Question
in the file TicTacToe.java is a program that will implement a basic game of Tic-Tic-Toe that will be played by a human player and the
in the file TicTacToe.java is a program that will implement a basic game of Tic-Tic-Toe that will be played by a human player and the computer with basic AI implemented. There will be a total of six methods, three of which are already written for you,
including main() . The three methods you will need to finish are: Method Purpose initBoard() This method simply sets all elements in the array of char s to a space ' ' .
movePlayer() This method implements the logic to let the human player pick a valid move on the board and then record the move.
moveAI() This method implements basic random AI for the computer to make a legal move on the board.
The three methods that are already written for you are:
Method Purpose main() The main() method that implements the game-playing logic.
printBoard() This method prints the Tic-Tac-Toe board to the screen.
checkWinner() This method will check the current board and return one of four char values depending on if a winner has been determined: X if the human player (X's) wins O if the computer player (O's) wins T if the game is a tie (neither player wins) N if the game is not done yet (no winner, spaces still available) When you initially create a char array, it initializes every element to the NULL character (an unprintable character).
The initBoard() method needs to set each of the elements in the array parameter board to a space ' ' character so that it looks like this: ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' You will know if your array is correctly initialized if, when you print the board the first time, it looks like a tic-tactoe board. As the game progresses, the board array will start to look like this: 'X' ' ' 'O' ' ' 'X' ' ' ' ' ' ' 'O' Implementing the initBoard() method The initialization is easiest to do with a nested for -loop structure, like this: 1. Loop r through all rows of board 1. Loop c through all columns in row r of board 1. Assign a space character ' ' to board[r][c] The moveHuman() method allows the human player to select a spot on the board to make a move. The move needs to be validated that the row and column are not out of bounds (i.e., less than 0 or greater than 2 ) and that the target spot is not already occupied. Here is the basic outline of what needs to happen in this method: 1. Loop until you get a valid move from the human player: Get a potential row and column position from the user The position should be within bounds (0 and 2 for both row and column) The potential spot they want to select should be a space (unoccupied) 2. With a valid position, mark the position of the human player's move with a capital X . The moveAI() method is the computer's artificial intelligence (AI) to make a move on the board. The AI is simply choosing random locations on the board that are not already occupied. Here is the basic outline of what needs to happen in this method: 1. Loop until you get a valid move for the computer player: Generate a random row between 0 and 2 (inclusive) Generate a random column between 0 and 2 (inclusive) The potential spot for row,column should be a space (unoccupied) 2. With a valid position, mark the position of the computer player's move with a capital 'O'
below is the starter code for the program:
import java.util.Random; import java.util.Scanner; public class TicTacToe { public static void main(String[] args) { char[][] board = new char[ 3 ][ 3 ]; // Initialize the board to spaces initBoard(board); // Print the game board printBoard(board); while (checkWinner(board) == 'N') { moveHuman(board); printBoard(board); if (checkWinner(board) == 'X' || checkWinner(board) == 'T') { break; } moveAI(board); printBoard(board); } // Check to see who the winner is if (checkWinner(board) == 'X') { System.out.println("It looks like X wins!"); } else if (checkWinner(board) == 'O') { System.out.println("It looks like O wins!"); } else { System.out.println("It looks