Question
In Java, really need help with the Customer class The Date class. The Date class will represent a date. (Java has both Date and Calendar
In Java, really need help with the Customer class
- The Date class. The Date class will represent a date. (Java has both Date and Calendar in the API, but both are more complex than needed for this homework.) A Date contains a day, month, and a year. The Date class should have the following methods:
-
getDay: takes no input and returns an int that is the day of the date. The day should be a value between 1 and 31.
-
getMonth: takes no input and returns an int that is the month of the date. The month should be between 1 and 12.
-
getYear: takes no input and returns an int that is the year of the date.
-
incrementDay: takes no input and returns nothing. The method adds 1 to the day of the date. If the day exceeds the number of days for the month (assume no leap years), the day is set to 1 and the month is incremented. If the month exceeds 12, the month is set to 1 and the year is incremented.
-
toString: overrides the toString method inherited from Object so that the string returned is the date in "xx/xx/xxxx" format.
-
equals: overrides the equals method inherited from Object so that the method returns true if the input is a Date object (our Date, not the API Date) with the same month and day (the year may be different). Otherwise the method returns false.
-
It has input int day, int month, int year and initializes the Date object with the given inputs. You may assume that the inputs are all valid values.
-
-
The Stock class. The Stock class represents a stock. The Stock class will have a ticker symbol, a current price, a dividend rate, the date the dividend is paid, the number of shares owned, the cost basis of the stock, and the capital gains earned by the stock. The class should have the following methods:
-
getTickerSymbol: takes no input and returns a String that is the stock market ticker symbol. The ticker symbol will never change.
-
getCurrentPrice: takes no input and returns a double that is the current price for a share of the stock.
-
setCurrentPrice: takes a double as input and returns nothing. Changes the current price of a share of the stock.
-
getDividendRate: takes no input and returns a double that is the dividend rate for the stock.
-
setDividendRate: takes a double as input and returns nothing. Changes the dividend rate for the stock.
-
getDividendDate: takes no input and returns a Date that is the dividend date for the stock, or the method returns null if the stock does not pay dividends.
-
setDividendDate: takes a Date as input and returns nothing. Changes the dividend date for the stock. If the stock does not pay dividends, the dividend date should be null.
-
getNumberShares: takes no input and returns an int that is the number of shares owned in the stock.
-
getCostBasis: takes no input and returns a double that is the cost basis for the owned shares.
-
getCapitalGains: takes no input and returns a double that is the total capital gains earned so far.
-
payDividend: takes no input and returns a double. Returns the dividend rate multiplied by the number of shares.
-
buy: takes an int and a double as input and returns a double. The input values are the number of shares being purchased and the commission. Increases the number of shares owned in the stock by the number of shares purchased. Increases the cost basis for the stock by (number of shares purchased x the current price) + commission. Returns the (number of shares purchased x the current price) + commission.
-
sell: takes an int and a double as input and returns a double. The inputs are the number of shares being sold and the commission. If number of shares being sold is greater than the number currently owned, returns 0 and makes no other changes. Otherwise, the number of shares owned is decreased by number of shares sold. The cost basis is decreased by the ratio of number of shares sold to the number of shares originally owned. (For example, if you sell 1/3 of your shares, the cost basis should decrease by 1/3.) The capital gains is increased by the difference between (number of shares sold x the current price) - commission and the amount that the cost basis decreased. Returns (number of shares sold x the current price) - commission.
-
split: takes two int values as input and returns a double. The input values are the numerator and the denominator of the split fraction. If either input value is zero, nothing is done and 0 is returned. Otherwise, the number of owned shares changes by the ratio numerator / denominator. (For example, split(2,1) should double the number of shares while split(1,2) should result in one half the number of shares.) If the resulting number of shares contains a fractional share (not a whole number), then the fractional share must be sold so that the final number of shares is a whole number. This is exactly like selling shares normally, except that no commission is charged. The amount returned is the amount of money made from the sale (amount sold times price), and if there is no fractional share because the result of the split is a whole number, then 0 should be returned.
-
One has two inputs: a String (the ticker symbol) and a double (the current price).
-
One has four inputs: a String (the ticker symbol), a double (the current price), a Date (the dividend date), and a double (the dividend rate).
-
-
The Cash class. The Cash class represents an account that deals in money. The Cash class will have a balance, an interest rate, and a record of the amount of interest earned. The class should have the following methods.
-
getBalance: takes no input and returns a double that is the current balance in the account.
-
getInterestRate: takes no input and returns a double that is the interest rate.
-
setInterestRate: takes a double as input and returns nothing. Changes the interest rate.
-
transfer: takes a double as input and returns nothing. Reduces the current balance by the input amount.
-
getInterestEarned: takes no input and returns a double that is the amount of interest accrued this month.
-
processDay: takes no input and returns nothing. If the account balance is positive, multiplies the balance by the interest rate (divided by 365) and adds the amount to the current monthly interest.
-
processMonth: takes no input and returns nothing. Adds the current monthly interest to the balance and sets the current monthly interest to zero.
