Question
In Part A, you will create a type named World to represent the world for the program. You will eventually put the World type and
In Part A, you will create a type named World to represent the world for the program. You will eventually put the World type and its associated constants and functions in its own module, but for now put all the code in a file named BigMain.cpp
By the end of Part A, your World type will have functions with the following prototypes:
void worldLoadAll (World world, string game_name);
void worldLoadNodes (World world, string filename);
void worldLoadDescriptions (World world, string filename);
void worldDebugPrint (const World world);
void worldFindStart (const World world, unsigned int& row, unsigned int& column);
bool worldIsValid (const World world, unsigned int row, unsigned int column);
void worldPrintDescription (const World world, unsigned int row, unsigned int column);
bool worldCanGoNorth (const World world, unsigned int row, unsigned int column);
bool worldCanGoSouth (const World world, unsigned int row, unsigned int column);
bool worldCanGoEast (const World world, unsigned int row, unsigned int column);
bool worldCanGoWest (const World world, unsigned int row, unsigned int column);
bool worldIsDeath (const World world, unsigned int row, unsigned int column);
bool worldIsVictory (const World world, unsigned int row, unsigned int column);
Perform the following steps:
Use #include to include various libraries. Remember to put using namespace std;
Define ROW_COUNT, COLUMN_COUNT, INACCESSIBLE, DEATH, START, and VICTORY as constants with the values 6, 9, 0, 1, 2, and 3, respectively. Use a typedef command to define the World type to be a 2D array of unsigned ints of dimensions ROW_COUNT by COLUMN_COUNT.
Define DESCRIPTION_COUNT as a constant with the value 24.
Copy in the function prototypes shown above.
Create a global array of DESCRIPTION_COUNT strings.
Add a function named worldLoadNodes that takes a World and string as parameters. The string represents the file name for the world nodes. You should first open this file for reading. Then load (i.e., read) the first ROW_COUNT * COLUMN_COUNT integers from the file into the World array. At the end, close the file. If the file cannot be opened, print an error message. Hereafter, you should always print an error message when a file cannot be opened.
Hint: Start by copying the prototype for the function and in the copy, replace the semi-colon with a pair of braces { }.
Hint: Read in the world nodes using formatted I/O (>> notation).
Hint: For debugging, write a simple main function that declares a variable named world of the World type and then calls the worldLoadNodes function with this variable as the first argument and "blizzard_grid.txt" as the second argument.
Hint: If you are using Visual Studio, the program window will usually close as soon as the program ends. To prevent this, print a message saying "Press [ENTER] to continue... " at the end of main and then read in a line of input with getline.
Hint: In the main function, display the values in the my_world variable. Since the my_world variable is actually a 2D array, use two nested for-loops with cout statements inside. Printing a tab ('\t') after every number (in the inner loop) makes things neater, as does printing newline (endl) after every line (in the outer loop). Later you can move this display code to the worldDebugPrint function.
Add a function named worldLoadDescriptions that takes a World and string as parameters. The string represents the file name for the descriptions of the world nodes. Open the file and read the number of descriptions in the file. If it is not equal to DESCRIPTION_COUNT, print an error message and terminate the program. Otherwise, read the descriptions from the file into the global description array and close the file.
Hint: The World parameter is not actually used at this time. It is needed in a later assignment.
Hint: After reading the number of descriptions using >>, use a .ignore to ignore the remainder of the line of input. Start the description number at zero (0). Then read the other lines from the description file one at a time using getline. If the line you just read is not blank, you should add it to the current description in the array and then add a newline character (' ') as well. Otherwise, if you have not read enough descriptions yet, advance to the next description by incrementing the description number. Otherwise, you are done and should close the file and return from the function.
Hint: For debugging, add a call to the worldLoadDescriptions function to your main function. Make sure to indicate the filename as blizzard_text.txt.
Hint: For debugging, display each description number and description as you are reading the descriptions. Later you can move this code to the worldDebugPrint function.
Comment: This function is perhaps the hardest part of Assignment 1!
Add a function named worldLoadAll that takes a World and string as parameters. The string represents the world name. Use the world name, such as "blizzard", to calculate the names of the node data file, such as "blizzard_grid.txt", and the text data file, such as "blizzard_text.txt". Use these filenames to call the worldLoadNodes and worldLoadDescriptions functions.
Hint: For debugging, change the main program to call the worldLoadAll function instead of the worldLoadNodes and worldLoadDescriptions functions.
For the remainder of Part A, it is assumed that you will add tests to the main function for each new function.
Add a function named worldDebugPrint that takes a World as a parameter. It should print out all the node values in the world in a grid, separated by tabs. Do not print a tab after the last node in a row.
Add a function named worldIsValid that takes a World, a row, and a column as parameters. The row and column represent a location, and the function should return a bool indicating whether that location is a valid world location. Valid world locations have a row value strictly less that ROW_COUNT and a column value strictly less than COLUMN_COUNT.
Hint: For debugging, put in quite a few tests of the worldIsValid function into the main function and print out the results of each. Example: cout << "worldIsValid with (0, 10) = " << worldIsValid(my_world, 0, 10) << endl; What happens if you pass it a negative number? If it runs, print out the value of row and column inside the function to understand why.
Add a function named worldPrintDescription that takes a World, a row, and a column as parameters. The function should determine the description number for that node and then print that description. When printing the descriptions, use an array lookup instead of many if statements.
Example: Suppose you wanted to print the node with row 1 and column 2. (This is the second row and third column because the array is indexed from 0.) The description number for that node is 7, so the program would print:
You are on a mountain slope, lost in the raging blizzard. Everything is white.
Add a set of four functions, named worldCanGoNorth, worldCanGoSouth, worldCanGoEast, and worldCanGoWest. Each of these functions should take a World and a row and column, representing a location, as parameters and return a bool indicating whether the player can move in the indicated direction from that node. If the move would take the player out of the array (you can check with the worldIsValid function) or to a node with a value of INACCESSIBLE, the function should return false. Otherwise, the player can move in that direction and the function should return true.
Add a set of two functions, named worldIsDeath and worldIsVictory. Each of these functions should take a World and a row and column, representing a location, as parameters and return a bool indicating whether that node is of the indicated type.
Add a function named worldFindStartNode. The function should takes a World and two references to unsigned ints, representing a row and a column, as parameters. The function should search the World for a node with the value START and set the row and column parameters to the location of the START node. You can return from the function as soon as you find a START node.
Hint: You can test the function by adding the following lines to the main function: unsigned int start_row, start_column;
worldFindStart(my_world, start_row, start_column);
cout << "worldFindStart: row = "
<< start_row
<< ", column = "
<< start_column
<< endl;
I need C++ code, any help would be wonderful
Step by Step Solution
There are 3 Steps involved in it
Step: 1
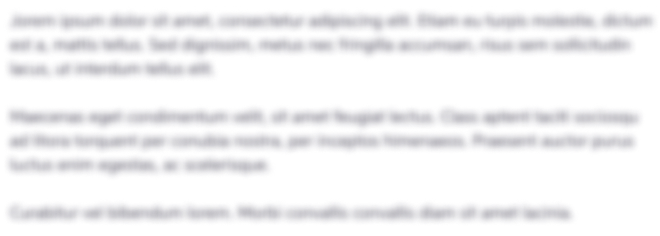
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started