Question
In Java I have to do the following: code your LoanProcessing class file. For your LoanProcessing source file make sure to extend BankRecords . Include
In Java I have to do the following:
code your LoanProcessing class file.
For your LoanProcessing source file make sure to extendBankRecords.
Include the following methods for your file.
Include in main() your readData() method call which will process your BankRecord objects. Theninstantiate a Dao object and trigger your createTable() method,your insertRecords(your BankRecords array object)method and retrieveRecords() method in that order. Of course you can comment out your createTable / insertRecords once youve accomplished that to test how your output statements appear. Logic for that follows next.
Once you have retrieved a recordset, print out all the records from the recordset to the console in a nice columnar format included with heading names for id, income and pep. Doesnt hurt to have a title too, like Loan Analysis Report.
Sample starter code for main (fill in code where comments are shown to do so):
BankRecords br = new BankRecords();
br.readData();
Dao dao = new Dao();
dao.createTable();
dao.insertRecords(robjs); // perform inserts
ResultSet rs = dao.retrieveRecords(); // fill result set object
// Create heading for display
// Extract data from result set
while (rs.next()) {
// Retrieve data by column name (i.e., for id,income,pep)
// Display values for id,income,pep
}
rs.close();// closes result set object
Extra Credit options
-Include SQL Prepared statements when inserting records (+5 points)
-Include besides console, output a JTable GUI output display of your recordset
data(+5 points)
For a JTable to trigger code, build a frame with Javax Swing components which will
display your loan data into a JTable.Column headings must be included as well as a
scrollbar for proper appearance of your display within a GUI frame showing your table
component.
Oryou for extra credit you may choose the following option
-Serialize and deserialize BankRecord objects using the java.util.Map class. (+10 points)
Name your serializable file bankrecords.ser. Make your application sleep for 5 seconds
between the processes. Display to the console the time difference between the serialization
and deserialization processes.?
Include a zip file of allyour project source code(new and old), plus jar filein an orderly fashion and a snapshot of your app at runtime which must include a table creation message, an insertion message and the first few rows of your record results showing the Loan Analysis Report data into a doc file into BB for credit.If you have any extra credit,snapshot that as well and label your snapshot(s) accordingly.
How do I code the remaining part of the code given above to have everything the code requires to have?
The three other files of the project:
Client:
public abstract class Client {
abstract void readData();
abstract void processData();
abstract void printData();
}
Records:
import java.io.FileWriter;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Arrays;
import javax.swing.JOptionPane;
public class Records extends BankRecords{
private static FileWriter fw = null;
public Records()
{
try
{
fw = new FileWriter("bankrecords.txt");
}
catch(IOException e)
{
e.printStackTrace();
}
}
//male Comparator
private static void maleComparator()
{
Arrays.sort(inforec, new MaleComparator());
int MaleInner = 0, MaleSuburbun = 0, MaleTown = 0, MaleRural = 0;
for(int i=0; i < inforec.length; i++)
{
if(inforec[i].getgender().equals("MALE") && inforec[i].getregion().equals("INNER_CITY")&& inforec[i].getauto().equals("YES") && inforec[i].getChild() == 1)
MaleInner++;
}
System.out.println("Males with car and 1 children in INNER_CITY: "+ MaleInner);
for(int i=0; i < inforec.length; i++)
{
if(inforec[i].getgender().equals("MALE") && inforec[i].getregion().equals("TOWN")&& inforec[i].getauto().equals("YES") && inforec[i].getChild() == 1)
MaleTown++;
}
System.out.println("Males with car and 1 childern in TOWN: " + MaleTown);
for(int i=0; i < inforec.length; i++)
{
if(inforec[i].getgender().equals("MALE") && inforec[i].getregion().equals("RURAL")&& inforec[i].getauto().equals("YES") && inforec[i].getChild() == 1)
MaleRural++;
}
System.out.println("Males with car and 1 children in RURAL: " + MaleRural);
for(int i =0; i < inforec.length; i++)
{
if(inforec[i].getgender().equals("MALE") && inforec[i].getregion().equals("SUBURBAN")&& inforec[i].getauto().equals("YES") && inforec[i].getChild() == 1)
MaleSuburbun++;
}
System.out.println("Males with car and 1 children in Suburban: " + MaleSuburbun);
try
{
