Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In java you will be creating a simple expression calculator in Calculator.java. To do so you need two stacks: one to contain operators and the
In java you will be creating a simple expression calculator in Calculator.java. To do so you need two stacks: one to contain operators and the other to contain operands (numbers). When an operand is encountered it is pushed onto the operand stack. When an operator is encountered, it is processed as described in the infix to postfix algorithms. When an operator is popped off the operator stack, it is processed as described in the postfix evaluation algorithm: the top two operands are popped off the operand stack, the operation is performed, and the result is pushed back onto the operand stack. a. Implement numberStackToString using the hints provided. Enter arithmetic expression or cancel. 2+3*4 numberStack: 2.0 operatorStack: numberStack: 2.0 operatorStack: numberStack: 2.0 3.0 operatorStack: numberStack: 2.0 3.0 operatorStack: numberStack: 2.0 3.0 4.0 operatorStack: numberStack: 2.0 3.0 4.0 operatorStack: numberStack: 2.0 3.0 4.0 operatorStack: 2+3*4 = 4.0 b. Ditto operatorStackToString. Enter arithmetic expression or cancel. 2+3*4 numberStack: 2.0 operatorStack: numberStack: 2.0 operatorStack: + numberStack: 2.0 3.0 operatorStack: + numberStack: 2.0 3.0 operatorStack: + * numberStack: 2.0 3.0 4.0 operatorStack: + * numberStack: 2.0 3.0 4.0 operatorStack: + numberStack: 2.0 3.0 4.0 operatorStack: 2+3*4 = 4.0 c. Finish evaluateOperator by adding cases for -,*,/, and ^. Use Math.pow for ^. d. Implement evaluateTopOperator. It should pop an operator off the operator stack and two number off the number stack. Then it should call evaluateOperator and push the result on the number stack. Call displayStacks() before you return. Enter arithmetic expression or cancel. 4-2 numberStack: 4.0 operatorStack: numberStack: 4.0 operatorStack: - numberStack: 4.0 2.0 operatorStack: - numberStack: -2.0 operatorStack: 4-2 = -2.0 e. Did you get -2 instead of 2? If so, then fix evaluateTopOperator! Remember, things come out of a stack in the reverse order that they go in. Enter arithmetic expression or cancel. 4-2 numberStack: 4.0 operatorStack: numberStack: 4.0 operatorStack: - numberStack: 4.0 2.0 operatorStack: - numberStack: 2.0 operatorStack: 4-2 = 2.0 f. Try 2*3+4. You should bet 14 instead of 10 because it doesn't understand that * has higher "precedence" than +. You will fix this for the homework. Here, I show where it is in the expression for each step by writing out the expression and putting an arrow below what is being read. So 2*3+4 ^ means we are reading the 2. 2*3+4 ^ numberStack: 2.0 operatorStack: 2*3+4 ^ numberStack: 2.0 operatorStack: * 2*3+4 ^ numberStack: 2.0 3.0 operatorStack: * 2*3+4 ^ numberStack: 2.0 3.0 operatorStack: * + 2*3+4 ^ numberStack: 2.0 3.0 4.0 operatorStack: * + 2*3+4 ^ numberStack: 2.0 7.0 operatorStack: * 2*3+4 ^ numberStack: 14.0 operatorStack: 2*3+4 = 14.0
package prog05; import java.util.Stack; import java.util.Scanner; import javax.swing.SwingUtilities; import prog02.UserInterface; import prog02.GUI; import prog02.ConsoleUI; public class Calculator { static final String OPERATORS = "()+-*/u^"; static final int[] PRECEDENCE = { -1, -1, 1, 1, 2, 2, 3, 4 }; StackoperatorStack = new Stack (); Stack numberStack = new Stack (); UserInterface ui = new GUI("Calculator"); Calculator (UserInterface ui) { this.ui = ui; } void emptyStacks () { while (!numberStack.empty()) numberStack.pop(); while (!operatorStack.empty()) operatorStack.pop(); } String numberStackToString () { String s = "numberStack: "; Stack helperStack = new Stack (); // EXERCISE // Put every element of numberStack into helperStack // You will need to use a loop. What kind? // What condition? When can you stop moving elements out of numberStack? // What method do you use to take an element out of numberStack? // What method do you use to put that element into helperStack. // Now put the back, but also add each one to s: // s = s + " " + number; return s; } String operatorStackToString () { String s = "operatorStack: "; // EXERCISE return s; } void displayStacks () { ui.sendMessage(numberStackToString() + " " + operatorStackToString()); } void doNumber (double x) { numberStack.push(x); displayStacks(); } void doOperator (char op) { processOperator(op); displayStacks(); } double doEquals () { while (!operatorStack.empty()) evaluateTopOperator(); return numberStack.pop(); } double evaluateOperator (double a, char op, double b) { switch (op) { case '+': return a + b; // EXERCISE } System.out.println("Unknown operator " + op); return 0; } void evaluateTopOperator () { char op = operatorStack.pop(); // EXERCISE displayStacks(); } void processOperator (char op) { operatorStack.push(op); } static boolean checkTokens (UserInterface ui, Object[] tokens) { for (Object token : tokens) if (token instanceof Character && OPERATORS.indexOf((Character) token) == -1) { ui.sendMessage(token + " is not a valid operator."); return false; } return true; } static void processExpressions (UserInterface ui, Calculator calculator) { while (true) { String line = ui.getInfo("Enter arithmetic expression or cancel."); if (line == null) return; Object[] tokens = Tokenizer.tokenize(line); if (!checkTokens(ui, tokens)) continue; try { for (Object token : tokens) if (token instanceof Double) calculator.doNumber((Double) token); else calculator.doOperator((Character) token); double result = calculator.doEquals(); ui.sendMessage(line + " = " + result); } catch (Exception e) { ui.sendMessage("Bad expression."); calculator.emptyStacks(); } } } public static void main (String[] args) { UserInterface ui = new ConsoleUI(); Calculator calculator = new Calculator(ui); processExpressions(ui, calculator); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
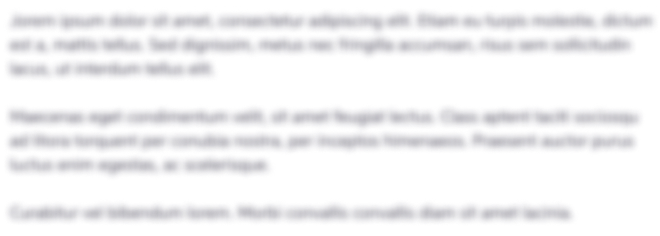
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started