Answered step by step
Verified Expert Solution
Question
00
1 Approved Answer
In C, please read carefully and have the output similar in the picture. This is my 3rd post and people are posting codes not related
In C, please read carefully and have the output similar in the picture.
This is my 3rd post and people are posting codes not related to this problem or having different output.
The key string and cipher text are in the picture
Step by Step Solution
There are 3 Steps involved in it
Step: 1
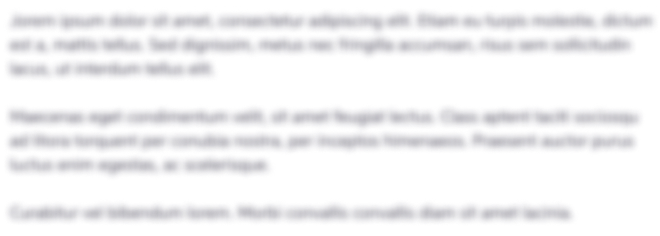
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started