Question
IN JAVA: Define a Cat class and a Dog class. The Cat class will have a function eat, (this is functionally a setter, like setHunger)
IN JAVA:
Define a Cat class and a Dog class. The Cat class will have a function eat, (this is functionally a setter, like setHunger) where the cat will set its satiety (satiety means how full you are, basically the opposite of hunger) to at most 100. The dog will also have a function eat, however, it can eat as much food as there it is given. You can feed the cat or dog by calling its function that has a parameter of how much food is given, however the cat will only eat as much as it takes to be full, while the dog will eat until it is bursting at the seams.
Create a Person class, with attributes name and age. The Cat and Dog will have an owner attribute, and they can react differently to different people. They both have a greet function, but the cat will only greet their owner.
Cats and Dogs may have different eating habits. A cat may only eat until its full, but a dog may eat everything it possibly can. Cats and Dogs can also react differently to different people. Let us imagine that a dog will greet anyone but a cat will only greet its owner.
Sometimes Cats can be friendly if you offer them treats. If a person who is not the owner tries to greet the cat with a treat, it will greet that person.
Sometimes we mix up peoples names. If someone has the same name as another person, and is close to the same age, we might mistake them for another person.
Part 1:
Create a person class. Give it the attributes name and age. Add the field Person friend to Person. Add a greet function to Person that takes in a parameter Person. If the person has the same name as friend and is within 5 years of age as friend. Print out Hello Friend. Otherwise, print whats your name.
Part 2:
Create a Dog class. The Dog has a String name, Person owner, and int satiety. Create a constructor for class Dog that takes in the parameters name, owner, and satiety. The Dog has a function eat, which takes in 1 parameter, food, as an int. When the function eat is called, the Dogs satiety increases by the amount of food given. The Dog has another function, greet, which takes in 1 parameter, person, as a Person object. When the function greet is called, the Dog prints a greeting in the console.
Part 3:
Create a Cat class. The Cat has a String name, Person owner, and int satiety. Create a constructor for class Cat that takes in the parameters name, owner, and satiety The Cat has a function eat, which takes in 1 parameter, food, as an int. When the function eat is called, the Cat satiety increases by the amount of food given, but never increases beyond 100. The Cat has another function, greet, which takes in 1 parameter, person, as a Person object. When the function greet is called, the Cat prints a greeting in the console only if the person is the same as its owner.
Part 4:
Add a field String favoriteTreat to the class Cat. Overload the constructor and create another one that can take a favoriteTreat. Also Overload the greet function and create another greet function that takes 2 parameters, person and treat. When the greet function is called, if its a person who isnt their owner, they will still greet them if the treat is the same as their favoriteTreat. If they owner is giving them their favoriteTreat, they will have a more ecstatic greeting. However, if they are full (their satiety is 100). They will ignore the person.
Part 5:
Finally, make everything come together. Create a Person object. Create a Dog and Cat object with the person as their owner. Call the greet function on the Dog with the person. Create a second Person. Call the greet function on the Cat with the second person. Call it again with a treat. Call the eat function for the Cat. Finally call the greet function on the Cat with the first person with a treat.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
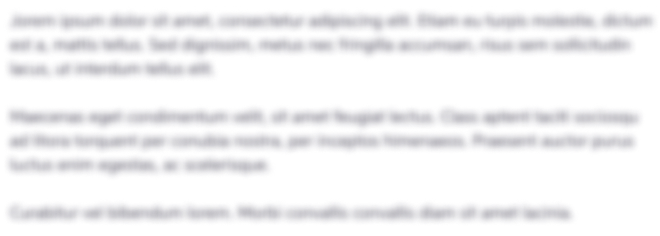
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started