Question
In this assignment, you will refactor the program that you wrote for Assignment 3. The output of this program should be exactly the same as
In this assignment, you will refactor the program that you wrote for Assignment 3. The output of this program should be exactly the same as Assignment 3 except that the data will be sorted. The program should present a menu to the user asking if they would like display the average grade, maximum grade, or minimum grade. The program should then display the information in a table. The table should include the student name along with the chosen data (average, minimum, or maximum). In addition, the information should be sorted from high to low by the data selected. Instead of using parallel arrays, you will need one vector of a struct of students. You can use the following struct: struct Student { string fName; string lName; double average; int max; int min; }; The program should do the following: ? Load the names of students into a vector of Student struct. For each student calculate the average, maximum, and minimum grade and store that in the struct as well. The file used will be called NamesGrades.txt. ? Continuously present a menu to the user given him/her the options of average, maximum, minimum, or quit. If the user chooses to quit, the program should end otherwise the presentation of the menu should continue. ? Display a table of appropriate grades based on the users selection sorted by the appropriate grades. Make sure you use good programming style. This includes commenting ALL your variables and commenting throughout your code. Comments should explain why you are doing something. Use good indentation (see my examples and the books examples for demonstration of good indentation). Make sure variable names are self-documenting. Make good use of white space. Group logical sections together with one blank line between logical sections.
// Global variables
const int MAX_STUDENTS = 25; // We will not process more than 25 students even if the file contains more
const int MAX_GRADES = 5; // Each student has exactly 5 grades
const string FILENAME = "NamesGrades.txt"; // The name of the file that it'll read
// Function declarations
int loadStudentNamesGrades(string students[], int grades[][MAX_GRADES], string fileName, int maxStudents);
void displayAverages(string students[], int grades[][MAX_GRADES], int studentCount);
void displayMax(string students[], int grades[][MAX_GRADES], int studentCount);
void displayMin(string students[], int grades[][MAX_GRADES], int studentCount);
char getLetterGrade(double grade);
int getLongestNameLength(string students[], int studentCount);
int main()
{
int studentCount = 0; // Number of students processing
int grades[MAX_STUDENTS][MAX_GRADES]; // Two dimensional array: Table of grades for students
string students[MAX_STUDENTS]; // Array: List of student's name
char choice; // Choice for user menu
// Get students and grades
studentCount = loadStudentNamesGrades(students, grades, FILENAME, MAX_STUDENTS);
// Loop until user says to quit
do
{
// present menu and get user's choice
cout << " Student Grade Report Program ";
cout << "\t1. Display Average Grade "
<< "\t2. Display Maximum Grade "
<< "\t3. Display Minimum Grade "
<< "\t4. Quit Program ";
cout << "Enter your choice (1-4): ";
cin >> choice;
while (getchar() != ' ');
// Process the choice
switch (choice)
{
case '1': // Average
displayAverages(students, grades, studentCount);
break;
case '2': // Maximum
displayMax(students, grades, studentCount);
break;
case '3': // Minimum
displayMin(students, grades, studentCount);
break;
case '4': // Quit
break;
default:
cout << "Invalid option. Please try again. ";
}
if (choice != '4')
{
cout << endl;
system("PAUSE");
system("CLS");
}
} while (choice != '4'); // End of do while loop
