Question
IN JAVA CODE - please use original code 3. Modify Restart.action() to start the the system by reading events from a text file. Use Scanner
IN JAVA CODE - please use original code
3. Modify Restart.action() to start the the system by reading events from a text file. Use Scanner and an appropriate regular expression.
4. Try running GreenhouseControls by:
- java GreenhouseControls -f examples1.txtu
and
java GreenhouseControls -f examples2.txt
5. The -f argument must be present. It must be either -f or -d. Please see Part 4. The event information must be in the format specified in examples1.txt and examples2.txt.
Step 3: Add functionality to simulate problems
1. Create a WindowMalfunction and PowerOut Events to simulate problems that may occur in a GreenhouseControls. The event should set the following boolean variables as appropriate in GreenhouseControls:
- windowok = false;
poweron = false;
After setting the variables, WindowMalfunction or PowerOut should throw an exception specifying the faulty condition. Create a ControllerException class that extends Exception for this purpose.
2. If an exception is thrown from WindowMalfunction or PowerOut, the Controller catches the exception, then initiates an emergency shutdown with an appropriate message. Add a method to Controller called shutdown, and override this method in GreenhouseControls to accomplish the shutdown.
3. Add an instance variable in GreenhouseControls called errorcode. It indicates the nature of the problem with an error code in an int variable errorcode (1 for WindowMalfunction and 2 for PowerOut), logs the time and the reason for the shutdown in a text file in the current directory called error.log and prints it to the console. It then serializes and saves the entire GreenhouseControls object in a file dump.out in the current directory before exiting.
4. Run the following to test this part:
- java GreenhouseControls f examples3.txt
and
java GreenhouseControls -f examples4.txt
Step 4: System restore
In this part, we add functionality for restoring the saved GreenhouseControls object and having it resume execution where it left off. It demonstrates the use of interfaces and the capability of Java methods to return objects.
1. Create the following interface
- interface Fixable {
// turns Power on, fix window and zeros out error codes
void fix ();
// logs to a text file in the current directory called fix.log
// prints to the console, and identify time and nature of
// the fix
void log();
}
2. Create inner classes PowerOn and FixWindow that implement Fixable. 3. Add a method to GreenhouseControls
4. Add a method within GreenhouseControls
5. Create a class Restore to restore the system after a shutdown. When this command line is called:
|
------------------------------------------ Current code -----------------------------------------
import java.util.List; import java.util.ArrayList; public class Controller { private final ListeventList = new ArrayList<>(); public void addEvent(Event c) { eventList.add(c); } public void run() { while (eventList.size() > 0) { for (Event e : new ArrayList<>(eventList)) { if (e.ready()) { System.out.println(e); e.action(); eventList.remove(e); } } } } }
import java.io.*; public abstract class Event { private long eventTime; protected final long delayTime; public Event (long delayTime) { this.delayTime = delayTime; start(); } public void start() { eventTime = System.nanoTime() + delayTime; } public boolean ready() { return System.nanoTime() >= eventTime; } public abstract void action(); }
import java.io.*; import java.util.Calendar; public class GreenHouseControls extends Controller { public class LightOn extends Event { public LightOn(long delayTime) { super (delayTime); } public void action() { light = true; } //to turn the light on public String toString() { return "Light is on."; } } public class LightOff extends Event { public LightOff(long delayTime) { super (delayTime); } public void action() { light = false; } //to turn light off public String toString() { return "Light is off."; } } public class WaterOn extends Event { public WaterOn (long delayTime) { super(delayTime); } public void action() { water = true; } //water on public String toString() { return "Greenhouse water is on."; } } public class WaterOff extends Event { public WaterOff(long delayTime) { super(delayTime); } public void action() { water = false; } //water off public String toString() { return "Greenhouse water is off."; } } public class ThermostatOn extends Event { public ThermostatOn(long delayTime) { super(delayTime); } public void action() { thermostat = "Night."; } //set thermostat to night public String toString() { return "Thermostat on Night Setting."; } } public class ThermostatOff extends Event { public ThermostatOff(long delayTime) { super(delayTime); } public void action() { thermostat = "Day"; } //set thermostat to day public String toString() { return "Thermostat on Day Setting."; } } public class FansOn extends Event { public FansOn(long delayTime) { super(delayTime); } public void action() { Fans = true; } //fans on public String toString() { return "Fans are on."; } } public class FansOff extends Event { public FansOff(long delayTime) { super(delayTime); } public void action() { Fans = false; } // fans off public String toString() { return "Fans are off."; } } public class Bell extends Event { public Bell(long delayTime) { super(delayTime); } public void action() { addEvent(new Bell(delayTime)); } public String toString() { return "Bing!"; } } public class Restart extends Event { private Event[] eventList; public Restart (long delayTime, Event[] eventList) { super(delayTime); this.eventList = eventList; for (Event e : eventList) { addEvent(e); } } public void action() { for (Event e : eventList) { e.start(); addEvent(e); } start(); addEvent(this); } public String toString() { return "Restarting System."; } } public static class Terminate extends Event { public Terminate(long delayTime) { super (delayTime); } public void action() { System.exit(0); } public String toString() { return "Terminating."; } } boolean Fans = false; private String thermostat = "Day"; private boolean water = false; private boolean light = false; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
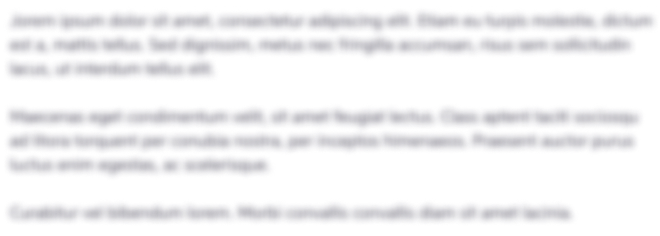
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started