Question
IN C Programming For this pre-lab you will create an abstract data type (ADT) which simulates operations associated with the ordinary concept of a list.
IN C Programming
For this pre-lab you will create an abstract data type (ADT) which simulates operations associated with the ordinary concept of a list. Those operations include: creating a list, adding items to the list, asking what the kth item is on the list, asking what item is at the end (tail) of the list, and throwing away (i.e., deleting or freeing) the list. For now well keep things simple and assume that we only need to store floats in our lists. Your ADT will be called List so youll need to use typedef with whatever struct you use/define to store the information youll need for these operations. An example of a struct definition that could work is:
struct listnode {
int maxSizeOfList, indexOfLastItemOfList;
float *array; }
Your typedef should allow a user to define a variable such as: List groceryList; Below are the interfaces / prototypes youll need to implement: 1. int createList(List *, int) This function creates and initializes a List variable. The first argument takes the address of a List variable so that its element values can be initialized by the function. The second argument is an integer giving the maximum possible size of the list. An array of that size will be allocated and the user is responsible for not adding more items to the list than this array can hold. Of course, because this is an ADT the user doesnt know anything about an array. S/he just knows that a maximum number of elements must be specified and that the number of items added to the list should not exceed that limit. The return value will be zero if the array cannot be allocated and nonzero otherwise. The user is responsible for checking the return value to determine whether an error occurred.
2. float addItem(float, List) This function takes the first argument and makes it the last item, i.e., the value at the end of the List specified by the second argument. (Why doesnt the second argument require a pointer?)
3. float getItem(int, List) This function returns the value located at the index given by the first argument.
4. int sizeOfList(List) This function returns the number of items on the list.
5. void deleteList(List *) This function deletes the memory allocated for the array. After this function is executed the user can call createList using the same List variable to define a new list. (Think about why this function is needed.)
Note that the addItem function can fail if the user attempts to add an item beyond the size limit set in createList. The function getItem also can encounter a logical error condition if the user attempts to access from an index beyond the number of items in the list or, even worse, beyond the size of the array. Because these two functions dont check for errors it is the users responsibility to ensure that such errors are not committed. It is therefore critical to include a warning about this in the documentation (comments) you associate with your functions.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
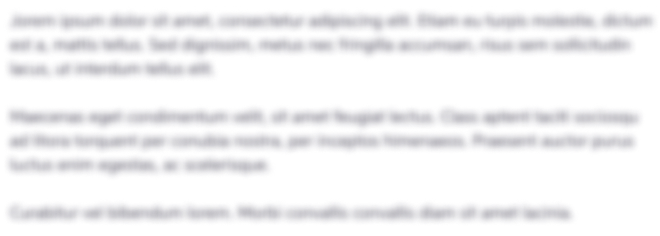
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started