Question
IN JAVA: Please do not use StringBuilder, and please use if else statements, switch statements, for loops, while loops, and arrays only:) Lab 04: Cryptography
IN JAVA: Please do not use StringBuilder, and please use if else statements, switch statements, for loops, while loops, and arrays only:)
Lab 04: Cryptography - Caesar Cipher Lab by: Brady Jacobs
In this lab, you will create a program to encrypt a secret message using a special CPSC 1060 variation of Caesar cipher. Terminology: Cryptography: study and practice of techniques for secure communication using encryption Plaintext: unencrypted text Ciphertext: encrypted text containing a secret message Background: Supposedly, Julius Caesar used to encrypt his messages for military instruction by shifting each letter in his messages by some number k. For instance, he might have written A as B, B as C, C as D, and then circling-back to change Z to A if k = 1. Examples: if k = 1: abc becomes bcd if k = 25: abc becomes zab if k = 14: hello world becomes vszzc kcfzr This is commonly called a Caesar Cipher. This Caesar cipher is easily broken since there are only 26 possible values for k since there are 26 letters in the alphabet. We could even write a Java program to brute-force a solution to a Caesar cipher. Still, it worked very well back in Caesars day because he relied on most of his enemies being illiterate. Another exciting way to break the Caesar cipher is to look at the English languages distinctive letter frequency distribution. The diagram below shows this distribution. Given a lengthy message, you would see a noticeable shift in this distribution and could predict the key used. Our goal in this lab is to apply the Caesar cipher and encrypt plaintext messages using a key; however, in hopes to make this encryption slightly more challenging to break, we will take the second half of the ciphertext and move it to the front. Tuesday: If desired, find a partner to work with In the CPSC1061 Canvas, under Assignments find Lab 4 Pre-Code Questions Complete the pre-lab activity As you proceed through the activity, ask yourself the following for each question: (You are encouraged to take notes and discuss your thoughts with your partner!) What type of variable do I need for these values? How many variables do you need? What would you name them? How would you write and solve these questions in Java? Be sure to submit before Wednesday 11:59 pm Program Specification: Implement your program using the template code from Canvas under Lab04. Make sure to extract the code before transferring it to VS Code. Your program should prompt users for a key to encode the message. This key is a non-negative integer. If a user enters input that is not an integer, the program should print an error message and exit to avoid throwing an exception. If a user enters a negative integer, print an error message and loop until the user enters valid input. Your user will then prompt the user for an input message to encode. Do not assume that k will be less than or equal to 26. Your program should work for all non-negative integer values. Do not worry if a user tries to enter an abnormally large number that would overflow an int in Java. We wont be trying to break your code. For instance, if k is 27, A should not become [ even though A + 27 is [ in ASCII. Instead, A should become B since B is 27 places away from A since Z wraps back around to A. Your program should preserve the case: capitalized letters should stay capitalized, and lowercase letters should remain lowercase. Your program should preserve punctuation and whitespace, meaning non-alphabetical characters should be unchanged. Your program should output the Caeser ciphertext and then perform the substring swap and print the final ciphertext. Your program should loop to ask the user for new plaintext to encrypt with the same key after the first iteration of the loop. Thursday: 1. Review your notes/Pseudocode from Tuesday. 2. Accessing the Key Prompt the user to enter the key they would like to encrypt with. Make sure you print the error statements and loop adequately based on the input from the user. 3. Caesar Cipher It is pretty easy for us to understand that A + 1 = B, but how can we communicate that same idea in Java code? Lets start by ignoring the key for now and just try to take in a message to be encrypted from the Scanner object and shift each character by one and print that as the Caesar ciphertext. The main idea here is to learn how to loop through a string and access each character and apply the desired shift of 1. After this, lets apply the last part to our special encryption process: take the second half of the cipher and move it to the front using substring in Java. 4. Putting it All Together If you have done all the previous steps, you will have a good understanding of where to go next. The last part is putting together the Caesar Cipher algorithm and adding a loop to ask for further messages to be encrypted. Remember to have clear comments, a clearly defined header, and well-formatted code. 5. Submit to Gradescope Make sure to submit before 11:59 pm on Monday, February 13th. Make sure your output matches how the test case output is formatted. TA Tips: Best to use the modulo (i.e., remainder) operator, %, to handle the wraparound from Z to A. Recall that ASCII maps all printable characters to corresponding numbers and that A = 65 while a = 97. Feel free to look up the ASCII table to plan your code accurately. Here are some sample interactions with the program. The users input is in blue:
Example 1: Key Value: xyz
Usage: Please enter a valid non-negative integer
Example 2: Key Value: -1
Usage: Try again, please enter a valid non-negative integer
Key Value: -3
Usage: Try again, please enter a valid non-negative integer
Key Value: 13
String to encode with key 13: hello, world
Caesar Cipher: uryyb, jbeyq
Ciphertext: jbeyquryyb,
Type q to quit, any other to continue: q
Example 3: Key Value: 100
String to encode with key 100:
Hello, World
Caesar Cipher: Dahhk, Sknhz
Ciphertext: SknhzDahhk,
Type q to quit, any other to continue: y
Key Value: 1 String to encode with key 13:
HELLO
Caesar Cipher: IFMMP
Ciphertext: MMPIF
Type q to quit, any other to continue: q
Step by Step Solution
There are 3 Steps involved in it
Step: 1
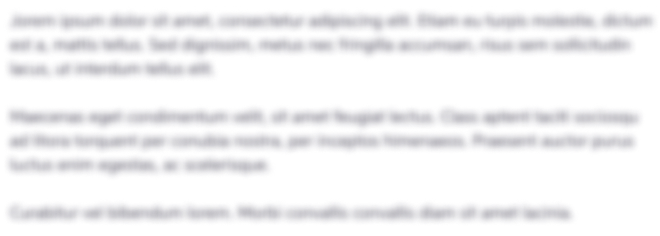
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started