Question
In C++, using only .h and main files.Use the ContactInfo class, Implement the operators in your code, and make the modifications to the code, to
In C++, using only .h and main files.Use the ContactInfo class, Implement the operators in your code, and make the modifications to the code, to create a one-dimensional array composed of instances of type ContactInfo and show its results in solving the following problems:
1. SearchableVector Modification Modify the SearchableVector class template presented in this chapter so that it per-
forms a binary search instead of a linear search. Test the template in a driver program.
2.SortableVector Class Template
Write a class template named SortableVector. The class should be derived from the SimpleVector class presented in this chapter. It should have a member function that sorts the array elements in ascending order. Sort with Selection Sort algorithm. Test the template in a driver program.
// ContactInfo class
#ifndef CONTACTINFO_H
#define CONTACTINFO_H
#include // Needed for strlen and strcpy
// ContactInfo class declaration.
class ContactInfo
{
private:
char *name; // The contact's name
char *phone; // The contact's phone number
// Private member function: initName
// This function initializes the name attribute.
void initName(char *n)
{ name = new char[strlen(n) + 1];
strcpy(name, n); }
// Private member function: initPhone
// This function initializes the phone attribute.
void initPhone(char *p)
{ phone = new char[strlen(p) + 1];
strcpy(phone, p); }
public:
// Constructor
ContactInfo(char *n, char *p)
{ // Initialize the name attribute.
initName(n);
// Initialize the phone attribute.
initPhone(n); }
// Destructor
~ContactInfo()
{ delete [] name;
delete [] phone; }
const char *getName() const { return name; }
const
char *getPhoneNumber() const { return phone; }
} #endif
//searchablevector
#include
#include "SimpleVector.hpp"
#include "SimpleVectorInterface.hpp"
template <class T>
class SearchableVector : public SimpleVector
{
public:
// Default constructor
SearchableVector();
// Constructor
SearchableVector(int size);
// Copy constructor
SearchableVector(const SearchableVector &);
// Accessor to find an item
int findItem(const T);
};
//*******************************************************
// Copy constructor *
//*******************************************************
// Default constructor
template <class T>
SearchableVector::SearchableVector() : SimpleVector(){ }
// Constructor
template <class T>
SearchableVector::SearchableVector(int size) : SimpleVector(size){ }
template <class T>
SearchableVector::SearchableVector(const SearchableVector &obj)
:SimpleVector(obj.getArraysize())
{
for (int count = 0; count < this->getArraysize(); count++)
this->operator[](count) = obj[count];
}
//********************************************************
// findItem function *
// This function searches for item. If item is found *
// the subscript is returned. Otherwise 1 is returned. *
//********************************************************
template <class T>
int SearchableVector::findItem(const T item)
{
for (int count = 0; count <= this->getItemCount(); count++)
{
if (SimpleVector::getElementAt(count) == item)
return count;
}
return -1;
};#endif /* SearchableVector_hpp */
//simplevector
#include
#include
#include // Needed for bad_alloc exception
#include // Needed for the exit function
using namespace std;
#include "SimpleVectorInterface.hpp"
template <class T>
class SimpleVector:public SimpleVectorInterface
{
//private:
protected:
T *aptr; // To point to the allocated array
void memError(); // Handles memory allocation errors
void subError(); // Handles subscripts out of range
int arraySize; //Number of elements of array
int itemCount;
public:
// Default constructor
SimpleVector();
// Constructor declaration
SimpleVector(int);
// Copy constructor declaration
SimpleVector(const SimpleVector &);
// Destructor declaration
virtual ~SimpleVector();
// Accessor to return the array size
int getArraySize() const;
// Accessor to return a specific element
int getItemCount() const;
T getElementAt(int position);
// Overloaded [] operator declaration
T &operator[](const int &);
virtual bool add(const T &newEntry);
virtual bool remove(const T &anEntry);
virtual int getIndexOf(const T &targetEntry) const;
virtual bool isEmpty() const;
virtual void display()const;
};
template <class T>
SimpleVector::SimpleVector()
{
aptr = 0;
arraySize = 0;
itemCount = 0;
}
//************************************************************
// Constructor for SimpleVector class. Sets the size of the *
// array and allocates memory for it. *
//************************************************************
template <class T>
SimpleVector::SimpleVector(int s)
{
arraySize = s;
itemCount = 0;
// Allocate memory for the array.