fw.write(" Males with car and 1 children in INNER_CITY: "+ MaleInner + " " + "Males with car and 1 childern in TOWN: " + MaleTown + " " + "Males with car and 1 children in RURAL: " + MaleRural + " Males with car and 1 children in Suburban: " + MaleSuburbun + " " );
}
catch (IOException e)
{
e.printStackTrace();
}
}
//Female with mortage and savings
private static void femaleComparator()
{
Arrays.sort(inforec, new FemaleComparator());
int female = 0;
for(int j=0; j < inforec.length; j++)
{
if(inforec[j].getgender().equals("FEMALE") && inforec[j].getMortage().equals("YES") && inforec[j].getsavings().equals("YES"))
female++;
}
System.out.println(" Females with Mortage: " + female);
System.out.println(" Females with Savings: " + female);
try
{
System.out.println("Information:");
fw.write(" Females with mortgage : " + female);
fw.write("Female Savings" + female);
}
catch (IOException e)
{
e.printStackTrace();
}
}
// The Avergae Income for Comparator
public static void AvgIncomeComparator()
{
Arrays.sort(inforec, new IncomeComparator());
double incomeave = 0, sum = 0;
for(int j =0; j < inforec.length; j++)
{
sum += inforec[j].getIncome();
}
incomeave = sum / inforec.length; //average of income sum
System.out.println(" Average Income is : " + incomeave);
try
{
fw.write("Average Income is : " + incomeave + " ");
}
catch(IOException e)
{
e.printStackTrace();
}
}
public static void main(String[] args) throws IOException
{
Records obj = new Records();
obj.readData();
femaleComparator();
maleComparator();
AvgIncomeComparator();
try{
fw.close();
}
catch(IOException e){
e.printStackTrace();
}
}
}
Bank Records:
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class BankRecords extends Client{
static BankRecords inforec[];
List> array = new ArrayList
>();
//declaring variables
String id;
int age;
String gender;
double income;
String married;
int child;
String auto;
String save_act;
String current_act;
String mortage;
String savings;
String pep;
String region;
//Reads Data
void readData()
{
String Line = "";
try(BufferedReader br = new BufferedReader (new FileReader("D:/bank-Detail.csv")))
{
int i = 0;
while((Line = br.readLine()) != null)
{
array.add(Arrays.asList(Line.split(",")));
}
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
processData();
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getgender() {
return gender;
}
public void setgender(String gender) {
this.gender = gender;
}
public String getregion() {
return region;
}
public void setregion(String region) {
this.region = region;
}
public double getIncome() {
return income;
}
public void setIncome(double income) {
this.income = income;
}
public String getMarried() {
return married;
}
public void setMarried(String married) {
this.married = married;
}
public int getChild() {
return child;
}
public void setChild(int child) {
this.child = child;
}
public String getauto() {
return auto;
}
public void setauto(String auto) {
this.auto = auto;
}
public String getSave_act() {
return save_act;
}
public void setSave_act(String save_act) {
this.save_act = save_act;
}
public String getCurrent_act() {
return current_act;
}
public void setCurrent_act(String current_act) {
this.current_act = current_act;
}
public String getPep() {
return pep;
}
public void setPep(String pep) {
this.pep = pep;
}
public String getMortage() {
return mortage;
}
public void setMortage(String mortage) {
this.mortage = mortage;
}
public String getsavings() {
return savings;
}
public void setsavings(String savings) {
this.savings = savings;
}
// Process Data
void processData()
{
int i = 0;
inforec = new BankRecords[array.size()];
for(List
{
inforec[i] = new BankRecords();
inforec[i].setId(row.get(0));
inforec[i].setAge(Integer.parseInt(row.get(1)));
inforec[i].setgender(row.get(4));
inforec[i].setregion(row.get(5));
inforec[i].setIncome(Double.parseDouble(row.get(6)));
inforec[i].setMarried(row.get(6));
inforec[i].setChild(Integer.parseInt(row.get(6)));
inforec[i].setauto(row.get(9));
inforec[i].setSave_act(row.get(9));
inforec[i].setCurrent_act(row.get(9));
inforec[i].setMortage(row.get(12));
inforec[i].setsavings(row.get(12));
inforec[i].setPep(row.get(11));
i++;
}
//Data would be printed
}
void printData()
{
System.out.println("ID\t\tAge\t\tSex\t\tRegion\t\tIncome\t\tMortgage\tsavings" );
for (int i=0; i<=24; i++)
{
String Final = String.format( "%s\t\t%s\t\t%s\t\t%-10s\t%8.2f\t%s",inforec[i].getId(),inforec[i].getAge(),inforec[i].getgender(),
inforec[i].getregion(),inforec[i].getIncome(), inforec[i].getMortage());
System.out.println(Final);
}
}
public static void main(String[] args) throws IOException
{
BankRecords sc = new BankRecords();
sc.readData();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
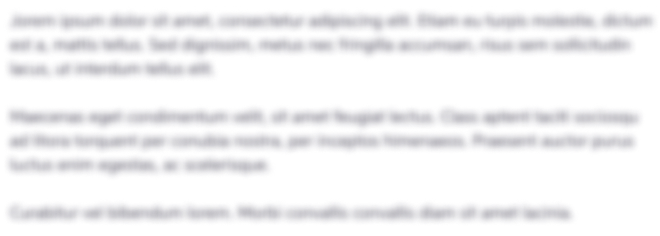
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started