Question
IN MARS PLEASE Description You are responsible for writing a string handling library using MIPS. You are to implement the functions listed below. All parameters
IN MARS PLEASE
Description You are responsible for writing a string handling library using MIPS. You are to implement the functions listed below. All parameters are listed in order, and should correspond to the registers $a0,...,$a3,$t0,...,$t9. This assignment may require you to create arrays at runtime. In order to do so, you should allocate them on the heap with a syscall:
For the full example, see heap allocation on the course website.
Testing A driver has been provided for you on the course website. To use it, you may simply start writing your functions in the file given. If your code is correct, you should recieve the following output:
Driver With A Correct Implementation Testing length, suffix, and memchr: 50 49 24 -1
Testing length, suffix, memchr, and strncmp: -1 1 0 -1
Consult the driver comments for a detailed explanation on what the numbers mean.
1 Submitting Your submission should make all function labels global.
For example, this means that your memchr function should be written as so: Global Labels . t e x t . . . . g l o b l memchr memchr : . . . In addition, your solution should only contain code that you wrote. In other words, before submitting your code make sure you have done the following:
1. All of your functions labels are global
2. All code in the original driver has been removed (including the data segment). Your code should be submitted to the departmental dropbox with the filename HW1.asm Interface strlen Finds the length of a string str.
Parameters $a0 - (addr) str Return (int) The length of the string suffix Finds (or constructs) a string that is the last len - n characters of the input string str. Parameters $a0 - (addr) str $a1 - (int) n Return (addr) The address of the suffix memchr Searches for the first occurrence of a character c in the first n bytes of the string pointed to, by the argument str.
Parameters $a0 - (addr) s $a1 - (byte) c $a2 - (int) n Return (int) 2 The index of the first occurence, or -1 if not found. strncmp Compares the first n bytes of the strings pointed to, by str0 to the string pointed to by str1. Note: Strings can be compared by the ASCII values of their constituent characters.
Parameters $a0 - (addr) str0 $a1 - (addr) str1 $a2 - (int) n Return (int) 0 if the strings are equal in the first n bytes (by comparison, not address!) A negative int if str0 < str1 in the first n bytes A positive int if str0 > str1 in the first n bytes
DRIVER :
# HW1 Driver # 2018 Daniel Pade # All of your functions should be placed between the comments below .data pangram: .asciiz "Cozy lummox gives smart squid who asks for job pen" less: .asciiz "ASCII arranges characters roughly alphabetically." more: .asciiz "ascii arranges characters roughly alphabetically." # Explanatory text test_suite_1: .asciiz "Testing `length', `suffix', and `memchr':" test_suite_2: .asciiz "Testing `length', `suffix', `memchr', and `strncmp':" .text j main #------------------ PUT YOUR CODE HERE --------------------------- length: jr $ra suffix: jr $ra memchr: jr $ra strncmp: jr $ra #------------------ DON'T MODIFY BELOW --------------------------- .globl main main: la $s0 pangram la $s1 less la $s2 more la $a0 test_suite_1 ori $v0 $0 4 syscall jal endl # Find the length of `pangram' -> print 50 jal tab or $a0 $0 $s0 jal length or $s3 $0 $v0 # We need the length for memchr or $a0 $0 $v0 ori $v0 $0 1 syscall jal endl # Search for `n' in all of pangram -> print 49 jal tab or $a0 $0 $s0 ori $a1 $0 0x6E or $a2 $0 $s3 jal memchr or $a0 $0 $v0 ori $v0 $0 1 syscall jal endl # Search for `n' in the last half of pangram -> print 24 jal tab srl $s3 $s3 1 or $a0 $0 $s0 or $a1 $0 $s3 jal suffix or $a0 $0 $v0 ori $a1 $0 0x6E or $a2 $0 $s3 jal memchr or $a0 $0 $v0 ori $v0 $0 1 syscall jal endl # Search for `n' in the first half of pangram -> print -1 jal tab or $a0 $0 $s0 ori $a1 $0 0x6E or $a2 $0 $s3 jal memchr or $a0 $0 $v0 ori $v0 $0 1 syscall jal endl jal endl la $a0 test_suite_2 ori $v0 $0 4 syscall jal endl # Verify less < more -> print -1 jal tab or $a0 $0 $s1 jal length or $s3 $0 $v0 # Store the length of the strings for later or $a0 $0 $s1 or $a1 $0 $s2 or $a2 $0 $s3 jal strncmp or $a0 $0 $v0 ori $v0 $0 1 syscall jal endl # Verify more > less -> print 1 jal tab jal space or $a0 $0 $s2 or $a1 $0 $s1 or $a2 $0 $s3 jal strncmp or $a0 $0 $v0 ori $v0 $0 1 syscall jal endl # Find the first occurrence of ' ' in less or $a0 $0 $s1 ori $a1 $0 0x20 or $a2 $0 $s3 jal memchr addi $s4 $v0 1 # Save the index of ' ' (+1) # Get the suffixes of less & more, along with the length or $a0 $0 $s1 or $a1 $0 $s4 jal suffix or $s1 $0 $v0 or $a0 $0 $s2 or $a1 $0 $s4 jal suffix or $s2 $0 $v0 or $a0 $0 $s1 jal length or $s3 $0 $v0 # Verify the suffixes are equal -> print 0 jal tab jal space or $a0 $0 $s1 or $a1 $0 $s2 or $a2 $0 $s3 jal strncmp or $a0 $0 $v0 ori $v0 $0 1 syscall jal endl # Verify smaller strings are less than larger strings -> print -1 jal tab addi $s4 $s3 -1 add $s5 $s1 $s4 sb $0 0($s5) or $a0 $0 $s1 or $a1 $0 $s2 or $a2 $0 $s3 jal strncmp or $a0 $0 $v0 ori $v0 $0 1 syscall ori $v0 $0 10 syscall ## Print a newline. # This can be called freely without concern of lost registers. .globl endl endl: addi $sp $sp -4 sw $a0 0($sp) addi $sp $sp -4 sw $v0 0($sp) ori $a0 $0 0xA ori $v0 $0 11 syscall lw $v0 0($sp) addi $sp $sp 4 lw $a0 0($sp) addi $sp $sp 4 jr $ra ## Print a tab # This can be called freely without concern of lost registers. .globl tab tab: addi $sp $sp -4 sw $a0 0($sp) addi $sp $sp -4 sw $v0 0($sp) ori $a0 $0 0x9 ori $v0 $0 11 syscall lw $v0 0($sp) addi $sp $sp 4 lw $a0 0($sp) addi $sp $sp 4 jr $ra ## Print a space # This can be called freely without concern of lost registers. .globl space space: addi $sp $sp -4 sw $a0 0($sp) addi $sp $sp -4 sw $v0 0($sp) ori $a0 $0 0x20 ori $v0 $0 11 syscall lw $v0 0($sp) addi $sp $sp 4 lw $a0 0($sp) addi $sp $sp 4 jr $ra
Step by Step Solution
There are 3 Steps involved in it
Step: 1
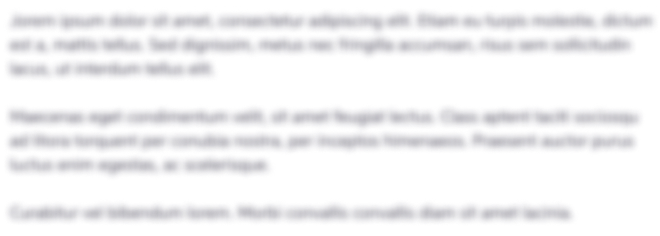
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started