Question
In this assignment, you will implement a sort method on singly-linked and doubly-linked lists. Implement the following sort member function on a singly-linked list: void
In this assignment, you will implement a sort method on singly-linked and doubly-linked lists.
Implement the following sort member function on a singly-linked list:
void sort(bool(*comp)(const T &, const T &) = defaultCompare);
Implement the following sort member function on a doubly-linked list:
void sort(bool(*comp)(const T &, const T &) = defaultCompare);
The sort() methods take as a parameter a comparator function, having a default assignment of defaultCompare, a static function defined as follows:
template
defaultCompare is defined as a static function in the header file compare.h. Both sort algorithms must provide ascending and descending sorts by switching comparators.
You may use any sort algorithm you choose. If you implement the quick-sort algorithm (successfully!) for both lists, you may earn an extra 5 points.
The starter files are provided below. Do not alter the class definition in any way. Use the driver provided below. Programs that crash are subject to a 50% penalty. Please submit the class implementation files only (slist.h and dlist.h). PLEASE NOTE: You may not use any Standard Template Library (STL) classes for this assignment. Use code provided below only.
Please test and compile your code for errors before submitting code that contains bugs or that is not working. Thanks!
compare.h:
#pragma once
template
static bool defaultCompare(const T &key1, const T &key2) {
return key1
}
dlist.h:
#pragma once
#include
#include "compare.h"
namespace cs20a
{
template
template
template
template
template
template
class dlist_node {
friend class dlist
friend class dlist_iterator
friend class const_dlist_iterator
friend class reverse_dlist_iterator
friend class const_reverse_dlist_iterator
private:
dlist_node() {};
dlist_node(const T& t, dlist_node
: value(t), prev(prev), next(next) {};
T value;
dlist_node
};
template
class dlist_iterator {
friend class dlist
public:
T& operator*() const { return np->value; };
bool operator == (const dlist_iterator
bool operator!=(const dlist_iterator
const dlist_iterator
np = np->next;
return *this;
};
const dlist_iterator
dlist_iterator
np = np->next;
return clone;
};
private:
dlist_node
dlist_iterator(dlist_node
};
template
class reverse_dlist_iterator {
friend class dlist
public:
T& operator*() const { return np->value; };
bool operator == (const reverse_dlist_iterator
bool operator!=(const reverse_dlist_iterator
const reverse_dlist_iterator
np = np->prev;
return *this;
};
const reverse_dlist_iterator
reverse_dlist_iterator
np = np->prev;
return clone;
};
private:
dlist_node
reverse_dlist_iterator(dlist_node
};
template
class const_dlist_iterator {
friend class dlist
public:
T const& operator*() const { return np->value; };
bool operator == (const const_dlist_iterator
bool operator!=(const const_dlist_iterator
const const_dlist_iterator
np = np->next;
return *this;
};
const const_dlist_iterator
const_dlist_iterator
np = np->next;
return clone;
};
private:
const dlist_node
const_dlist_iterator(const dlist_node
};
template
class const_reverse_dlist_iterator {
friend class dlist
public:
T const& operator*() const { return np->value; };
bool operator == (const const_reverse_dlist_iterator
bool operator!=(const const_reverse_dlist_iterator
const const_reverse_dlist_iterator
np = np->prev;
return *this;
};
const const_reverse_dlist_iterator
const_reverse_dlist_iterator
np = np->prev;
return clone;
};
private:
const dlist_node
const_reverse_dlist_iterator(const dlist_node
};
template
class dlist {
public:
typedef dlist_iterator
typedef const_dlist_iterator
typedef reverse_dlist_iterator
typedef const_reverse_dlist_iterator
public:
dlist();
dlist(const dlist
~dlist();
dlist
bool operator==(const dlist
bool operator!=(const dlist
void push_back(const T& element);
T pop_back();
void push_front(const T& element);
T pop_front();
T& front();
const T& front() const;
T& back();
