Question
In java---- The DeckTester.java file, provides a basic set of Deck tests. Add additional code at the bottom of the main method to create a
In java---- The DeckTester.java file, provides a basic set of Deck tests. Add additional code at the bottom of the main method to create a standard deck of 52 cards and test the shuffle method in the Deck class. After testing the shuffle class, use the Deck toString method to see the cards after every shuffle.
Deck:
import java.util.List; import java.util.ArrayList;
/** * The Deck class represents a shuffled deck of cards. * It provides several operations including * initialize, shuffle, deal, and check if empty. */ public class Deck {
/** * cards contains all the cards in the deck. */ private List
/** * size is the number of not-yet-dealt cards. * Cards are dealt from the top (highest index) down. * The next card to be dealt is at size - 1. */ private int size;
/** * Creates a new Deck
instance.
* It pairs each element of ranks with each element of suits, * and produces one of the corresponding card. * @param ranks is an array containing all of the card ranks. * @param suits is an array containing all of the card suits. * @param values is an array containing all of the card point values. */ public Deck(String[] ranks, String[] suits, int[] values) { cards = new ArrayList
/** * Determines if this deck is empty (no undealt cards). * @return true if this deck is empty, false otherwise. */ public boolean isEmpty() { return size == 0; }
/** * Accesses the number of undealt cards in this deck. * @return the number of undealt cards in this deck. */ public int size() { return size; }
/** * Randomly permute the given collection of cards * and reset the size to represent the entire deck. */ public void shuffle() { for( int x = size - 1; x >= 0; x-- ) { int y = (int)(Math.random() * x); Card k = cards.get(y); cards.set(y, cards.get(x)); cards.set(x, k); } }
/** * Deals a card from this deck. * @return the card just dealt, or null if all the cards have been * previously dealt. */ public Card deal() {
if (isEmpty()) {
return null;
} else {
size--;
Card c = cards.get(size);
return c;
}
} /** * Generates and returns a string representation of this deck. * @return a string representation of this deck. */ @Override public String toString() { String rtn = "size = " + size + " Undealt cards: ";
for (int k = size - 1; k >= 0; k--) { rtn = rtn + cards.get(k); if (k != 0) { rtn = rtn + ", "; } if ((size - k) % 2 == 0) { // Insert carriage returns so entire deck is visible on console. rtn = rtn + " "; } }
rtn = rtn + " Dealt cards: "; for (int k = cards.size() - 1; k >= size; k--) { rtn = rtn + cards.get(k); if (k != size) { rtn = rtn + ", "; } if ((k - cards.size()) % 2 == 0) { // Insert carriage returns so entire deck is visible on console. rtn = rtn + " "; } }
rtn = rtn + " "; return rtn; } }
DeckTester:
/** * This is a class that tests the Deck class. */ public class DeckTester {
/** * The main method in this class checks the Deck operations for consistency. * @param args is not used. */ public static void main(String[] args) { String[] ranks = {"jack", "queen", "king"}; String[] suits = {"blue", "red"}; int[] pointValues = {11, 12, 13}; Deck d = new Deck(ranks, suits, pointValues);
System.out.println("**** Original Deck Methods ****"); System.out.println(" toString: " + d.toString()); System.out.println(" isEmpty: " + d.isEmpty()); System.out.println(" size: " + d.size()); System.out.println(); System.out.println();
System.out.println("**** Deal a Card ****"); System.out.println(" deal: " + d.deal()); System.out.println(); System.out.println();
System.out.println("**** Deck Methods After 1 Card Dealt ****"); System.out.println(" toString: " + d.toString()); System.out.println(" isEmpty: " + d.isEmpty()); System.out.println(" size: " + d.size()); System.out.println(); System.out.println();
System.out.println("**** Deal Remaining 5 Cards ****"); for (int i = 0; i < 5; i++) { System.out.println(" deal: " + d.deal()); } System.out.println(); System.out.println();
System.out.println("**** Deck Methods After All Cards Dealt ****"); System.out.println(" toString: " + d.toString()); System.out.println(" isEmpty: " + d.isEmpty()); System.out.println(" size: " + d.size()); System.out.println(); System.out.println();
System.out.println("**** Deal a Card From Empty Deck ****"); System.out.println(" deal: " + d.deal()); System.out.println(); System.out.println();
/* *** TO BE COMPLETED *** */ } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
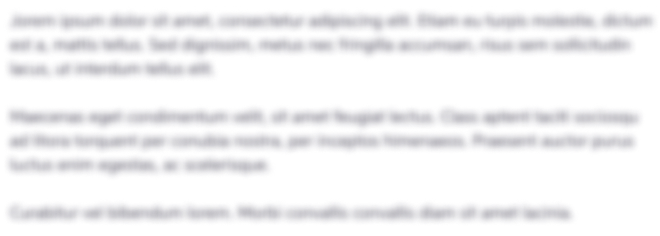
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started