Question
In Eclipse, create a Lab4 project and add class files called: TestBikes.java, Bicycle.java, MountainBike.java and RoadBike.java There is something wrong with the class Bicycle, fix
-
In Eclipse, create a Lab4 project and add class files called: TestBikes.java, Bicycle.java, MountainBike.java and RoadBike.java
-
There is something wrong with the class Bicycle, fix it.
-
Override the method applyBrake in MountainBike to change the new speed to be: speed = speed-decrement*2.
-
Write a new class called RoadBike that extends Bicycle. Because road bikes have skinny tires, add an attribute to track the tire width, and write getTireWidth and setTireWidth to get and set the values of tire width. The constructor of RoadBike also should have an argument to pass the tire width. Note: You dont need to write this class from scratch. RoadBike is almost identical to MountainBike, but deals with tireWidth rather than suspension
-
Uncomment the two lines in Main() which create and add RoadBikes to the ArrayList
-
Complete printList in TestBikes to iterate over the ArrayList, and check the type of the bike that is passed using instanceof. If the bike is of type MountainBike, it should print: This is a MountainBike and then it should call printDescription. If the bike is of type RoadBike, it should print: This is a RoadBike and then it should call printDescription.
Helpful hint: You can get the size of an ArrayList using size(), and retrieve an item in an ArrayList using get(i) where i is the position in the list
-
Run TestBikes.java to make sure your program works
/*################################################### This code should go in a TestBikes.java File ####################################################*/ import java.util.ArrayList; public class TestBikes { public static void main(String[] args){ ArrayList bikes = new ArrayList(); bikes.add(new MountainBike(2,10,1,"heavy")); bikes.add(new MountainBike(5,25,3,"light")); //bikes.add(new RoadBike(2,10,1,1)); //bikes.add(new RoadBike(5,25,3,2)); printList(bikes); } private static void printList(ArrayList bikes) { } } /*################################################### This code should go in a Bicycle.java File ####################################################*/ public class Bicycle { // the Bicycle class has three fields public int cadence; public int gear; public int speed; // the Bicycle class has one constructor public Bicycle(int startCadence, int startSpeed, int startGear) { gear = startGear; cadence = startCadence; speed = startSpeed; } // the Bicycle class has four methods public void setCadence(int newValue) { cadence = newValue; } public void setGear(int newValue) { gear = newValue; } public void applyBrake(int decrement) { speed -= decrement; } public void speedUp(int increment) { speed += increment; } public abstract void printDescription(); } /*################################################### This code should go in a MountainBike.java File ####################################################*/ public class MountainBike extends Bicycle { private String suspension; public MountainBike( int startCadence, int startSpeed, int startGear, String suspensionType){ super(startCadence, startSpeed, startGear); this.setSuspension(suspensionType); } public String getSuspension(){ return this.suspension; } public void setSuspension(String suspensionType) { this.suspension = suspensionType; } public void printDescription() { System.out.println("The " + "MountainBike has a " + getSuspension() + " suspension."); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
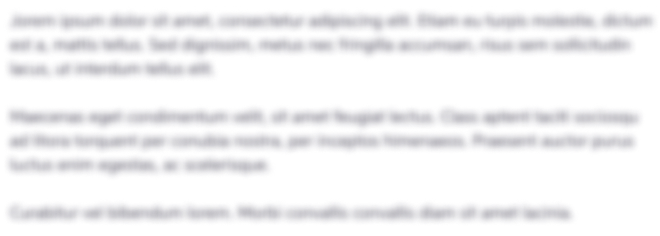
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started