const T& back() const;
bool empty() const;
bool full() const;
void clear();
long size() const;
void remove(const T& element);
bool contains(const T& element) const;
void sort(bool(*comp)(const T &, const T &) = defaultCompare);
iterator begin() { return dlist_iterator
iterator end() { return dlist_iterator
reverse_iterator rbegin() const { return reverse_dlist_iterator
reverse_iterator rend() const { return reverse_dlist_iterator
const_iterator cbegin() const { return const_dlist_iterator
const_iterator cend() const { return const_dlist_iterator
const_reverse_iterator crbegin() const { return const_reverse_dlist_iterator
const_reverse_iterator crend() const { return const_reverse_dlist_iterator
iterator insert(iterator position, const T& element) {
dlist_node
dlist_node
if (successor->prev != nullptr)
successor->prev->next = target;
successor->prev = target;
if (head->next == successor)
head->next = target;
_size++;
return iterator(target);
}
iterator erase(iterator position) {
dlist_node
dlist_node
killNode(target);
return iterator(successor);
}
private:
dlist_node
long _size;
bool(*compare)(const T &, const T &);
void makeEmpty();
void init();
void copy(const dlist
void killNode(dlist_node
dlist_node
};
template
dlist
init();
}
template
dlist
init();
copy(rhs);
}
template
dlist
if (this != &rhs) {
clear();
copy(rhs);
}
return *this;
}
template
dlist
makeEmpty();
}
template
void dlist
dlist_node
if (empty())
head->next = node;
else
tail->prev->next = node;
tail->prev = node;
_size++;
}
template
T dlist
if (empty())
throw std::logic_error("Empty list.");
T element = tail->prev->value;
killNode(tail->prev);
return element;
}
template
void dlist
dlist_node
if (empty())
tail->prev = node;
else
head->next->prev = node;
head->next = node;
_size++;
}
template
T dlist
if (empty())
throw std::logic_error("Empty list.");
T element = head->next->value;
killNode(head->next);
return element;
}
template
T& dlist
return head->next->value;
}
template
const T & dlist
return head->next->value;
}
template
T& dlist
return tail->prev->value;
}
template
const T & dlist
return tail->prev->value;
}
template
bool dlist
return head->next == nullptr;
}
template
bool dlist
return false;
}
template
void dlist
makeEmpty();
init();
}
template
long dlist
return _size;
}
template
bool dlist
if (this == &rhs)
return true;
if (size() != rhs.size())
return false;
dlist_node
dlist_node
while (ptr1 != nullptr || ptr2 != nullptr)
{
if (ptr1->value != ptr2->value)
return false;
ptr1 = ptr1->next;
ptr2 = ptr2->next;
}
return true;
}
template
bool dlist
return !(*this == rhs);
}
template
void dlist
dlist_node
while (p != nullptr) {
push_back(p->value);
p = p->next;
}
}
template
dlist_node
dlist_node
while (ptr != nullptr && ptr->value != element)
ptr = ptr->next;
return ptr;
}
template
void dlist
header.prev = header.next = nullptr;
tailer.prev = tailer.next = nullptr;
head = &header;
tail = &tailer;
_size = 0;
}
template
void dlist
if (target->next != nullptr)
target->next->prev = target->prev;
if (target->prev != nullptr)
target->prev->next = target->next;
if (head->next == target)
head->next = target->next;
if (tail->prev == target)
tail->prev = target->prev;
delete target;
_size--;
}
template
void dlist
if (_size == 0)
return;
//*** Implement this function ***
}
template
void dlist
dlist_node
dlist_node
while (ptr != nullptr) {
target = ptr;
ptr = ptr->next;
delete target;
}
}
template
void dlist
dlist_node
if (target == nullptr)
throw std::logic_error("Node not found.");
killNode(target);
}
template
bool dlist
dlist_node
return node != nullptr;
}
}
slist.h:
#pragma once
#include
#include "compare.h"
namespace cs20a
{
template
template
template
template
template
class slist_node {
friend class slist
friend class slist_iterator
friend class const_slist_iterator
private:
slist_node() {};
slist_node(const T& t, slist_node
T value;
slist_node
};
template
class slist_iterator {
friend class slist
public:
T& operator*() const { return np->value; };
bool operator == (const slist_iterator
bool operator!=(const slist_iterator
