Question
In this assignment, you will practice basic C programming by performing a simple task: encoding a stream of data into a base64 format. All diagnostic
In this assignment, you will practice basic C programming by performing a simple task: encoding a stream of data into a base64 format. All diagnostic and error messages should be printed to stderr. Your program must run in O(1) memory for any size of input. To put this more explicitly, you cannot have a massive array that you read all of the input into before processing. You must implement a stream processor that reads a minimally sized chunk of input and produces a corresponding chunk of output, in a loop.
If a filename is passed as an argument, you do not need to parse the filename or validate it yourself. Let fopen do that for you; it will return an error if the file cannot be opened. Whether you open a file or use stdin as a default source when no file is to be opened, the remainder of your program should be exactly the same regardless. Notice that the stdin variable has a type FILE*, and that fopen also returns a type FILE*. Perhaps you can use a generic variable of type FILE* to refer to the input stream throughout your program (HINT!), and only change it if there is a file to open (HINT!).
I am also providing the following snippet as an alphabet array, as described in the base64 specification.
static char const alphabet[] = "ABCDEFGHIJKLMNOPQRSTUVWXYZ" "abcdefghijklmnopqrstuvwxyz" "0123456789+/=";
To properly encode the data, you need to read in three bytes (8 bits * 3 = 24 bits) and then split this into four 6-bit values which then index into the alphabet array to determine the four characters of output. This requires some bit manipulation. The code below gives you the numeric index of the output character (0-63) which you then need to look up in the alphabet[] array to find an actual character to output!
uint8_t in[3]; uint8_t out_idx[4]; // Upper 6 bits of byte 0 out_idx[0] = in[0] >> 2; // Lower 2 bits of byte 0, shift left and or with the upper 4 bits of byte 1 out_idx[1] = ((in[0] & 0x03u) << 4) | (in[1] >> 4); // Lower 4 bits of byte 1, shift left and or with ??? out_idx[2] = ((in[1] & 0x0Fu) << 2) | // ??? fill in the rest of this statement out_idx[3] = // What comes next?
Here is some psudocode to use as template for this assignment:
int main(int argc, char *argv[]) { int input_fd = STDIN_FILENO; /* TODO: If there is a FILE argument and it isn't "-", then open that file and point input_fd at the open file. */ while (1) { /* TODO: read three bytes */
/* TODO: If end-of-file AND no bytes to process, print newline and exit */
/* TODO: process input using base64 algorithm */
/* TODO: Write four output bytes */
/* TODO: If end-of-file, print newline and exit */
/* TODO: If count == 76, print a newline */ } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
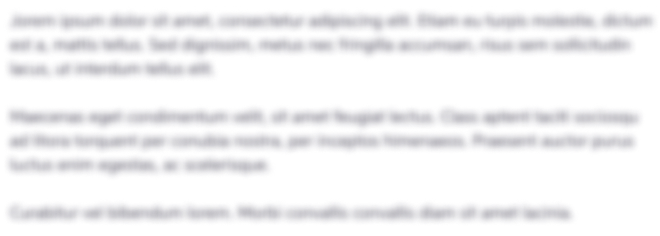
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started