Answered step by step
Verified Expert Solution
Question
1 Approved Answer
IN java FILE insects.txt CS 1450 Data Structures and Algorithms - Spring 2020 Assignment #3 Due Date: Feb 12, 2020 at 1:40pm (MW class), Feb
IN java
FILE insects.txt
CS 1450 Data Structures and Algorithms - Spring 2020 Assignment #3 Due Date: Feb 12, 2020 at 1:40pm (MW class), Feb 13, 2020 at 1:40pm (T/R class) Purpose: Learn to write abstract classes and interfaces. Practice using arrays and ArrayLists. Effort: Individual Points: 100 Deliverables: Upload a .zip file with the ONE source code file (.java file) to Canvas. Hand in a hardcopy version of the code and your design notes at beginning of class. Assignment Description The table below shows types of insects with different abilities - pollinators, builders, predators, and decomposers. Each insect has a fixed set of abilities as is listed in the table with a Y. Type Pollinator Builder Predator Decomposer Honeybee Y Y N Ladybug Ant NYYY Praying Mantis N N Y bug YN Specifications 1. Create a Java class called LastNameFirstName Assignment3 2. Follow "CS1450 Programming Assignments Policy" Read the specifications to get an overview of assignment. When writing code, create the classes 1'! 3. Write a test program (i.e. main) that performs the following steps: a. Creates a polymorphic array to store the insect objects. b. For each line in the file insects.txt: i. Read the information for the insect. ii. Create an insect object. iii. Place the insect object into array. c. AFTER all insect objects are in the array, perform these 2 tasks on the array of insects: i. Find the insects that do not help with the decomposition process ii. Find the one insect with the highest level of all abilities combined For task i, create a method that takes the array of all insects and returns an ArrayList of specific insects (non-decomposers). For task i, create a method that takes the array of all insects and returns an ArrayList of specific insects (non-decomposers). public static ArrayList findDoNotDecompose(Insect() insects) For task ii, create a method that returns the array index of the most able insect: public static int findMostAble(Insect() insects) Also create a method to display the abilities of an insect. Display the values of the abilities associated with the insect sent to method. public static void display Abilities(Insect insect) NOTE: be sure to place these 3 methods inside the Assignment3 class, right after main. d. Displays on the console the following for each method in step 3c (see output below) Insect's name Insect's type Insect's ability i.e. pollinator) Insect's purpose (use abstract purpose() method) Insect's numeric ability level 4. Test file information: a. Run your code on the provided test file insects.txt b. This file is an example so DO NOT assume that your code should work for only 5 insects in the order specified in the file. Represents number of Details for insects in file h Honey 9800 a Flix 0 10 7 10 each insect. See info p Manny 0 0 100 format of the line a Queen 0899 1 Francis 9080 below about the c. l line is an integer value representing the number of insects in the file d. Remaining lines contain details for each insect. The format is as follows: h Honey 9 8 0 0 Name Pollinate Build Predator Type Decompose Ability Ability Ability Ability e. Note: If any ability is zero (pollinate, build, predator, decompose) then the insect does not have that ability (i.e., it's not part of its nature). For example: Honeybees are not decomposers; so their decompose ability is set to 0. Interfaces Pollinator, Builder, Predator, and Decomposer Interfaces Description Each interface represents a specific ability such as pollinator, builder, etc. The specific ability is measured as a numeric value from 0 to 10. . For example, Ants are excellent decomposer with decompose abilities of 10, or 9 etc. but not so good at pollinating so their pollinate ability is a 0. You may assume no two insects will have the same ability levels. Public Methods Pollinator interface has a method named pollinate() Returns integer value representing ability to pollinate Builder interface has a method named buildo Returns integer value representing ability to build nests, etc. Predator interface has a method named predator() Returns integer value representing ability to eat other insects/plants Decomposer interface has a method named decompose) Returns integer value representing ability to decompose dead animal/plants Classes Insect Class Description ABSTRACT class that represents a generic insect. Superclass in the hierarchy. Private Data Fields type - String for type of insect (i.e. Honeybee, Ladybug, etc.) name - String for insect's name Public Methods Constructor - none . Unlike assignment #2, here setters are provided in the Insect class to set the instance variables so no constructor is needed. Getter for each private data field Setter for each