17/17, 129 The Fate of the Furious, 1.215, F. Gary Gary, 4/14/17, 136 The Dark Knight Rises, 1.085, Christopher Nolan, 7/20/12, 165 Toy Story 3, 1.067, Lee Unkrich, 7/18/10, 103 Transformers: Age of Extinction, 1.104, Michael Bay, 6/27/14, 165 Frozen, 1.276, Chris Buck & Jennifer Lee, 11/27/13, 102 Jurassic Park, 1.03, Steven Spielberg, 6/11/93, 127 The Lord of the Rings: The Return of the King, 1.12, Peter Jackson, 12/17/03, 200 Titanic, 2.187, James Cameron, 12/19/97, 195
Step by Step Solution
There are 3 Steps involved in it
Step: 1
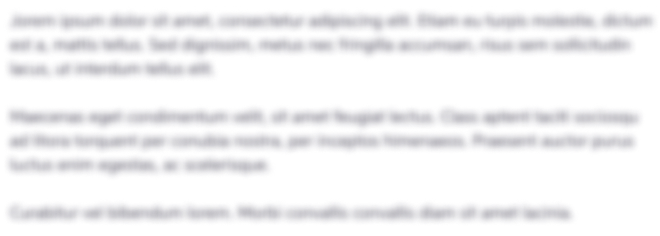
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started