like it's a tie!"); } // If the winner is 'X' or 'O', print that, otherwise, it is a tie } /** * Initializes the board to spaces. All elements will be set to a space * character. * * @param board The game board */ public static void initBoard(char[][] board) { } /** * Makes a move for the AI, and marks the board with an 'O'. * * @param board The game board */ public static void moveHuman(char[][] board) { Scanner in = new Scanner(System.in); } /** * Makes a move for the AI, and marks the board with an 'O'. * * @param board The game board */ public static void moveAI(char[][] board) { System.out.println("Now it's O's turn!"); } /**************** NOTHING ELSE NEEDS TO BE MODIFIED! *******************/ /** * Prints out the tic-tac-toe board * * @param board The game board */ public static void printBoard(char[][] board) { // Box drawing unicode characters: char a = '\u250c'; // U+250C : top-left char b = '\u2510'; // U+2510 : top-right char c = '\u2514'; // U+2514 : bottom-left char d = '\u2518'; // U+2518 : bottom-right char e = '\u252c'; // U+252C : top-vertical-connector char f = '\u2534'; // U+2534 : bottom-vertical-connector char g = '\u251c'; // U+251C : left-horizontal-connector char h = '\u2524'; // U+2524 : right-horizontal-connector char i = '\u253c'; // U+253C : center plus sign connector char j = '\u2500'; // U+2500 : horizontal char k = '\u2502'; // U+2502 : vertical String l = j + "" + j + "" + j; // Three horizontals // Print out the game board System.out.printf(" 0 1 2 " + " %c%s%c%s%c%s%c " + "0 %c %c %c %c %c %c %c " + " %c%s%c%s%c%s%c " + "1 %c %c %c %c %c %c %c " + " %c%s%c%s%c%s%c " + "2 %c %c %c %c %c %c %c " + " %c%s%c%s%c%s%c ", a, l, e, l, e, l, b, k, board[0][0], k, board[0][1], k, board[0][2], k, g, l, i, l, i, l, h, k, board[1][0], k, board[1][1], k, board[1][2], k, g, l, i, l, i, l, h, k, board[2][0], k, board[2][1], k, board[2][2], k, c, l, f, l, f, l, d); } /** * Checks the result of the game * * @param board The game board * @return 'X' if 'X' is the winner * 'O' if 'O' is the winner * 'T' if the game is a tie * 'N' if the game isn't finished */ public static char checkWinner(char[][] board) { if (board[0][0] == 'X' && board[0][1] == 'X' && board[0][2] == 'X' || // Check row 0 board[1][0] == 'X' && board[1][1] == 'X' && board[1][2] == 'X' || // Check row 1 board[2][0] == 'X' && board[2][1] == 'X' && board[2][2] == 'X' || // Check row 2 board[0][0] == 'X' && board[1][0] == 'X' && board[2][0] == 'X' || // Check col 0 board[0][1] == 'X' && board[1][1] == 'X' && board[2][1] == 'X' || // Check col 1 board[0][2] == 'X' && board[1][2] == 'X' && board[2][2] == 'X' || // Check col 2 board[0][0] == 'X' && board[1][1] == 'X' && board[2][2] == 'X' || // Check diag \ board[0][2] == 'X' && board[1][1] == 'X' && board[2][0] == 'X') { // Check diag / return 'X'; } else if (board[0][0] == 'O' && board[0][1] == 'O' && board[0][2] == 'O' || // Check row 0 board[1][0] == 'O' && board[1][1] == 'O' && board[1][2] == 'O' || // Check row 1 board[2][0] == 'O' && board[2][1] == 'O' && board[2][2] == 'O' || // Check row 2 board[0][0] == 'O' && board[1][0] == 'O' && board[2][0] == 'O' || // Check col 0 board[0][1] == 'O' && board[1][1] == 'O' && board[2][1] == 'O' || // Check col 1 board[0][2] == 'O' && board[1][2] == 'O' && board[2][2] == 'O' || // Check col 2 board[0][0] == 'O' && board[1][1] == 'O' && board[2][2] == 'O' || // Check diag \ board[0][2] == 'O' && board[1][1] == 'O' && board[2][0] == 'O') { // Check diag / return 'O'; } boolean finished = true; // If there is a blank space in the board, the game isn't finished yet for (int i = 0; i < board.length; i++) { for (int j = 0; j < board[ i ].length; j++) { if (board[ i ][ j ] == ' ') { finished = false; } } } // If the board is finished and 'X' or 'O' wasn't returned, then it is a tie // Otherwise, the game is not finished yet if (finished) { return 'T'; } else { return 'N'; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
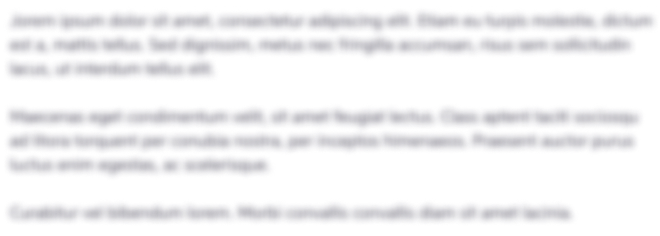
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started