const slist_iterator
np = np->next;
return *this;
};
const slist_iterator
slist_iterator
np = np->next;
return clone;
};
private:
slist_node
slist_iterator(slist_node
};
template
class const_slist_iterator {
friend class slist
public:
T const& operator*() const { return np->value; };
bool operator == (const const_slist_iterator
bool operator!=(const const_slist_iterator
const const_slist_iterator
np = np->next;
return *this;
};
const const_slist_iterator
const_slist_iterator
np = np->next;
return clone;
};
private:
const slist_node
const_slist_iterator(const slist_node
};
template
class slist {
public:
typedef slist_iterator
typedef const_slist_iterator
public:
slist();
slist(const slist
~slist();
slist
bool operator==(const slist
bool operator!=(const slist
void push_back(const T& element);
T pop_back();
void push_front(const T& element);
T pop_front();
T& front();
const T& front() const;
T& back();
const T& back() const;
bool empty() const;
bool full() const;
void clear();
long size() const;
void remove(const T& element);
bool contains(const T& element) const;
void sort(bool(*comp)(const T &, const T &) = defaultCompare);
iterator begin() { return slist_iterator
iterator end() { return slist_iterator
const_iterator cbegin() const { return const_slist_iterator
const_iterator cend() const { return const_slist_iterator
iterator insert(iterator position, const T& element) {
slist_node
slist_node
if (head->next == successor)
head->next = target;
_size++;
return iterator(target);
}
iterator erase(iterator position) {
slist_node
slist_node
killNode(target);
return iterator(successor);
}
private:
slist_node
long _size;
bool(*compare)(const T &, const T &);
void makeEmpty();
void init();
void copy(const slist
void killNode(slist_node
slist_node
slist_node
};
template
slist
init();
}
template
slist
init();
copy(rhs);
}
template
slist
if (this != &rhs) {
clear();
copy(rhs);
}
return *this;
}
template
slist
makeEmpty();
}
template
void slist
slist_node
if (empty())
head->next = node;
else
tail->next = node;
tail = node;
_size++;
}
template
T slist
if (empty())
throw std::logic_error("Empty list.");
T element = tail->value;
killNode(tail);
return element;
}
template
void slist
slist_node
if (empty())
tail = node;
head->next = node;
_size++;
}
template
T slist
if (empty())
throw std::logic_error("Empty list.");
T element = head->next->value;
killNode(head->next);
return element;
}
template
T& slist
return head->next->value;
}
template
const T & slist
return head->next->value;
}
template
T& slist
return tail->value;
}
template
const T & slist
return tail->value;
}
template
bool slist
return head->next == nullptr;
}
template
bool slist
return false;
}
template
void slist
makeEmpty();
init();
}
template
long slist
return _size;
}
template
bool slist
if (this == &rhs)
return true;
if (size() != rhs.size())
return false;
slist_node
slist_node
while (p1 != nullptr || p2 != nullptr)
{
if (p1->value != p2->value)
return false;
p1 = p1->next;
p2 = p2->next;
}
return true;
}
template
bool slist
return !(*this == rhs);
}
template
void slist
slist_node
while (p != nullptr) {
push_back(p->value);
p = p->next;
}
}
template
void slist
header.next = nullptr;
head = tail = &header;
_size = 0;
}
template
void slist
slist_node
previous->next = target->next;
if (tail == target)
tail = previous;
delete target;
_size--;
}
template
void slist
if (_size == 0)
return;
//*** Implement this function ***
}
template
void slist
slist_node
slist_node
while (ptr != nullptr) {
target = ptr;
ptr = ptr->next;
delete target;
}
}
template
slist_node
slist_node
while (ptr != nullptr && ptr->value != element)
ptr = ptr->next;
return ptr;
}
template
slist_node
slist_node
while (ptr->next != nullptr && ptr->next != node)
ptr = ptr->next;
return ((ptr->next != nullptr) ? ptr : nullptr);
}
template
void slist
slist_node
if (target == nullptr)
throw std::logic_error("Node not found.");
killNode(target);
}
template
bool slist
slist_node
return node != nullptr;
}
}
ListMenu.cpp:
// Menu.cpp : Defines the entry point for the console application.
