Question
***In Java, please. I already have code for this problem that works, with the exception of tons of sand. I also posted my code if
***In Java, please. I already have code for this problem that works, with the exception of "tons of sand". I also posted my code if it helps. I need help obtaining the correct outputs for both examples. I appreciate some assistance in deriving the correct output that you can find below.
Problem Description and Given Info
Gene's Green Golf Center is remodeling their golf course, and has hired you to help write a program that will be used to determine what materials will be needed to complete the project. There are 18 holes on the golf course, but Gene likes to keep things simple, so every hole looks very similar. A typical Hole at Gene's Green Golf Center looks like the image below - only the length and width change.
Given the length and width of a hole (in yards), Gene needs to know the following:
Total square yards of rough sod, rounded up to the nearest square yard.
Total square yards of smooth sod, rounded up to the nearest square yard.
Tons of sand needed for the sand trap (US tons), rounded up to the nearest ton.
Number of bushes needed, rounded down to the nearest bush.
The program should take, as inputs from the user, the length and the width of a hole (both in yards). The program should then perform all necessary calculations and produce a tidy report with all of the required information regarding materials needed to construct the hole.
Other Details
Gene will provide the length and width of the hole in yards.
The Tee area is a circle with a diameter that is always one-third of the course width.
The Putting Green is a circle with a diameter that is always two-thirds of the course width.
The Sand Trap is a circle with a diameter that is always one-quarter of the course width.
The Sand Trap is always two foot deep.
Sand weighs 80 lbs. per cubic foot.
The course is surrounded on all sides by Bushes. Gene wants to plant one bush for every yard of the course perimeter, leaving two openings - one for the Entry Trail, and one for the Exit Trail. These two openings are both one yard wide.
Part 9 - Full Program
Design and construct a complete program for Gene's Green. Use the methods that you created in the previous Individual Assignments.
Additional Methods
You may also find it helpful to design and write some additional methods to do each of the following:
compute the total area of smooth sod, given the total length and width of a hole as arguments
the total area of smooth sod is equal to the area of the tee plus the area of the putting green
compute the total area of rough sod, given the total length and width of a hole as arguments
the total area of rough sod is equal to the total area of the hole (length times width) minus the areas of the tee, the putting green, and the sand trap
compute the total tons of sand needed, given the total length and width of a hole as arguments
to compute the tons of sand needed, you will need to compute the total square feet of the sand trap. With the total square feet, you can compute the total cubic feet of sand needed. From the total cubic feet of sand needed, you can compute the total pounds of sand needed. From the total pounds of sand needed, you can compute the tons of sand needed.
Each of the methods described in the list above could call one or more of the methods you already wrote (e.g. areaOfRectangle, areaOfCircle, etc.) as helper methods to compute some needed values.
You will also need to implement the code necessary to collect and store the program inputs. Then you will need to implement the code that will call your methods to compute all the required program outputs. Finally, you will need to implement the code to print out a nicely formatted report with all of the information that Gene needs.
Here are some examples of what Gene should see when he runs your program.
Example 1
Enter Course Length : 20 Enter Course Width : 10 Total square yards of rough sod : 152 Total square yards of smooth sod : 44 Tons of sand : 4 Number of bushes : 58
Example 2
Enter Course Length : 20 Enter Course Width : 20 Total square yards of rough sod : 206 Total square yards of smooth sod : 175 Tons of sand : 15 Number of bushes : 78
Make sure that your program collects the two required inputs in this order:
Course length (yards)
Course width (yards)
Helpful Hints:
Make sure that your program outputs the amount of materials needed as four lines of text, formatted exactly as shown(including spelling, capitalization, spaces, and punctuation).
There are 2,000 pounds in a ton
There are 9 square feet in a square yard
Remember that when you divide an int by an int you will always get a truncated (rounded down) int
The Math.ceil method may be useful for rounding up
My Code:
import java.util.Scanner;
public class GenesGreen { public static void main(String[] args) { // declare and create your Scanner Scanner scnr = new Scanner(System.in); // prompt for and collect the required inputs System.out.print("Enter Course Length : "); double length = scnr.nextDouble(); System.out.print("Enter Course Width : "); double width = scnr.nextDouble(); // call your methods to compute the required outputs double product = length * width; double totalAreas = (areaOfTee(length, width) + areaOfPuttingGreen(length, width) + areaOfSandTrap(length, width)); double roughSod = Math.ceil((product - totalAreas)); double smoothSod = Math.ceil(areaOfTee(length, width) + areaOfPuttingGreen(length, width)); double perimeter = width + width + length + length; double totalsqft = totalSquareFeetOfSandTrap(width); double tonsOfSand = Math.ceil(totalsqft * 3.0 * 80.0 * 2.0 / 2000.0); System.out.println("Total square yards of rough sod : " + (int)roughSod); System.out.println("Total square yards of smooth sod : " + (int)smoothSod); System.out.println("Tons of sand : " + (int)tonsOfSand); System.out.println("Number of bushes : " + (int)(perimeter -2)); } // display the results public static double areaOfTee(double length, double width){ double diameter = 1.0/3.0 * width; double radius = diameter / 2.0; double totalTeeArea = 3.141592653 * radius * radius; return totalTeeArea; } public static double areaOfPuttingGreen(double length, double width){ double diameter = 2.0/3.0 * width; double radius = diameter / 2.0; double totalPuttingGreenArea = 3.141592653 * radius * radius; return totalPuttingGreenArea; } public static double areaOfSandTrap(double length, double width) { double diameter = width * 1.0/4.0; double radius = diameter / 2.0; double totalSandTrapArea = 3.1415926 * radius * radius; return totalSandTrapArea; } public static double totalSquareFeetOfSandTrap(double width){ double diameter = width * 1.0/4.0; double radius = diameter / 2.0; double totalsqft= radius * 2.0 * 3.1415926; return totalsqft; } // copy your method definitions from the previous parts here below public static double tonsOfSand(double length, double width) { double sandTrapRadius = width / 4.0; double sandTrapArea = areaOfCircle(sandTrapRadius); double sandTrapVolume = sandTrapArea * 2; double sandTrapWeight = sandTrapVolume * 80; return sandTrapWeight / 2000.0;} public static double areaOfCircle(double radius) { return Math.PI * Math.pow(radius, 2); } // add any additional methods you may want here below below }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
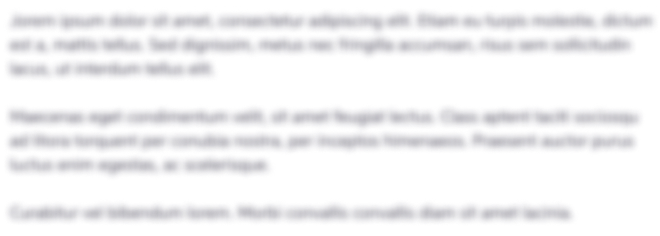
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started