Question
In this assignment you will refine the Circle class in order to practice the following advanced class writing techniques: 1)Aggregation 2)Multiple Constructors 3)Copy Constructors 4)The
In this assignment you will refine the Circle class in order to practice the following advanced class writing techniques:
1)Aggregation
2)Multiple Constructors
3)Copy Constructors
4)The toString method
5)The equals method
The final goal is to create a class with the following design. Class uses doubles, instead of the floats in the first assignment. The new members and methods are listed in green.
Class Circle
Point center // This sentence is green double radius |
Circle(Point o, double r) //This sentence is green Circle(double xValue, double yValue, double r) // green Circle() //green Circle(Circle c) //green
Point getCenter() //green void setCenter(Point p) //green
void setX(double value) double getX()
void setY(double value) double getY()
void setRadius(double value) double getRadius()
double getArea()
String toString() //green boolean equals(Circle c) //green
boolean doesOverlap(Circle c) |
Step 1: The Point class
Begin by downloading Point.java. Take some time to familiarize yourself with the class. It is a simple class for storing an x and y coordinate.
Add a method named distance that accepts a Point as an argument and return the distance between this argument, and the point that receives the invocation. It should have the following signature:
public double distance(Point other)
Step 2: Aggregation
In the previous circle class assignment the class was given three instance members: x, y, and radius. Create a new Circle class that uses has only two members: a member named center of type Point, and member radius of type double.
Rewrite the existing setters and getters for x and y so that they use the center member. Add setters and getters for the center, so that users of the class can access the point directly.
Rewrite the doesOverlap method so that it uses the center member.
Step 3: Constructors
Create four constructors. Each constructor is described below:
The two argument constructor. This constructor should accept a Point object and double. It should initialize the new objects center member so that it refers to a copy of the point argument, and the radius member to the double value.
The three argument constructor. This constructor should accept three double values, for x, y, and radius respectively. It should create a new Point object to store the x and y values, and store this Point object in the center member. It should store the third double value into the radius member. Note that you can use the two argument constructor to write the three argument constructor.
The no-argument constructor. This constructor should create a new Point object with an x and y coordinate of 0, and use this point object to initialize the center member. It should set the radius to 1.
The copy constructor. This constructor should accept a Circle as an argument. It should set the center member to a copy of the point arguments center member. It should set the radius member to the point arguments radius value;
Step 4: toString
Write a toString method for your Circle class. You format the string however you like, as long as it includes the objects x, y, and radius values.
Step 5: equals
Write an equals method that returns true if the argument Circle has the same x, y and radius values as the receiving circle.
What to Submit?
Submit all of the files necessary to compile your project. That is probably Circle.java, Point.java, and code that contains a main method that tests each of the new features. You can put your main method in the circle class, or in a separate tester class. The main method should construct circles using all four constructors. It should test the doesOverlap, toString, and equals methods.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
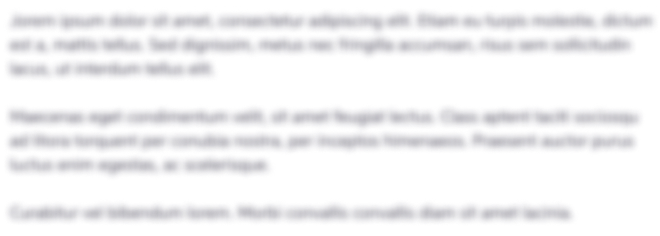
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started