In class we discussed the timeslice CPU scheduling algorithm. We also considered other ways that the processes in the CPU could be scheduled. Modify the time-slicing CPU scheduling algorithm
Use the programming language given (use python)
# Given: A list of processes with execution times # Find: A schedule of the processes using time slices import queue import random # Function to open the file using exception handling def openFile(): goodFile = False while goodFile == False: fname = input("Enter name of data file: ") try: inFile = open(fname, 'r') goodFile = True except IOError: print("Invalid filename, please try again ... ") return inFile # Function to get the time slice value and the processes from the file into the queue # Queue will contain a string with process ID and exec time separated by a comma def getProcs(cpuQ): infile = openFile() # Get the first line in the file containing the time slice value line = infile.readline() # Strip the from the line and convert to an integer tslice = int(line.strip()) # Loop through the file inserting processes into the queue for line in infile: proc = line.strip() cpuQ.put(proc) infile.close() return tslice, cpuQ # Function to print the contents of the queue def printQueue(tslice, cpuQ): print("The time slice is ",tslice, " The contents of the queue are: ") for i in range(cpuQ.qsize()): proc = cpuQ.get() cpuQ.put(proc) print(proc) # Function to execute the processes in the queue def scheduleProcs(tslice, cpuQ): # While the queue is not empty while (cpuQ.empty() != True): # Get next process from queue proc = cpuQ.get() # Separate the process ID and the execution time from the process info PID, exectime = proc.split(",") # Convert exectime to an integer exectime = int(exectime) print("Getting next process - Process ", PID," has ", exectime," instructions to execute") # Initialize the timer timer = 0 # While proc still has time in slice and still has code to execute while (timer 0): # In a real computer, the OS would take an instruction from process out of memory and execute it # Execute an instruction of process exectime = exectime - 1 # Count one tick of the timer timer = timer + 1 print("Executing instruction ", exectime," of process ", PID,". Timer = ", timer) # If proc still has instructions to execute put it back in the queue if (exectime > 0): # Create string with new exec time and process ID proc = PID + "," + str(exectime) # Put the process back in the queue cpuQ.put(proc) print("Put process ", PID," back in queue with ", exectime," instructions left to execute") else: print("*** Process ", PID, " Complete ***") return # Main function def main(): # Create the scheduling queue cpuQ = queue.Queue() # Get the processes from the data file tslice, cpuQ = getProcs(cpuQ) # Print the queue printQueue(tslice, cpuQ) # Schedule the processes scheduleProcs(tslice, cpuQ)
to implement priority-based scheduling.
Assume that there are two priority levels in the operating system, HIGH and LOW. In the data following data file:
3 P1,11,H P2,5,L P3,3,M P4,7,H P5,8,L P6,9,M P7,5,H P8,2,M P9,4,L
each process has associated with it a priority specified by 'H' for high, 'M' for medium and 'L' for low. Write the program so that high priority processes get three times the time slice, medium priority processes get two times the time slice, and low priority processes get only one time slice.
Note: The input file has three items on each line instead of two. You will have to split the input accordingly. You also have to make sure put the process back into the queue in the correct format.
Your output will look something like this:
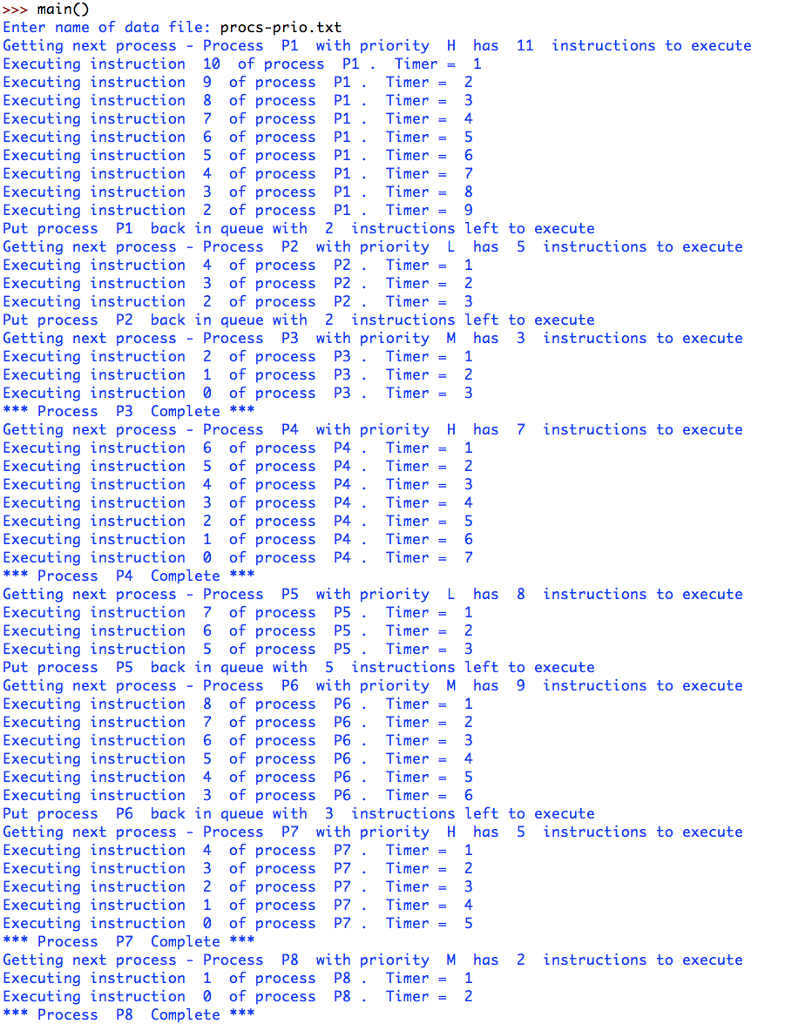
>>>mainO Enter name of data file:procs-prio.txt Getting next process-Process P1 with priority H has 11 instructions to execute Executing instruction 10 of process P1 Timer1 Executing instruction 9 of process P1 . Timer= 2 Executing instruction 8 of process P1 . Timer3 Executing instruction 7 of process P1 Timer4 Executing instruction 6 of process P1 . Timer5 Executing instruction 5 of process P1 . Timer 6 Executing instruction 4 of process P1 . Timer7 Executing instruction 3 of process P1 . Timer8 Executing instruction 2 of process P1Timer9 Put process P1back in queue with 2 instructions left to execute Getting next process-ProcessP2 with priority L has 5 instructions to execute Executing instruction 4 of process P2 . Timer= 1 Executing instruction 3 of process P2. Timer 2 Executing instruction 2 of process P2 . Timer- 3 Put process P2 back in queue with 2 instructions left to execute Getting next process Process P3 with priority M has 3 instructions