Question
*** in java *** worksheet worksheet1.java // Worksheet #1: Stacks import java.io.File; import java.io.IOException; import java.util.ArrayList; import java.util.Scanner; public class Worksheet1_Spring2020 { public static void
*** in java ***
worksheet
worksheet1.java
// Worksheet #1: Stacks
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Scanner;
public class Worksheet1_Spring2020 {
public static void main(String[] args) throws IOException {
// Name of file to read from
final String BOOKS_FILE_NAME = "Worksheet1Books.txt";
// Setup a file reference variable to refer to text file
File booksFileName = new File(BOOKS_FILE_NAME);
// First value in file is the number of books in the file
Scanner booksFile = new Scanner (booksFileName);
int numBooksInFile = booksFile.nextInt();
//****************************************
// Worksheet1 Question #2:
// Write code to create a stack of books
//*****************************************
// ADD YOUR CODE HERE
// Create book objects from the details provided in the file
for(int i = 0; i
// Read information from file and create a book
String name = (booksFile.nextLine()).trim();
BookW1 book = new BookW1(name);
// Print the books's details
System.out.println(book.print());
//****************************************
// Worksheet1 Question #3:
// Write code to add the book to the stack
//****************************************
// ADD YOUR CODE HERE
} // for each book
//****************************************
// Worksheet1 Question #4
// Write code to print the name of each book on the stack.
// Use a while loop to perform this task.
//****************************************
// ADD YOUR CODE HERE
System.out.println();
System.out.println();
//****************************************
// Worksheet1 Question #5
// Write code to examine the top element on the stack
//****************************************
// ADD YOUR CODE HERE
System.out.println();
System.out.println();
booksFile.close();
} // main
} // Worksheet1
// Class representing the generic stack - named it GenericStackSp20 to avoid name
clashing issues
class GenericStackSp20
private ArrayList
ArrayList
public GenericStackSp20() {
list = new ArrayList();
}
public boolean isEmpty (){
return list.isEmpty();
}
public int getSize(){
return list.size();
}
// Returns the top element on the top without removing it
// Since using array list to store elements, this means getting the last
element in the array
public E peek(){
return list.get(getSize() - 1);
}
// Removed and returns the top element on the stack
// Since using array list to store elements, this means removing the last
element in the array
public E pop(){
E value = list.get(getSize()-1);
list.remove(getSize() - 1);
return value;
}
// Place a new element on the top of the stack
// Since using array list to store elements, this means adding the element to
the array
public void push(E value){
list.add(value);
}
} // GenericStackSp20
class BookW1 {
private String name;
public BookW1 (String name) {
this.name = name;
}
public String getName() {
return name;
}
// Create and returns a string containing book details
public String print() {
return String.format("%s", name);
}
} // BookW1
worksheet2.java
// Worksheet #2: Nested Objects
import java.util.LinkedList;
import java.util.Queue;
public class Worksheet2_Spring2020 {
public static void main(String[] args) {
// Create some books to be added to the queue
BookW2 javaBook = new BookW2("Java");
BookW2 cBook = new BookW2("C Programming");
BookW2 pythonBook = new BookW2("Python");
//*******************************************
// Worksheet2 Question #1
// Queue in main - no nesting
// Practice creating a queue here and adding the three books
// Name your queue - queueNotNested
//*******************************************
// ADD CODE HERE
// Test your non-nested queue by displaying the values in the queue
System.out.println("Values in a queue that is not nested in a class");
int queueLength = queueNotNested.size();
for (int i = 0; i
System.out.println("aQueue[" + i + "] = " +
queueNotNested.remove().getName());
}
//********************************
// Worksheet2 Question #2
// See BookQueue Class below
//********************************
//********************************
// Worksheet2 Question #3a
// Use this code to test your BookQueue class
// Queue is now nested in a class
// Create an object of type BookQueue and add the three books
//********************************
// ADD CODE HERE
BookQueue aQueue = new BookQueue();
//********************************
// Worksheet2 Question #3b
// Test your nested queue by displaying the names of the books in the
queue
//********************************
// ADD CODE HERE
System.out.println();
System.out.println("Values in a queue nested in a class");
} // main
} // Worksheet2
//********************************
//Worksheet2 Question #2
//Write the code for each method
//********************************
class BookQueue {
private Queue
public int size() {
// ADD CODE HERE
}
public void offer(BookW2 book) {
// ADD CODE HERE
}
public BookW2 remove() {
// ADD CODE HERE
}
} // BookQueue
class BookW2 {
private String name;
public BookW2 (String name) {
this.name = name;
}
public String getName() {
return name;
}
// Create and returns a string containing book details
public String print() {
return String.format("%s", name);
}
} // BookW1
just need a start on this. i am new to coding and need help
Worksheet #1: Stacks (15 pts) Download and import the file Worksheet1.java. Use the file to answer the following questions. Please write your answers in the worksheet. Add code to the file to see if it works but do not turn in the code. 1) Notice Worksheet1.java contains the generic stack class from assignment 5 and a Book class. If we wanted to create a stack of books, give a short explanation of what changes, if any, are needed to the generic class to create a stack of books. Give a short reason why. (2pts) 2) Note that the file contains a Book class. What code is needed to create a stack of books? (2 pts) a. In main, find the comment "Worksheetl Question #2" b. At this point, what code is needed to create a stack of books called bookStack? Write code here: 3) What code is needed to add new book object to the bookStack? (2 pts) c. In main, find the comment "Worksheetl Question #3" d. At this point, what code is needed to add a book to the stack? Write that code here: 4) What code is needed to print the name of each book in the bookStack? Use a while loop. (6 pts) e. In main, find the comment Worksheetl Question #4 f. At this point, write a while loop to print the name of each book. Write that code here: 5) What code is needed to examine the top element on the bookStack? (3 pts) g. In main, find the comment Worksheetl Question #5" h. At this point, add one line of code to display the book on top of the stack. Write that code here: i. What error occurs when you add the line in 5h and run the file? Why does this occur? Worksheet #2: Nested Objects (12 pts) Download and import the file Worksheet2.java. Use the file to answer the following questions. 1) Let's create a queue in main. This means, the queue is not nested inside a class. In main, find the comment Worksheet2 Question #I a. At this point, write the declaration for a queue of books and place the books that have been created for you into this queue using the offer method. Write that code here: (2 pt) 2) Next, let's move the queue inside a class called Book Queue. Complete the Book Queue class below by writing on the worksheet the code required for each method. Find Worksheet2 Question #2. (6 pts) class Book Queue private QueueStep by Step Solution
There are 3 Steps involved in it
Step: 1
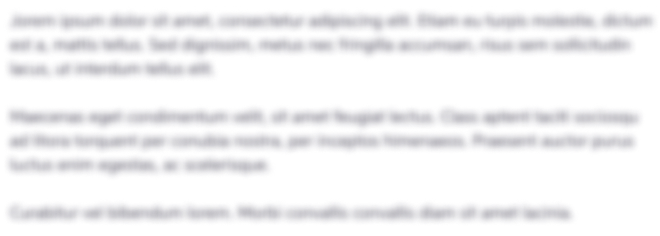
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started