Question
IN PYTHON HOW WOULD I COMPLETE THE FOLLOWING: Goals At the completion of this assignment, you should: have gained additional experience working with lists have
IN PYTHON HOW WOULD I COMPLETE THE FOLLOWING:
Goals
At the completion of this assignment, you should:
- have gained additional experience working with lists
- have gained additional experience working with input, strip() function and while loops
- have gained additional experience working with conditionals, logical and Boolean operators, and break
- have gained additional experience using functions
- have gained experience using string isdigit() function
- have gained additional experience creating more complex applications
Getting Started
For this assignment you will create a simple list manager program. Your list manager program will support the following use cases:
- Add data to a list
- Delete individual list items
- Clear the entire list
- Output available commands
The assignment contains the required functions, and a general outline to follow to implement the list manager program. As usual you are encouraged to follow the incremental development steps, including incremental testing.
Assignment
Part I: Required Functions
Create a file called cis122-assign07-list-manager.py for your list manager program.
Below is a list of required functions, where t is a list parameter. You should create all of these functions in your code file with a single statement pass (see the Python online documentation for pass (Links to an external site.), course text, or course lecture notes, if you forgot the purpose of pass).
- cmd_help()
- cmd_add(t)
- cmd_delete(t)
- cmd_list(t)
- cmd_clear(t)
- get_max_list_item_size(t)
You will also need to use your padding functions from Assignment 6. Copy all three functions into your code file.
- pad_string()
- pad_left()
- pad_right()
Part II: List variable
You will require a list variable to hold your list items. The list item should be declared outside of any function, and initially be empty.
Part III: Primary Input Loop
You will require a primary input loop to prompt for list commands. The loop must only handle the commands, and then call the appropriate function to handle the command. You must use the strip() function to remove leading and trailing white space. If the Enter key is pressed with no input, exit the program.
The prompt must be: "Enter a command (? for help): ".
Part IV: Help Command
Your list manager must display help.
- If the ? is entered as a command, display a list of commands, and a command description.
- The commands must be contained within a list.
- The command descriptions must be contained within a separate list.
- These two lists must contain the same number of items.
- Use the get_max_list_item_size() function to find the maximum length of all of the commands.
- Use a for loop to iterate through the loops to display the help listing.
- Use the pad_right() function and the maximum command length to format the command, with a space between the command and the command description of five (5) spaces.
- Add "Empty to exit" as a final help tip.
See the sample output below.
Part V: Add Command
Your list manager program must support adding data to the list.
- The add command will prompt the user for data to add until the Enter key is pressed with no input.
- After each data is entered, display a notification that data was added, and a count of the total number of list items.
See the sample output below.
Part VI: List Command
The list command will display the total number of items in the list, and each item in the list.
See the image above in Part V and the add command for sample output.
Part VII: Clear Command
The clear command will clear (delete) all items in the list, and specify how many items were removed and that the list is now empty.
You may use the list clear() function to empty the list.
See the sample output below.
Part VIII: Delete Command
The delete command will remove items from the list. Your list manager must support the shortcut del command as well.
- The delete command will display all items with the index number next to each item.
- Use the pad_right() function to format the index column, with a space of two (2) blank spaces between the index and the list item value.
- The delete command will continuously prompt for data to delete until either all data is deleted, or the Enter key is pressed with no input.
- The delete command must validate the entered index is a valid integer, and the value is in the range appropriate for the number of items in the list.
- Use the strip() and string isdigit() (Links to an external site.) function to test if the entered index is a valid integer.
- Output appropriate error messages if incorrect data is entered.
See the sample output below.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
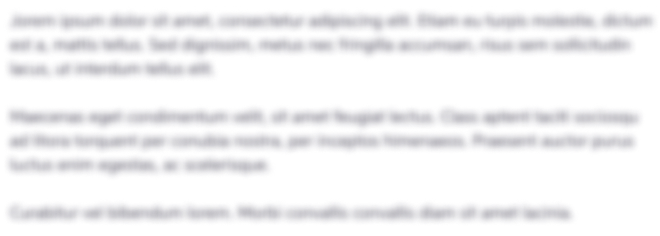
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started