Question
In this assignment, you will update the Money, Date, Bill, and ArrayList classes from previous homeworks using new ideas that we have discussed in class.
In this assignment, you will update the Money, Date, Bill, and ArrayList classes from previous homeworks using new ideas that we have discussed in class. Build a driver to test the functionality. Your code should compile with your driver, but note that you will want to test your code more fully, as I will also do.
1. Fix any privacy (and other) errors that were noted in your comments for the previous iteration of this homework.
2. Modify the Money, Date, and Bill class to implement the Comparable interface. The supplied driver performs a simple test on this. Remember that compareTo takes an Object parameter and you should check to make sure that the object is actually a Money object.
3. Modify the Money, Date, and Bill classes to implement the Cloneable interface. Note that Money and Date can simply copy their private instance variables, since they store only primitive and immutable types. However, you will need to override the clone method, to make it public, since it is protected in the Object class. The Bill class will need to do more, since it incorporates the Money and Date classes, which are mutable. Note that it can (and should) use the clone methods of those classes. Be sure to remove any use of the copy constructor for Money, Date, and Bill in the rest of the code (the definition can exist, but dont use it in other classes; use the clone method instead).
4. Build a class ExpenseAccount that extends your ArrayList. You should remove the limit on the number of bills that can be placed in an account by making your ArrayList dynamically resize itself.
5. Modify Money, Bill, and Date to implement the Serializable interface.
6. There is a separate extra credit portion that builds on this assignment.
/**
* Money.java
*
*/
public class Money {
private int dollar;// instance variable for dollar
private int cents;// instance variable for cent
// Constructor that sets the dollar amount and cents equal to zero if dollar
// less than zero
public Money(int dol) {
this.dollar = dol;// initialize dollar amount
// case when dollar is less than zero
if (dollar < 0) {
dollar = 0;// dollar becomes zero
}
}
// Constructor which initialize dollar and cent amounts
public Money(int dol, int cent) {
this.dollar = dol;// initialize dollar
this.cents = cent;// initialize cent
if (dollar < 0) {
dollar = 0;// dollar becomes zero
this.cents = cent;// the rest cents stays the same
}
}
// Copy constructor
public Money(Money other) {
this.dollar = other.dollar;// copy another dollar amount
this.cents = other.cents;// copy another cent amount
}
// get dollar amount
public int getDollars() {
return dollar;
}
// get cent amount
public int getCents() {
return cents;
}
// set the valid range for dollar and cent
public void setMoney(int dollars, int cent) {
this.dollar = dollars;// initialize dollar
this.cents = cent;// initialize cent
// cases when dollar is less than zero
if (dollar < 0) {
dollar = 0;// set dollar amount equal to zero
cents = cent;// set cents as the amount cents left
}
// case when cent is less than zero
if (cents < 0) {
cents = 0;// set cent equal to zero
}
// cases when cent is greater than 99
if (cents > 99) {
dollar += cents / 100;// dollar add up if cents greater than 100
cents = cents % 100;// the rest of cents becomes the new cents
}
}
//get the entire amount of money with dollars and cents
public double getMoney() {
return dollar + cents / 100.0;//return dollars and cents
}
//add new dollars into the current amount of dollars
public void add(int dollars) {
this.dollar += dollars;
}
//add new amount of dollars and cents to the current amount of dollars and cents
public void add(int dollars, int cents) {
//case when cents greater than 99
if (cents > 99) {
this.dollar += cents / 100;//dollar increments if there are more than 100 cents
this.cents += cents % 100;//the rest of cents after mode 100 is the new amount of cents
} else {
dollar += dollars;//add new dollars
this.cents += cents;//add new cents
}
}
//add new money object to the current money object
public void add(Money other) {
this.dollar += other.dollar;//add new object of dollar to the current dollar
this.cents += other.cents;//add new object of cents to the current cents
}
//checking whether two money have the same amount
public boolean equals(Object o) {
Money other = (Money) o;//make a new object
//check whether the amount of two objects of dollars and cents are the same
if (this.dollar == other.dollar && this.cents == other.cents) {
return true;
} else {
return false;
}
}
//return the correct format of dollars and cents
public String toString() {
String price = "$" + dollar + "." + cents;//formatting dollars and cents
//when the first digit of cent is zero