//
#define DOUBLE
#include
#include "slist.h"
#include "dlist.h"
using namespace std;
using namespace cs20a;
enum CHOICE { APPEND, REMOVE, CONTAINS, SIZE, MAKEEMPTY, ISEMPTY, QUIT, PRINT, SORTASCENDING, SORTDESCENDING, OTHER };
CHOICE menu();
void printList(slist
void printList(dlist
bool sortAscending(const int &val1, const int &val2);
bool sortDescending(const int &val1, const int &val2);
int main(int argc, char* argv[]) {
#ifdef SINGLE
slist
#else
dlist
#endif
int value = 0;
CHOICE choice;
do {
choice = menu();
switch (choice) {
case MAKEEMPTY:
list.clear();
break;
case ISEMPTY:
if (list.empty())
cout
else
cout
break;
case SIZE:
cout
break;
case REMOVE:
cout
cin >> value;
list.remove(value);
break;
case APPEND:
cout
cin >> value;
srand(value);
cout
cin >> value;
for (int i = 0; i
list.push_back(rand() % value);
break;
case CONTAINS:
cout
cin >> value;
if (list.contains(value))
cout
else
cout
break;
case PRINT:
printList(list);
break;
case SORTASCENDING:
list.sort(sortAscending);
break;
case SORTDESCENDING:
list.sort(sortDescending);
break;
case OTHER:
cout
}
} while (choice != QUIT);
return(0);
}
CHOICE menu() {
char choice;
CHOICE result;
cout
cin >> choice;
switch (choice) {
case 'M':
case 'm':
result = MAKEEMPTY;
break;
case 'I':
case 'i':
result = ISEMPTY;
break;
case 'A':
case 'a':
result = APPEND;
break;
case 'R':
case 'r':
result = REMOVE;
break;
case 'C':
case 'c':
result = CONTAINS;
break;
case 'S':
case 's':
result = SIZE;
break;
case 'P':
case 'p':
result = PRINT;
break;
case 'Q':
case 'q':
result = QUIT;
break;
case '1':
result = SORTASCENDING;
break;
case '2':
result = SORTDESCENDING;
break;
default:
result = OTHER;
break;
}
return(result);
}
void printList(slist
if (l.empty())
cout
else {
for (slist
cout ";
}
cout
}
}
void printList(dlist
if (l.empty())
cout
else {
for (dlist
cout ";
}
cout
}
}
bool sortAscending(const int & val1, const int & val2) {
return val1
}
bool sortDescending(const int & val1, const int & val2) {
return val1 > val2;
}
slist sll srand (837) for (int i 0; i K 100 1++ sll push back (rand()); sll. Sort sll sort descendingCompare) default: ascending sort descending Compare static compare functionStep by Step Solution
There are 3 Steps involved in it
Step: 1
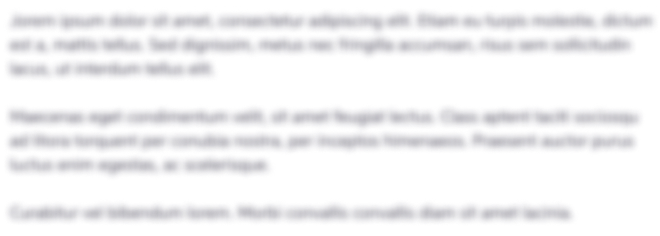
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started