try
{
aptr = new T[s];
}
catch (bad_alloc)
{
memError();
}
}
//*******************************************
// Copy Constructor for SimpleVector class. *
//*******************************************
template <class T>
SimpleVector::SimpleVector(const SimpleVector &obj)
{
// Copy the array size.
arraySize = obj.arraySize;
itemCount = obj.itemCount;
// Allocate memory for the array.
aptr = new T[arraySize];
if (aptr == 0)
memError();
// Copy the elements of obj's array.
for (int count = 0; count < getItemCount(); count++)
* (aptr + count) = *(obj.aptr + count);
}
//**************************************
// Destructor for SimpleVector class. *
//**************************************
template <class T>
SimpleVector::~SimpleVector()
{
if (itemCount > 0)
delete[] aptr;
}
//********************************************************
// memError function. Displays an error message and *
// terminates the program when memory allocation fails. *
//********************************************************
template <class T>
void SimpleVector::memError()
{
cout << "ERROR:Cannot allocate memory. ";
exit(EXIT_FAILURE);
}
//************************************************************
// subError function. Displays an error message and *
// terminates the program when a subscript is out of range. *
//************************************************************
template <class T>
void SimpleVector::subError()
{
cout << "ERROR: Subscript out of range. ";
exit(EXIT_FAILURE);
}
//*******************************************************
// getElementAt function. The argument is a subscript. *
// This function returns the value stored at the *
// subcript in the array. *
//*******************************************************
template <class T>
T SimpleVector::getElementAt(int sub)
{
if (sub < 0 || sub > getItemCount())
subError();
return aptr[sub];
}
//********************************************************
// Overloaded [] operator. The argument is a subscript. *
// This function returns a reference to the element *
// in the array indexed by the subscript. *
//********************************************************
template <class T>
T &SimpleVector::operator[](const int &sub)
{
if (sub < 0 || sub > getItemCount())
subError();
return aptr[sub];
}
template <class T>
int SimpleVector::getArraySize() const{
return arraySize;
}
template <class T>
int SimpleVector::getItemCount() const{
return itemCount;
}
template <class T>
bool SimpleVector::add(const T &newEntry){
bool hasRoomToAdd = (itemCount < getArraySize());
if (hasRoomToAdd){
aptr[itemCount] = newEntry;
itemCount++;
}//end if
return hasRoomToAdd;
}
template <class T>
int SimpleVector::getIndexOf(const T &targetEntry) const{
bool found = false;
int result = -1;
int searchIndex = 0;
while (!found && (searchIndex < getItemCount())){
if (aptr[searchIndex] == targetEntry){
found = true;
result = searchIndex;
} //end if
else{
searchIndex++;
}//end else
}//end whule
return result;
}
template <class T>
bool SimpleVector::isEmpty() const{
bool result;
if (getItemCount() == 0)
result=true;
else result = false;
return result;
}
template <class T>
bool SimpleVector::remove(const T &anEntry){
int locatedIndex = getIndexOf(anEntry);
bool canRemoveItem = !isEmpty() && (locatedIndex > -1);
if (canRemoveItem){
itemCount--;
aptr[locatedIndex] = aptr[itemCount];
}//end if
return canRemoveItem;
}
template <class T>
void SimpleVector::display() const{
cout << "El contenido dentro del arreglo es:";
for (int index = 0; index < getItemCount(); index++){
cout << aptr[index] << endl;
}//end for
}#endif /* SimpleVector_hpp */
//simplevectorinterface
#ifndef SimpleVectorInterface_hpp
#define SimpleVectorInterface_hpp
#include
template<class T>
class SimpleVectorInterface
{
public:
~SimpleVectorInterface() {}
virtual bool add(const T &newEntry)=0;
virtual bool remove(const T &anEntry)=0;
virtual int getIndexOf(const T &targetEntry) const=0;
virtual bool isEmpty() const =0 ;
virtual void display()const=0;
};#endif /* SimpleVectorInterface_hpp */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
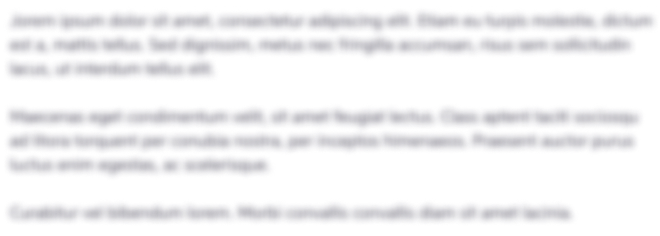
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started