Question
In Java 1. Updated CustomLinkedList class with the definitions of the methods: findAll, update, updateAll, updateAtPos. 2. Updated Driver class with the new options. 3.
In Java
1. Updated CustomLinkedList class with the definitions of the methods: findAll, update, updateAll, updateAtPos.
2. Updated Driver class with the new options.
3. Implement a Queue using int array of size 10. Your class must contain the methods as enQueue, deQueue, peek, isEmpty, getSize, display. Create a driver class to demonstrate the working of the Queue class.
4. Implement a Stack using two different ways, (two different classes)
a. using an array of size 10,
b. using the CustomLinkedList class.
Your each class must contain the methods like push, pop, getSize, display. Also, create a driver class each to demonstrate the working of the two Stack classes.
--------------------------------------------------------------------------------
Here is the code provided:
package linkedlist_queue;
/* * This class is a driver for the CustomQueue * * @author Dr. Chetan Jaiswal * August 29 2018 */
import java.util.Scanner;
public class CustomQueueDriver {
public static void main(String[] args) { Scanner scan = new Scanner(System.in); /* Creating object of class linkedList */ CustomQueue q = new CustomQueue(); System.out.println("My Queue"); /* Perform list operations */ while(true) { System.out.println(" Queue Options "); System.out.println("1. Enqueue"); System.out.println("2. Dequeue"); System.out.println("3. Check Empty?"); System.out.println("4. Display"); System.out.println("5. Peek"); System.out.println("6. Get Size"); System.out.println("7. exit"); int choice = scan.nextInt(); switch (choice) { case 1 : //Enqueue System.out.println("Enter integer element to insert"); q.enqueue( scan.nextInt() ); break; case 2 : //Dequeue q.dequeue(); break; case 3 : //Check empty System.out.println(q.isEmpty()?"Empty":"Not Empty"); break; case 4 : //display q.display(); break; case 5 : //peek System.out.println(q.peek()); break; case 6 : //get size System.out.println("Size = "+ q.getSize()); break; case 7 : //terminate scan.close(); System.exit(0); default : System.out.println("Wrong Entry "); break; } } } }
---------------------------
package linkedlist_queue;
/* * This class implements the logic of a queue using linked list * * @author Dr. Chetan Jaiswal * August 29 2018 */ public class CustomQueue { private CustomLinkedList q; private Node front, rear; public CustomQueue() { q = new CustomLinkedList(); front = q.getStart(); rear = q.getEnd(); } public void updatePointers() { front = q.getStart(); if(front==null) { rear = front; return; } rear = q.getEnd(); } public void enqueue(int data) { q.insertAtEnd(data); updatePointers(); System.out.print("Operation Enqueue-"); display(); if(front != null) { System.out.println("Front is:" + front.getData()); System.out.println("Rear is:" + rear.getData()); } } public void dequeue() { if(front == null) { System.out.println("Queue is empty"); return; } q.deleteAtPos(1); updatePointers(); System.out.print("Operation Dequeue-"); display(); if(front != null) { System.out.println("Front is:" + front.getData()); System.out.println("Rear is:" + rear.getData()); } } public int peek() //return -1 in case Queue is empty { if(front == null) { System.out.println("Queue is empty"); return -1; } return front.getData(); } public boolean isEmpty() { return front==null?true:false; } public void display() { System.out.print(" Queue = "); if (q.getSize() == 0) { System.out.print("empty "); return; } if (front.getNext() == null) { System.out.println(front.getData() ); return; } Node aNode = front; System.out.print(front.getData()+ " <- "); aNode = front.getNext(); while (aNode.getNext() != null) { System.out.print(aNode.getData()+ " <- "); aNode = aNode.getNext(); } System.out.print(aNode.getData()+ " "); } public int getSize()//-1 indicates that the queue is empty { if(front == null) { System.out.println("Queue is empty"); return -1; } return q.getSize(); } }
-----------------------------------
package linkedlist_queue;
import java.util.Scanner; /* * This class is a driver for the Singly or One way linked list * * @author Dr. Chetan Jaiswal * August 29 2018 */
public class LinkedListDriver { public static void main(String[] args) { Scanner scan = new Scanner(System.in); /* Creating object of class linkedList */ CustomLinkedList list = new CustomLinkedList(); System.out.println("My Linked List"); /* Perform list operations */ while(true) { System.out.println(" Singly Linked List Options "); System.out.println("1. insert at begining"); System.out.println("2. insert at end"); System.out.println("3. insert at position"); System.out.println("4. delete at position"); System.out.println("5. check empty"); System.out.println("6. get size"); System.out.println("7. exit"); int choice = scan.nextInt(); switch (choice) { case 1 : //Insert at beginning System.out.println("Enter integer element to insert"); list.insertAtStart( scan.nextInt() ); break; case 2 : //Insert at end System.out.println("Enter integer element to insert"); list.insertAtEnd( scan.nextInt() ); break; case 3 : //Insert at position System.out.println("Enter integer element to insert"); int num = scan.nextInt() ; System.out.println("Enter position"); int pos = scan.nextInt() ; if (pos <= 1 || pos > list.getSize() ) System.out.println("Invalid position "); else list.insertAtPos(num, pos); break; case 4 : //delete at position System.out.println("Enter position"); int p = scan.nextInt() ; if (p < 1 || p > list.getSize() ) System.out.println("Invalid position "); else list.deleteAtPos(p); break; case 5 : //check empty System.out.println("Empty status = "+ list.isEmpty()); break; case 6 : //get size System.out.println("Size = "+ list.getSize() +" "); break; case 7 : //terminate scan.close(); System.exit(0); default : System.out.println("Wrong Entry "); break; } /* Display List */ LinkedListDriver.display(list); } } /* Function to display elements */ public static void display(CustomLinkedList list) { Node start = list.getStart(); System.out.print(" Linked List = "); if (list.getSize() == 0) { System.out.print("empty "); return; } if (start.getNext() == null) { System.out.println(start.getData() ); return; } Node aNode = start; System.out.print(start.getData()+ "->"); aNode = start.getNext(); while (aNode.getNext() != null) { System.out.print(aNode.getData()+ "->"); aNode = aNode.getNext(); } System.out.print(aNode.getData()+ " "); } }
