Question
In Java, Write an exception class named InvalidTestScore. Modify the TestScores class you wrote in Programming Challenge 1 so that it throws an InvalidTestScore exception
In Java, Write an exception class named InvalidTestScore. Modify the TestScores class you wrote in Programming Challenge 1 so that it throws an InvalidTestScore exception if any of the test scores in the array are invalid.
Here's the code I need help in being modified to fit this question:
TestScores.java
public class TestScores
{
private int[]score;
private int avg;
public TestScores(int[] testScores) throws IllegalArgumentException
{
avg = validScore(testScores);
score = testScores;
}
private int validScore(int[] testScores)
{
for (int i = 0; i < testScores.length; i++)
{
if(testScores[i] < 0 || testScores[i] > 100)
{
throw new IllegalArgumentException
("Test score" + (i+1) + "must be between 0-100.");
}
avg += testScores[i];
}
return (avg / testScores.length);
}
public int getAvg()
{
return avg;
}
}
TestScoreDemo.java
import java.util.Scanner;
public class TestScoreDemo
{
public static void main(String[] args)
{
int[] scores = getArray(readLine("Enter # of tests: "));
try
{
TestScores t = new TestScores(scores);
System.out.println(" Average Test Score: " + t.getAvg());
}
catch (IllegalArgumentException i)
{
System.out.println("Invalid test score range." + i.getMessage());
}
}
public static int[] getArray(int woosah)
{
int[] scores = new int[woosah];
for (int i = 0; i < scores.length; i++)
{
scores[i] = readLine(" Enter score for Test " + (i+1) +": ");
}
return scores;
}
public static int readLine(String line)
{
Scanner key = new Scanner (System.in);
System.out.println(line);
return key.nextInt();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
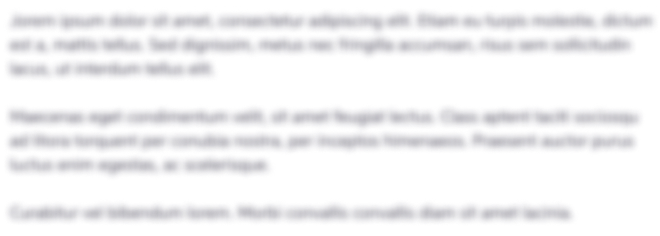
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started