to execute Executing instruction 2 of process P3 . Timer- 1 Executing instruction 1 of process P3 Timer2 Executing instruction 0 of process P3 Timer3 Process P3 Complete*** Getting next process-Process P4 with priority H has 7 instructions to execute Executing instruction 6 of process P4 . Timer 1 Executing instruction 5 of process P4 . Timer= 2 Executing instruction 4 of process P4 . Timer- 3 Executing instruction 3 of process P4.Timer4 Executing instruction 2 of process P4 . Timer5 Executing instruction 1 of process P4 . Timer 6 Executing instruction0 of process P4 . Timer7 Process Getting next process Process P5 with priority L has 8 instructions to execute Executing instruction 7 of process P5 . Timer- 1 Executing instruction 6 of process P5 Timer2 Executing instruction 5 of process P5Timer3 Put process P5 back in queue with 5 instructions left to execute Getting next process-Process P6 with priority M has9 instructions to execute Executing instruction 8 of process P6 . Timer 1 Executing instruction 7 of process P6 . Timer= 2 Executing instruction 6 of process P6 . Timer- 3 Executing instruction 5 of process P6 Timer4 Executing instruction 4 of process P6 Timer5 Executing instruction 3 of process P6.Timer 6 Put process P6 back in queue with 3 instructions left to execute Getting next process -Process P7 with priority H has 5 instructions to execute Executing instruction 4 of process P7Timer 1 Executing instruction 3 of process P7. Timer 2 Executing instruction 2 of process P7Timer3 Executing instruction 1 of process P7 . Timer= 4 Executing instruction of process P7.Timer 5 ***Process P7Complete*** Getting next process - Process P8 with priority M has 2 instructions to execute Executing instruction 1 of process P8. Timer 1 Executing instruction0 of process P8 Timer 2 Process P8 Complete** >>>mainO Enter name of data file:procs-prio.txt Getting next process-Process P1 with priority H has 11 instructions to execute Executing instruction 10 of process P1 Timer1 Executing instruction 9 of process P1 . Timer= 2 Executing instruction 8 of process P1 . Timer3 Executing instruction 7 of process P1 Timer4 Executing instruction 6 of process P1 . Timer5 Executing instruction 5 of process P1 . Timer 6 Executing instruction 4 of process P1 . Timer7 Executing instruction 3 of process P1 . Timer8 Executing instruction 2 of process P1Timer9 Put process P1back in queue with 2 instructions left to execute Getting next process-ProcessP2 with priority L has 5 instructions to execute Executing instruction 4 of process P2 . Timer= 1 Executing instruction 3 of process P2. Timer 2 Executing instruction 2 of process P2 . Timer- 3 Put process P2 back in queue with 2 instructions left to execute Getting next process Process P3 with priority M has 3 instructions to execute Executing instruction 2 of process P3 . Timer- 1 Executing instruction 1 of process P3 Timer2 Executing instruction 0 of process P3 Timer3 Process P3 Complete*** Getting next process-Process P4 with priority H has 7 instructions to execute Executing instruction 6 of process P4 . Timer 1 Executing instruction 5 of process P4 . Timer= 2 Executing instruction 4 of process P4 . Timer- 3 Executing instruction 3 of process P4.Timer4 Executing instruction 2 of process P4 . Timer5 Executing instruction 1 of process P4 . Timer 6 Executing instruction0 of process P4 . Timer7 Process Getting next process Process P5 with priority L has 8 instructions to execute Executing instruction 7 of process P5 . Timer- 1 Executing instruction 6 of process P5 Timer2 Executing instruction 5 of process P5Timer3 Put process P5 back in queue with 5 instructions left to execute Getting next process-Process P6 with priority M has9 instructions to execute Executing instruction 8 of process P6 . Timer 1 Executing instruction 7 of process P6 . Timer= 2 Executing instruction 6 of process P6 . Timer- 3 Executing instruction 5 of process P6 Timer4 Executing instruction 4 of process P6 Timer5 Executing instruction 3 of process P6.Timer 6 Put process P6 back in queue with 3 instructions left to execute Getting next process -Process P7 with priority H has 5 instructions to execute Executing instruction 4 of process P7Timer 1 Executing instruction 3 of process P7. Timer 2 Executing instruction 2 of process P7Timer3 Executing instruction 1 of process P7 . Timer= 4 Executing instruction of process P7.Timer 5 ***Process P7Complete*** Getting next process - Process P8 with priority M has 2 instructions to execute Executing instruction 1 of process P8. Timer 1 Executing instruction0 of process P8 Timer 2 Process P8 Complete**