-------------------------------------
package linkedlist_queue;
/* * This class implements a Singly or One way linked list * The standard options for linked list operations are also implemented (like insert a node or deleting a node) * @author Dr. Chetan Jaiswal * August 23 2018 */ public class CustomLinkedList { private Node start; private Node end ; private int size ; /* Constructor */ public CustomLinkedList() { start = null; end = null; size = 0; } /* Function to check if list is empty */ /** * * @return true: if the list is empty, false: otherwise */ public boolean isEmpty() { if(start == null) return true; else return false; } /* Function to get size of list */ /** * * @return size of the list (# of nodes) */ public int getSize() { return size; } /* Function to insert an element at beginning */ /** * * @param val value to be inserted at the first node of the list */ public void insertAtStart(int val) { Node node = new Node(val, null); size++ ; if(start == null) { start = node; end = start; } else { node.setNext(start); start = node; } } /* Function to insert an element at end */ /** * * @param val value to be inserted at the last node of the list */ public void insertAtEnd(int val) { Node node = new Node(val,null); size++ ; if(start == null) { start = node; end = start; } else { end.setNext(node); end = node; } } /* Function to insert an element at position */ /** * * @param val value to be inserted in the list * @param pos position of the value in the list */ public void insertAtPos(int val , int pos) { //write code here to make it safe method call //should print error msg if user tries to insert at the start //or at the end. Should return after printing the msg Node node = new Node(val, null); Node aNode = start; pos = pos - 1 ; for (int i = 1; i < size; i++) { if (i == pos) { Node tmp = aNode.getNext() ; aNode.setNext(node); node.setNext(tmp); break; } aNode = aNode.getNext(); } size++ ; } /* Function to delete an element at position */ /** * * @param pos position from which the item needs to be deleted (node position) */ public void deleteAtPos(int pos) { if (pos == 1) { start = start.getNext(); size--; return ; } if (pos == size) { Node s = start; Node t = start; while (s != end) { t = s; s = s.getNext(); } end = t; end.setNext(null); size --; return; } Node aNode = start; pos = pos - 1 ; for (int i = 1; i < size - 1; i++) { if (i == pos) { Node tmp = aNode.getNext(); tmp = tmp.getNext(); aNode.setNext(tmp); break; } aNode = aNode.getNext(); } size-- ; } /** * * @param val accepts an int argument for the value to be searched * @return the position of the data (node), -1 if not found */ public int findData(int val) { Node aNode = start; int position=1; while(!(aNode == null)) { if(aNode.getData()==val) { System.out.println("Found in LinkedList"); return position; } aNode = aNode.getNext(); position++; } return -1; } /** * * @param val accepts an int argument for the value to be searched * @return an int array with all the positions of the val in the linkedlist, -1 if not found */ public int[] findAll(int val) { int [] positions = null; //TO DO return positions; } /** * updates the first occurrence of initialVal to newVal * @param initialVal old val to be updated * @param newVal new value with which old val is replaced */ public void update(int initialVal, int newVal) { //TO DO } /** * updates all the occurrences of initialVal to newVal * @param initialVal old val to be updated * @param newVal new value with which old val is replaced */ public void updateAll(int initialVal, int newVal) { //TO DO } /** * updates the value of a node at the positio pos * @param pos position of the node * @param newVal new value that needs to be updated at the pos */ public void updateAtPos(int pos, int newVal) { // TO DO } /** * * @return the start reference of the linkedlist */ public Node getStart() { return start; } /** * * @return the end reference of the linkedlist */ public Node getEnd() { return end; } }
-------------------------------------------------------
package linkedlist_queue;
/* * This class implements the NODE of a Singly or One way linked list * * @author Dr. Chetan Jaiswal * August 29 2018 */ public class Node { private int data; private Node next; /* Constructor */ public Node() { next = null; data = 0; } /* Constructor */ public Node(int d,Node n) { data = d; next = n; } /* Function to set link to next Node */ public void setNext(Node n) { next = n; } /* Function to set data to current Node */ public void setData(int d) { data = d; } /* Function to get link to next node */ public Node getNext() { return next; } /* Function to get data from current Node */ public int getData() { return data; } }
-------------------------------------------
Thank you, I apologize for the rather vague instructions, my prof is not very good at explaining thing hence why I came here for help.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
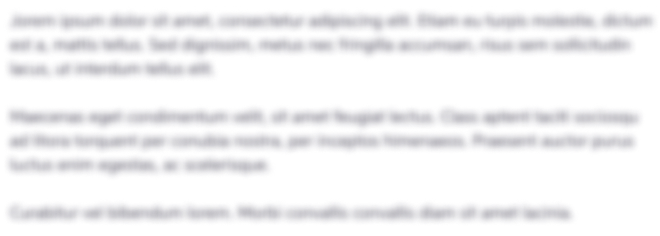
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started