// End of program
// Make sure we place the end message on a new line
cout << endl;
system("PAUSE");
return 0;
}
int loadStudentNamesGrades(string students[], int grades[][MAX_GRADES], string fileName, int maxStudents)
{
ifstream inFile; // Input File Stream
string studentName, // Name of student
studentLastName; // Last name of student
int numStudents = 0; // Number of students actually read
// Open the file
inFile.open(fileName);
if (!inFile)
{
cout << "File not found " << fileName << endl;
system("PAUSE");
exit(EXIT_FAILURE);
}
// Loop through each row
for (int i = 0;
i < maxStudents && (inFile >> studentName >> studentLastName);
i++, numStudents++)
{
// Loop through all the grades of each row
for (int j = 0; j < MAX_GRADES; j++)
{
inFile >> grades[i][j];
}
// Combine the student's name with the student's last name
students[i] = studentName + " " + studentLastName;
}
// Close the file
inFile.close();
return numStudents;
}
void displayAverages(string students[], int grades[][MAX_GRADES], int studentCount)
{
double average; // Average grade if all student's grades
int total; // Total of all grades (Accumulator)
// Get the longest name
int maxLength = getLongestNameLength(students, studentCount); // Longest name
// Set up Table header
cout << setprecision(1) << fixed << showpoint;
cout << " Grade Averages ";
cout << setw(maxLength + 1) << left << "Name"
<< setw(8) << right << "Average"
<< setw(8) << "Grade" << endl;
// Students
for (int i = 0; i < studentCount; i++)
{
cout << setw(maxLength + 1) << left << students[i];
total = 0; // Zeros out accumulator
// Process the grades
for (int j = 0; j < MAX_GRADES; j++)
{
total += grades[i][j];
}
average = static_cast
cout << setw(8) << right << average
<< setw(8) << getLetterGrade(average)
<< endl;
}
}
void displayMax(string students[], int grades[][MAX_GRADES], int studentCount)
{
int maxGrade; // Max grade so far
int maxLength = getLongestNameLength(students, studentCount); // Longest name
// Header
cout << " Max grades ";
cout << setw(maxLength + 1) << left << "Name"
<< setw(4) << right << "Max"
<< setw(8) << "Grade" << endl;
// Max Grades
for (int i = 0; i < studentCount; i++)
{
cout << setw(maxLength + 1) << left << students[i];
maxGrade = grades[i][0]; // Initial Grade
for (int j = 0; j < MAX_GRADES; j++)
{
if (grades[i][j] > maxGrade)
{
maxGrade = grades[i][j];
}
}
cout << setw(4) << right << maxGrade
<< setw(8) << getLetterGrade(maxGrade)
<< endl;
}
}
void displayMin(string students[], int grades[][MAX_GRADES], int studentCount)
{
int minGrade; // Max grade so far
int maxLength = getLongestNameLength(students, studentCount); // Longest name
// Set up the header
cout << " Min grades ";
cout << setw(maxLength + 1) << left << "Name"
<< setw(4) << right << "Min"
<< setw(8) << "Grade" << endl;
// Min Grades
for (int i = 0; i < studentCount; i++)
{
cout << setw(maxLength + 1) << left << students[i];
minGrade = grades[i][0]; // Initial Grade
for (int j = 0; j < MAX_GRADES; j++)
{
if (grades[i][j] < minGrade)
{
minGrade = grades[i][j];
}
}
cout << setw(4) << right << minGrade
<< setw(8) << getLetterGrade(minGrade)
<< endl;
}
}
char getLetterGrade(double grade)
{
if (grade >= 90.0)
return 'A';
else if (grade >= 80.0)
return 'B';
else if (grade >= 70.0)
return 'C';
else if (grade >= 60.0)
return 'D';
else
return 'F';
}
int getLongestNameLength(string students[], int studentCount)
{
int maxLength = 0; // Max length so far
for (int i = 0; i < studentCount; i++)
{
// Is it longer
if (students[i].length() > maxLength)
{
maxLength = students[i].length();
}
}
return maxLength;
}
Has to be written in c++ and compile in visual studio 2017
Step by Step Solution
There are 3 Steps involved in it
Step: 1
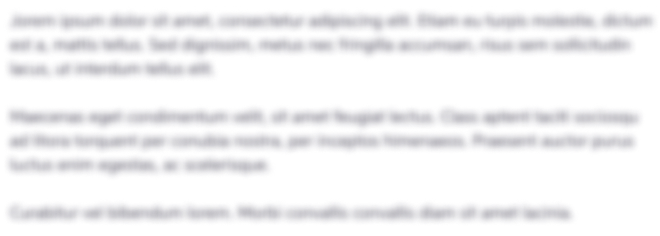
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started