In this assignment, you will create a program that allows theuser to draw in a window using brushes of different sizes andcolors. The user will
In this assignment, you will create a program that allows theuser to draw in a window using brushes of different sizes andcolors. The user will be able to change the size and color of thebrush using a menu, and the user will be able to clear the canvasto start a new drawing. A common technique used to draw a line isto use a series of solid circles. You can see this if you lookclosely at the lower portion of the letter J in the examplebelow.
I will have you build four classes for this program, each ofwhich you will place in a the same file. You will then build eachclass and, finally, build your main method and get the program torun. The UML diagram on the next page shows the structure of theprogram. When the user moves the mouse with the left mouse buttondown, the program will create and display a series of Circleobjects rendered on a DrawingPanel canvas. The DrawingPanel willexist inside a DrawingFrame, which is the top-level object youcreate in the main() method of the DrawingProgram class.
Getting Started To begin, create a Java file namedDrawingProgram.java. Within that file, create a public class namedDrawingProgram and build a main() method within it. Below thisclass (make sure you get below the closing curly brace of theDrawingProgram class), build each class as directed in theinstructions below.
The Circle Class This class will enable the user to draw ?lines?of various sizes and colors at specific locations. 1. Create aclass named Circle and place it within your source code file forthis assignment. 2. Create the following private data fields: a. Anint named size b. A Point named center for the location (this classhas an x,y coordinate) c. A Color named color to store the color ofthe circle the user draws 3. Create a constructor that acceptsan integer for the size, a Point for the location, and a Colorvariable as arguments. Choose appropriate names for the arguments.Within the constructor, call the corresponding set methods to setthe values passed in to the constructor. 4. Create get and setmethods for each field. a. In the setSize() method, make sure thesize is greater than or equal to zero. If an invalid value isprovided, set the size to 1. b. For the setLocation() method, novalidation is required. Simply assign the variable in the classholding the Point for the circle equal to the Point object passedinto the method. c. For the setColor() method, no validation isrequired. Simply assign the variable in the class holding the Colorequal to the Color object passed into the method. 5. Create apublic void method named draw that accepts a Graphics object namedg. Note: You will need to import the appropriate awt library forGraphics class to be recognized. Take a minute to research theGraphics class. Note that it has two methods, setColor() andfillOval(). We will need to use both of them. Within the method,write the code needed to accomplish the following tasks: a. Callthe setColor() method of Graphics object g, i.e. g.setColor(), andpass to it the Color stored within the class by calling getColor().b. Call the fillOval() method of the Graphics object. Note that itrequires four arguments. The first two are the x and y coordinatesof the oval (circle) to be drawn. For the first two arguments, callthe getCenter() method of Graphics object g to obtain the Point ofthe circle. Use the x and y attributes of the Point object. Thethird and fourth arguments are the width and height. Since we wantthe program to draw a circle, these two values should be the same.Use the getSize() method of the Circle object for botharguments.
The DrawingPanel Class This class provides the canvas upon whichthe circles are drawn. When you look at the figures back at thestart of the assignment, the white area within the window is aDrawingPanel object. Once you get the program working and startdrawing lines, you may notice that you are really just drawing aseries of circles in different colors and/or sizes. The faster youmove the mouse, the more you will notice this. Follow theinstructions on the next page to build this class. 1. Create theDrawingPanel class and declare it as follows: class DrawingPanelextends JPanel implements MouseMotionListener { }Inheriting theMouseMotionListener inheritance means that you?re going to have toimplement the mouseDragged() and mouseMoved() methods. 2. Createfour private data fields as described below. a. An integer to holdthe size (diameter) of the circles currently being drawn. b. Avariable to hold the Color for the circles currently bring drawnnamed circleColor. Note that this field is not the same as the oneyou declared in the Circle class. This field is designed to holdthe color the user wants to use to draw the ?lines? on the canvas.It will be passed to the individual, little circles that end upbeing drawn when the user moves the mouse around. c. A referencevariable that points to a Circle object. Do not instantiate aCircle object; just create a reference to one, like this: privateCircle drawingCircle; d. An ArrayList that can hold Circle objects.Refer back to Chapter 4, around page 140, or look up ArrayList onthe Web to learn more about it. Basically, an ArrayList is anarray, but it?s more than just that. Being an object, it has manyhelpful methods and it?s strongly typed, which means you have todeclare the type of objects you can store in it when you create it.Declare your ArrayList as shown: private ArrayList circleArrayList= new ArrayList(); 3. You?re going to need to implement a series ofmethods for the DrawingPanel class. a. Build the get and setmethods for size, circleColor, and location. Don?t worry aboutproviding validation, since the data will be sent from theDrawingFrame down into the DrawingPanel. b. Create a constructorthat takes two arguments ? a color and a size. Call the setSize()and setCircleColor() methods. After you write those two statements,write a third statement that makes this class aMouseMotionListener. To do this, write the following codestatement: addMouseMotionListener(this); c. Create apaintComponent() method that overrides the corresponding method ofits parent (the JFrame class, who inherits the method from it?sparent, the JComponent class). The heading for this method shouldbe written as follows: public void paintComponent(Graphics g) Thefirst thing we must do within this method is call the superclassmethod so that all of the default things take place.super.paintComponent(g);
Next, create an Iterator to loop through the circleArrayListthat we created as a field Iterator circleIterator =circleArrayList.iterator(); Create a local Circle object to drawcalled drawCircle and use it in a while loop to iterate through theArrayList and draw the Circle objects within it. Here is the whileloop code: Circle drawCircle; // iterate, move through theArrayList element by element while( circleIterator.hasNext() ) {drawCircle = (Circle) circleIterator.next(); drawCircle.draw(g); //draw each circle } d. Create a mouseDragged() method (see page 847for details). The mouseDragged() method heading is: public voidmouseDragged( MouseEvent event ) This event occurs when a mousebutton is held down and the user moves the mouse. What we want hereis to let the user erase when the right button is down and drawwhen the left button is down. To do this, we must begin by askingwhich button is being held down by the user. To test for the rightbutton press, we can use the conditional expression if (event.isMetaDown() ) If the right mouse button is down, draw acircle whose color is the color of the background. You can do thiswith a line of code like this (the methods names may be differentdepending on how you named your fields): Circle newCircle = newCircle( getCircleDiameter(), event.getPoint(), this.getBackground()); If the user clicked the left button, then you should draw acircle using the selected color. Use the same syntax as above, onlyreplace the third argument with getCircleColor(). In either case,add the newCircle to the ArrayList like this: circleArrayList.add(newCircle ); Finally, repaint the panel to display the newly drawncircle using the repaint() command. 3.5. Create a mouseMoved()method. This method does nothing, so there shouldn't be any codebetween the { and } curly brackets.
