Question
In Java, implement a simulation of a universitys registrars office. The user program to test the circular array based queue and stack using a linked
In Java, implement a simulation of a universitys registrars office. The user program to test the circular array based queue and stack using a linked list, is provided below. You will simulate a typical days worth of processing in two ways, by using a queue and by using a stack, to see if one approach provides a lower average wait time and a lower longest wait time. The simulation works as follows:
For each minute that the office is open (8 hours or 480 minutes)
Determine if any new students enter the office and for each new student,
add the student to the waiting line (enqueue for the queue, push for the stack) add 1 to the number of students in the simulation
For each registrar, if that registrar is currently processing a student, reduce the students process time by 1 minute
else
remove the next student from the line (dequeue or pop)
compute the students wait time (current time - time entered line) add this to total wait time
if this wait time > longest wait time, save as new longest wait time determine the students processing time
The loop iterates until these three conditions are all true: current time >= 480, line is empty and all registrars are all free. This means that once the time elapses, the simulation continues until all students still waiting have been serviced. However, after 480 minutes, no new students are allowed to enter the line.
The simulation should end by outputting the following information:
Number of registrars used in this simulation
Number of students serviced
Average wait time
Longest wait time
Run your simulation for 2, 3, 4, and 5 registrars using a queue. Repeat the simulation using a stack. You might use an outer for loop in your program to iterate through the number of registrars for convenience so that you only need to run it once for each of the queue and stack rather than running each 4 times.
Both the queue and the stack will just store int values. The int stored will be the time the student enters the line. In this way, when you remove the student (the int), you can compute the students wait time as the current time the value stored in the queue/stack.
Implement your stack using a linked structure (which requires defining a Node class, which can be the same as the Node used in program 1, or a nested inner class). Implement the queue using a circular array.
Here is output from my program. Notice how the average wait time was more for the queue but the longest wait time was more for the stack. I also included in my output the average processing time for each student (number of minutes each student took). You do not need to output this but I found it helpful when debugging my program. Make sure you format any doubles/floats to limit the number of decimal points of accuracy and format the output so that it lines up fairly nicely.
Using the queue:
Registrars | Students | Avg Wait | Avg Process | Longest |
2 | 646 | 268.020 | 2.228 | 560 |
3 | 589 | 85.660 | 2.319 | 174 |
4 | 584 | 10.029 | 2.336 | 27 |
5 | 581 | 0.525 | 2.267 | 4 |
Using the stack:
Registrars | Students | Avg Wait | Avg Process | Longest |
2 | 613 | 240.754 | 2.116 | 954 |
3 | 553 | 47.781 | 2.193 | 580 |
4 | 604 | 12.619 | 2.262 | 411 |
5 | 601 | 1.032 | 2.133 | 67 |
User program to test stack and queue:
import java.util.Random;
public class RegistrarSimulation
{
public static void main(String[] args)
{
//MyQueue q; // the queue-based waiting line
MyStack s; // the stack-based waiting line
Random g = new Random();
int time, temp, num, i, wait, numStudents,totalWait, longest, totalProcess, freeReg; // time is the elapsed time from 0 to 480 (or beyond), temp stores random values,
// wait is this student's wait time while totalWait is all students wait time combined, freeReg, longest is the
// longest wait time and totalProcess is total amount of time all students were serviced for. The variable freeReg counts how
// many registrars are free. Once 480 minutes elapse and all of the registrars are free, we can stop simulating.
double averageWait, averageProcess; // the averages are what we want our simulation to tell us: average wait time and average servicing time
int[] registrar=new int[6]; // we will test registrars from 2 to 5 so we need as many as 4 registrars
for(i=2;i<6;i++) registrar[i]=0; // initialize all to 0 meaning they are all currently free to start the simulation, note that we will not be using registrar[0] or registrar[1]
System.out.println("Registrars Students Avg Wait Avg Process Longest"); // output headers
for(int numRegistrars=2;numRegistrars<6;numRegistrars++) // iterate through all of the registrars we will be testing (2-5)
{
//q=new MyQueue(1000); // this will simulate using a queue, comment this out and uncomment the next line to test the stack
s=new MyStack();
time=0;numStudents=0;totalWait=0;longest=0;totalProcess=0;freeReg=0; // initialize all variables used for numRegistrars
//while(time<480||!q.isEmpty()) // simulate while we still have time and the waiting line is not empty, for the stack, change q to s
while(time<480||!s.isEmpty())
{
if(time<480) // during the first 8 hours, still admit new students to the line
{
temp=g.nextInt(100); // randomly generate number of students
if(temp<35) num=0;
else if(temp<53) num=1;
else if(temp<84) num=2;
else if(temp<97) num=3;
else num=0;
numStudents+=num; // add these students to the total number we have processed so far
for(i=0;i //q.enqueue(time); // comment this instruction and uncomment the next for the stack version s.push(time); } // at this point, we process students either with a registrar or waiting in line for(i=2;i<=numRegistrars;i++) // for each registrar, if currently handling a student, subtract 1 from the number of minutes left to service the student { if(registrar[i]>0) registrar[i]--; // this registrar helps his/her current student for 1 more minute else { // this registrar is free //if(!q.isEmpty()) // is there a student waiting in line? If so, move that student to this registrar if(!s.isEmpty()) // comment out the previous instruction and uncomment this one to switch from queue to stack { //temp=q.dequeue(); // retrieve next student from line, comment this instruction and uncomment next temp=s.pop(); // to switch from queue to stack wait=time-temp; // the value recorded in the queue/stack was the time when the student entered the line, time-temp is the student's wait time if(wait>longest) longest=wait; // add that time to total wait and see if that wait time is longer than the previous longest and if so, remember it totalWait+=wait; temp=g.nextInt(100); // randomly determine how much time this student will require with this registrar if(temp<30) num=1; else if(temp<63) num=2; else if(temp<86) num=3; else if(temp<94) num=4; else if(temp<98) num=5; else num=6; registrar[i]=num; // set this registrar to the number of minutes this student will need totalProcess+=num; // totalProcess is the total amount of time all students need } } } time++; // this minute is now over } // having reached here, 480 minutes have elapsed and then all of the waiting students have also been processed, compute and output our results averageWait=(double)totalWait/numStudents; averageProcess=(double)totalProcess/numStudents; // note: we add 2 to numRegistrars since numRegistrars is equal to 0-3 but should be 2-5 System.out.printf("%5d %6d %7.3f %7.3f %6d ", numRegistrars, numStudents, averageWait, averageProcess, longest); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
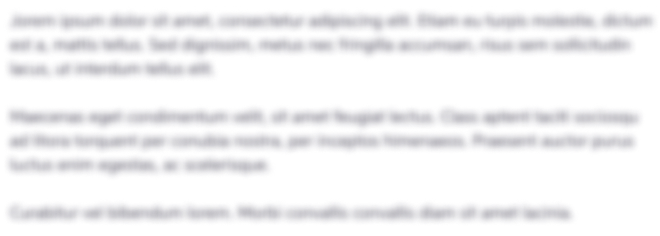
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started