-
-
The Savings class. The Savings class is a Cash class that represents money deposited or earned by the customer. The Savings class has the same properties and behaviors of the Cash class plus the following two additional methods:
-
deposit: takes a double as input and returns nothing. Increases the balance by the input amount.
-
withdraw: takes a double as input and returns a boolean. If the input amount is less than or equal to the current balance, reduces the balance by the input amount and returns true. Otherwise returns false and makes no change to the balance.
-
-
The Loan class. The Loan class is a Cash class the represents money borrowed by the customer. The Loan class has all the same properties and behaviors of the Cash class in addition to the loan limit, the overdraft penalty as well as whether the loan is overdrafted. The class should have all the methods of Cash except the processDay and processMonth will need additional behavior as listed below and there will be four new getter/setter methods to correspond to the additional features of the Loan class:
-
getLoanLimit: takes no input and returns a double that is the loan limit.
-
setLoanLimit: takes a double as input and returns nothing. Changes the loan limit .
-
getOverdraftPenalty: takes no input and returns a double that is the overdraft penalty.
-
setOverdraftPenalty: takes a double as input and returns nothing. Changes the overdraft penalty.
- processDay: takes no input and returns nothing. This method should have the same behavior as in the Cash class plus if the current balance exceeds the loan limit, then the method should record that the loan is overdrafted for this month.
- processMonth: takes no input and returns nothing. This method should have the same behavior as in the Cash class plus if the loan was ever overdrafted since the last time processMonth was called, then the overdraft penalty should be added to the balance.
-
-
The Customer class. The Customer class represents a customer account. The Customer class will have a customer name (first and last), the customer's stock, the customer's savings, the customer's loan, the commission, and the date. The class should have the following methods.
-
getFirstName: takes no input and returns a String that is the first name associated with the account.
-
setFirstName: takes a String as input and returns nothing. Changes the first name associated with the account.
-
getLastName: takes no input and returns String the last name associated with the account.
-
setLastName: takes a String as input and returns nothing. Changes the last name associated with the account.
-
getStock: takes no input and returns a Stock that is the stock associated with this customer.
-
setStock: takes a Stock as input and returns nothing. Changes the stock instance associated with this customer.
-
getSavings: takes no input and returns a Savings that is the savings account associated with this customer. The savings account will not change.
-
getLoan: takes no input and returns a Loan that is the loan associated with this customer. The loan will not change.
-
getCommissionAmount: takes no input and returns a double that is the commission amount for the account.
-
setCommissionAmount: takes a double as input and returns nothing. Changes the commission amount for the account.
-
getDate: takes no input and returns a Date instance.
-
setDate: takes a Date as input and returns nothing. Changes the Date on the account.
-
currentValue: takes no input and returns a double that is the Savings balance plus the Stock number of shares times the Stock current price and subtracted by the Loan balance.
-
deposit: takes a double as input and returns nothing. Adds the input amount to the savings balance.
-
payLoan: takes a double as input and returns nothing. Reduces the loan balance by the input amount.
-
withdraw: takes a double as input and returns a boolean. If the input amount is less than or equal to the savings balance, reduces the savings balance by the input amount and returns true. Otherwise returns false and makes no change to the savings balance. (Hint: how much do you need to code?)
-
sellShares: takes a int and a String as input and returns nothing. The input is the number of shares being sold and the String is the ticker symbol of the stock that is being sold. If the customer's stock's ticker symbol matches that input to this method, then call the stock's sell method with the amount of shares input to this method and the customer's commission amount. The amount returned by the sell method should be added to the customer's savings.
-
buyShares: takes an int and a String as input and returns a boolean. The inputs are the number of shares to purchase and the ticker symbol of the stock being purchased. If the ticker symbol of the customer's stock matches the input ticker symbol and if the total value of the customer (from the currentValue) method is equal or larger than the amount it would take to purchase the stock ((the number of shares times the stock's price) plus the commission), the stock's buy method is called, the saving balance is reduced by the amount returned from the buy method, and the buyShares method returns true. On the other hand if either the input ticker is not the same as the customer's stock's ticker, or if the cost of the puchase is larger than the customer's current value, the method returns false and no other processing is done.
- incrementDate: takes no input and returns nothing. Calls the associated method of the Date class to increment the date. If the date now equals the stock's dividend date, call the payDividend method of the stock and deposit the amount returned into the savings. If the savings balance is negative, transfer that balance to the loan (increasing the balance of the loan), and set the savings balance to zero. Then call the processDay method of both the savings and loan instances. Finally, if the month changed as a result of the increment, call the processMonth method of the savings and loan instances.
-
Step by Step Solution
There are 3 Steps involved in it
Step: 1
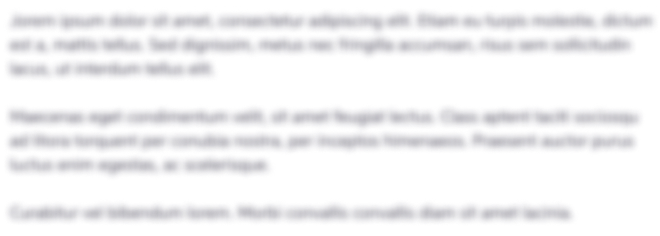
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started