Question
In Java Please Use the Student class provided. Write the compareTo( ) method to compare Students by ID. Create and array of five Student addresses.
In Java Please
Use the Student class provided. Write the compareTo( ) method to compare Students by ID. Create and array of five Student addresses. Sort the students by their ID. Rewrite the compareTo( ) method so the comparison is based on full name. Run the sort again.
The data must be read from a text file.
The Student class is :
public class Student {
private String lastName; private String firstName; private int ID; private double gpa; private int creditHours; Student[] studentArray = new Student[7]; public Student() { lastName = new String("unknown"); firstName = new String("unknown"); ID = 0; gpa = 0.0; creditHours = 0;
}
public Student(String inLast, String inFirst, int inID, double inGPA, int inCredits) { lastName = new String(inLast); firstName = new String(inFirst); ID = inID;; gpa = inGPA > 0?inGPA:0; creditHours = inCredits> 0?inCredits:0; }
public String toString( ) { return " Student Name: " + this.lastName + ", " + firstName + "\tID: " + this.ID + "\tGPA: " + this.gpa + "\tCredits: " + creditHours; }
//The equals( ) method must return a boolean. //When you write this code, you determine what fields to //use to determine if two Student objects should be consisdered equal. //Notice the paramter is a reference to another Student //Write this equals( ) method based on the ID field //The compareTo( ) method must return an int. //When you write this code, you determine what fields to //use to determine the ordering of two Student objects. //Notice the paramter is a reference to another Student //Write the compareTo( ) method based on the name fields
public void setGPA(double inG) { gpa = inG >= 0? inG:gpa; }
public void setCredits(double inCredits) { gpa = inCredits >= 0? inCredits:inCredits; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
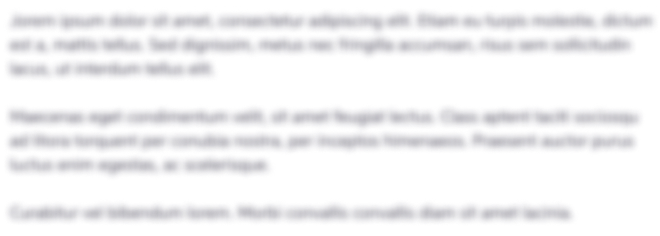
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started