Create the DrawingFrame Class The DrawingFrame class containsthe user interface for the user to set the color and size of thelines to draw. It also houses the drawingPanel. The DrawingFrame isa JFrame class which, of course, will need to implement theActionListener interface. Therefore, we will need to create an PAGE5 OF 7 CIS 117 JAVA PROGRAMMING I PROFESSOR T. KOETSactionPerformed method. We covered this in the last assignment, andwe will do this again in a bit. But first things first. 1. First ofall, you must declare and implement the fields. a. Create aDrawingPanel field: private DrawingPanel drawPanel; b. Create aButtonGroup for both the color selector radio buttons and anotherfor the size selector radio buttons. If you use menu items, you donot need the button groups. c. Create four constants (final typesin Java) in this class d. final int SMALL = 4; final int MEDIUM =8; final int LARGE = 10; Use whatever values you want for the sizes? 4, 8, and 10; 6, 10, and 12; 8, 12, and 14 ? it?s up to you aslong as the sizes are distinct enough to be practical. Note: Tocreate constants in Java, you declare the variable using the finalkeyword, which means you can't change the values at run time. Thiskeyword is similar to const in C++ (but not exactly the same). 2.Create two methods for the class as described. a. Create aconstructor. Within the constructor, set up the DrawingPanel topaint small, black circles. Let?s assume you call your objectdrawPanel (you must use the name you gave your DrawingPanel whenyou created it up in the fields section of the class). Remember, aDrawingPanel takes two arguments ? color and size. Then, set thebackground color of the panel to white. drawPanel = newDrawingPanel( Color.BLACK, SMALL ); drawPanel.setBackground(Color.WHITE ); You will need to add the drawPanel to the centerlocation of the main frame. You will also need to add actionlisteners to all of the menu items that will respond to a userclicking on that option. objectName.addActionListener(this); If youuse radio buttons add them to the corresponding group ButtonGroupaGroup = new ButtonGroup(); aGroup.add( redRadioButton ); Repeatfor other color radio buttons. Repeat for size buttons if you useradio buttons. Add mnemonics to your menu items, only be sure notto use the same letter twice. Finally, add the drawPanel to thecenter of the frame. PAGE 6 OF 7 CIS 117 JAVA PROGRAMMING IPROFESSOR T. KOETS b. Build the actionPerformed method. Since theDrawingFrame implements the ActionListener interface, it mustdefine an actionPerformed method. Don't panic, this one is easy,just a bunch of if statements. If you use radio buttons in yourmenu, you can use a similar if test, one for each radio button: if(redRadioButton.isSelected() ) drawPanel.setCircleColor( Color.RED); if (smallRadioButton.isSelected() ) drawPanel.setCircleDiameter(SMALL ); If you use JMenuItems, you need to get the action command:public void actionPerformed( ActionEvent event ) { String arg =event.getActionCommand(); ... } Then use the equals() method tocheck for which menu item was selected. Here are two examples:if(arg.equals("Exit") ) { System.exit(0); } if(arg.equals("About")) { JOptionPane.showMessageDialog(null, "Drawing Program, version1.0 by Tim KoetsMarch 6, 2016"); } Build the DrawingProgramClass Since you?ve already created the class and the main() methodstub, you now need to put code within main() to get the programrunning. Your main() method should create a DrawingFrame object,set it?s title and location, assign a default close operation, andfinally make it visible
Drawing Program File Size Color Help Clear Exit JAVA X Drawing Program File Size Color Help
Step by Step Solution
3.46 Rating (153 Votes )
There are 3 Steps involved in it
Step: 1
Sure I understand your instructions Here is a summary of the steps involved in creating the four classes for your drawing program Circle class Create ...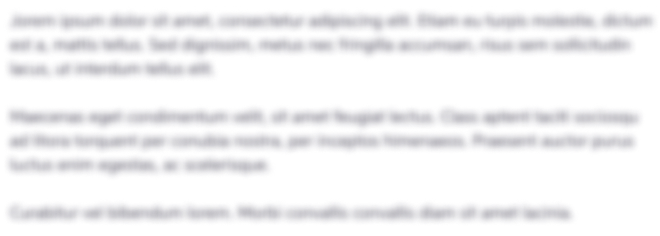
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started