Question
---IN JAVA-- Rewrite your Stack assignment using a Linked List instead of an array. You can use string as the object instead of Clothing. Here
---IN JAVA--
Rewrite your Stack assignment using a Linked List instead of an array. You can use string as the object instead of Clothing.
Here is the stack assignment.
package clothing;
class clothing{ private String size; private String color; public clothing(){ size = null; color = null; } public clothing(String a , String b){ size = a; color = b; } public void setSize(String a){ size = a; } public void setColor(String a){ color = a; } public String getSize(){ return size; } public String getColor(){ return color; } public String toString(){//output return "[Size=" + getSize()+ "," + "Color=" + getColor() + "]"; }
}
--next class--
package clothing;
class pants extends clothing{
public pants(){
super();
}
}
--next class--
package clothing;
class shirt extends clothing{
private String style;
public shirt(String a , String b){
super(a,b);
if (a.equals("S") || a.equals("M") || a.equals("L") || a.equals("XL")){
super.setSize(a);
}else{
super.setSize(null);
}
style = null;//didnt have a style so set to null
}
public void setSize(String a){
if (a.equals("S") || a.equals("M") || a.equals("L") || a.equals("XL")){
super.setSize(a);
}
else{
super.setSize(null);
}
}
public shirt(String a){
super(null,null);
style = a;
}
public void setStyle(String a){
style = a;
}
public String getStyle(){
return style;
}
public String toString(){
return "[Style=" + getStyle()+ "," +"Size=" + getSize()+ "," + "Color=" + getColor() + "]";
}
}
--Next Class--
package clothing;
class socks extends clothing{
private boolean pair;
public socks(String a , String b){
super(a,b);
pair = true;
}
public socks(String a , String b, boolean pr){
super(a,b);
pair = pr;
}
public void setPair(boolean a){
pair = a;
}
public boolean getPair(){
return pair;
}
public String toString(){
return "[Pair=" + getPair()+ "," +"Size=" + getSize()+ "," + "Color=" + getColor() + "]";
}
}
--Driver Class--
package clothing;
public class clothingTester {
public static void main(String[] args){
// Test super class only
clothing suit = new clothing();
clothing jersey = new clothing("medium", "blue");
System.out.println("Clothing 1:" + suit);
System.out.println("Clothing 2:" + jersey);
System.out.println();
// Test shirt class
clothing blueShirt = new shirt("medium", "blue"); // sets size to null since not S, M, L or XL
shirt pinkShirt = new shirt("Short Sleeves"); // shirt class has one field called style
pinkShirt.setColor("Pink");
pinkShirt.setSize("M");
System.out.println("BlueShirt " + blueShirt);
System.out.println("PinkShirt " + pinkShirt);
System.out.println();
// Test pants class
pants blackPants = new pants();
blackPants.setColor("Black");
blackPants.setSize("M");
System.out.println("BlackPants " + blackPants);
System.out.println();
// Test sock class
clothing purpleSocks = new socks("9-10", "Purple"); // socks has field pair, boolean default true in this constructor
socks greenSock = new socks("7-8", "Green" ,false);
System.out.println("PurpleSocks " + purpleSocks);
System.out.println("GreenSocks " + greenSock);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
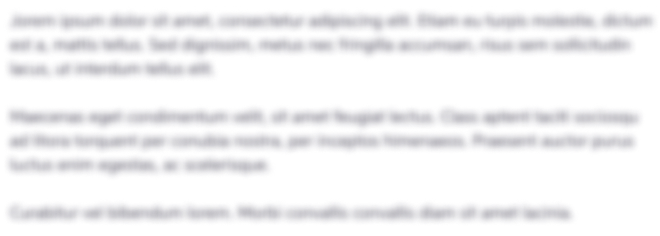
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started