private data field ABSTRACT method purpose() Returns string describing insect's purpose. Honeybee, Ladybug, Ant, and Praying Mantis Subclasses Description Each represents a specific insect-these are the subclasses in the hierarchy. o Each must extend Insect. o Each must implement only the interfaces for the insect's specific abilities. Private Data Fields Instance variables for each ability the specific insect (subclass) is known for. . For example, a honeybee needs a pollinate Ability and buildAbility (see 1 table above). Public Methods Constructors Takes the name of insect and the values for ONLY the abilities that insect has. Example: Honeybee constructor takes: name, pollinate Ability and build Ability. public Honeybee(String name, int pollinateAbility, int buildAbility) Subclass implements ONLY the interfaces for the abilities that insect is known for. . Example: Honeybee class implements ONLY Pollinator and Builder. . This means Honeybee must override pollinate() and build() interface methods Each subclass overrides the purpose() method in the abstract Insect class. . Use the following strings for each insect's purpose. Type Purpose Honeybee I'm popular for producing honey but I also pollinate 35% of the crops! Without me, 1/3 of the food you eat would not be available! Ladybug Named after the Virgin Mary, I'm considered good luck if I land on you! I'm a pest control expert cating up to 5,000 plant pests during my life span. Ant Don't squash me, I'm an ecosystem engineer! Me and my 20 million friends accelerate decomposition of dead wood, aerate soil, improve drainage, and eat insects like ticks and termites! Praying Mantis I'm an extreme predator quick enough to catch a fly. Release me in a garden and I'll eat beetles, grasshoppers, crickets and even pesky moths. Tips Must Do Use an array to store the insects you create from the information in the file. Use an ArrayList to retum insects from the methods specified in step 3c. Do not assume the file provided is what the grader will use. It is important to learn to not make assumptions when writing code. For example, do not assume there are 5 insects and do not assume the insects will be in the order they are presented in the file. Tip: Casting The methods find MostAble and display Abilities, need access to an insect's abilities so you will need to call the methods in the interfaces to obtain the abilities. To call a method in the interfaces, casting to Pollinator, Builder, Predator or Decomposer is required. o For example, to obtain the insect's pollinate ability, casting to Pollinator is required. o Why? Because the pollinate method is in the Pollinate interface and not in Insect class. o That is, when calling the insect's pollinate method on an insect object, you'll need to cast otherwise you'll get the error: "the method pollinate() is undefined for type Insect" Read Liang, section 11.9 Casting Objects and the instanceof Operator o Look at Listing 11.7, it contains both instanceof and casting. This should help you understand what is required to determine which interface you are working with in the findDoNotDecompose, find MostAble, displayAbilities. Tip: Build the classes first and Code Incrementally Before writing the code in main, build as much of the classes as possible Create a skeleton of each class before you write the code to create insects in main. Next get the code working in bits. Make sure you can read all the data from the file correctly, then create the insect objects, etc. Output Your output will look like the following when running against the provided test file insects.txt INSECTS THAT DON'T HELP WITH DECOMPOSITION! Honey is a Honeybee and does not help with decomposition I'm popular for producing honey but I also pollinate 35% of the crops! Without me, 1/3 of the food you eat would not be available! Pollinating ability 9 Building ability 8 Manny is a Praying Mantis and does not help with decomposition I'm an extreme predator quick enough to catch a fly. Release me in a garden and I'll eat beetles, grasshoppers, crickets and even pesky moths Predator ability 10 Francis is a Ladybug and does not help with decomposition Named after the Virgin Mary, I'm consider good luck if I land on you! I'm a pest control expert eating up to 5,000 plant pests during my life span. Pollinating ability 9 Predator ability 8 INSECT WITH MOST ABILITIES! The winner is Flix the Ant Don't squash me, I'm an ecosystem engineer! Me and my 20 million friends accelerate decomposition of dead wood, aerate soil, improve drainage, and eat insects like ticks and termites! Building ability 10 Predator ability 7 Decomposer ability 10 h Honey 9 8 0 0 a Flix 0 10 7 10 p Manny 0 0 10 0 a Queen 0 8 9 9 1 Francis 9 0 8 0







FILE insects.txt

Step by Step Solution
There are 3 Steps involved in it
Step: 1
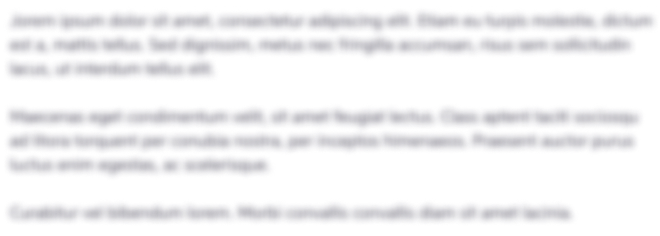
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started