if (cents == 0) {
return price + cents;//add another cent to have two decimal places
} else {
return price;//return the correct format of dollars and cents
}
}
}
/**
* Bill.java
*
*
*/
public class Bill {
//instance variables for bills
private Money amount;
private Date dueDate;
private Date paidDate;
String originator = null;
//Constructor that sets amount of money owed, due date, originator, and date paid
public Bill(Money amount, Date dueDate, String originator) {
this.amount = amount;
this.dueDate = dueDate;
this.originator = originator;
}
//Copy constructor
public Bill(Bill toCopy) {
this.amount = toCopy.amount;
this.dueDate = toCopy.dueDate;
this.originator = toCopy.originator;
this.paidDate = toCopy.paidDate;
}
//get the value of amount of money owed
public Money getAmount() {
return amount;
}
//get the date of due date
public Date getDueDate() {
return dueDate;
}
//get the name of the originator
public String getOriginator() {
return originator;
}
//check whether already paid or not
public boolean isPaid() {
if (paidDate != null) {
return true;
} else {
return false;
}
}
//set the date of paid date
public boolean setPaid(Date datePaid) {
//datePaid is after dueDate then return false and do nothing
if (this.dueDate.isAfter(datePaid)) {
return false;
}
//if not then update the date of paidDate and return true
else {
this.paidDate = datePaid;
return true;
}
}
//set the date of the due date
public boolean setDueDate(Date nextDate) {
//if not already paid resets the due date and returns true
if (isPaid() == false) {
dueDate = nextDate;
return true;
}
//if already paid then return true
else {
return false;
}
}
//change the amount of money owed
public boolean setAmount(Money amount) {
//if already paid then return false
if (isPaid() == true) {
return false;
}
//if not paid then update the amount of money owed and return true
else {
this.amount = amount;
return true;
}
}
//set the name of the originator
public void setOriginator(Bill b) {
this.originator = b.originator;
}
//reports the amount owed, due date, originator, if paid, and paid date
public String toString() {
return "Amount owed " + amount + " Due date:" + dueDate + " To:" + originator
+ " Paid? " + isPaid() + " Date paid:" + paidDate;
}
//check whether two bills' amount, dueDate, paidDate, and originator are the same
public boolean equals(Bill toCompare) {
Bill b = (Bill) toCompare;
if (this.amount == b.amount && this.dueDate == b.dueDate && this.paidDate == b.paidDate
&& this.originator == b.originator) {
return true;
}
else {
return false;
}
}
}
/**
* Date.java
*
*/
public class Date {
//instance variables for dates
private int year;
private int month;
private int day;
public Date paidDate;
//Constructor that sets month, day and year
public Date(int month, int day, int year) {
this.month = month;
this.day = day;
this.year = year;
}
//Copy constructor that copies month, day, and year
public Date(Date other) {
this.month = other.month;
this.day = other.month;
this.year = other.month;
}
//get the value of year
public int getYear() {
return this.year;
}
//get the value of month
public int getMonth() {
return this.month;
}
//get the value of day
public int getDay() {
return this.day;
}
//set the valid range for year
public void setYear(int year) {
//case when year is greater than or equal to 2014 and less than or equal to 2024
if (year >= 2014 && year <= 2024) {
this.year = year;//set the class year equal to the parameter year
} else {
System.out.println("invalid year");
}
}
//set the valid range for month
public void setMonth(int month) {
//case when month is greater than 0 and less than or equal to 12
if (month > 0 && month <= 12) {
this.month = month;//set the value of month as the parameter month
} else {
System.out.println("invalid month");
}
}
//set the valid range for day
public void setDay(int day) {
//case when day is greater than 0 and less than or equal to 31
if (day > 0 && day <= 31) {
this.day = day;//set the class day equal to the parameter day
} else {
System.out.println("invalid day");
}
}
//check whether the date is after the other date
public boolean isAfter(Date compareTo) {
//case when all year, month, and day values are the same
if (this.year < compareTo.year) {
return true;
} if(this.year == compareTo.year && this.month < compareTo.month) {
return true;
} if(this.year == compareTo.year && this.month == compareTo.month
&& this.day < compareTo.day) {
return true;
}else {
return false;
}
}
//check whether the two dates are the same
public boolean equals(Object date) {
Date d = (Date) date;//make an another object
if (this.day == d.day && this.month == d.month && this.year == d.year) {
return true;
} else {
return false;
}
}
//make a String to print the value of dates
public String toString() {
//invalid range for year
if (year < 2014 || year > 2024) {
return "invalid year";
}
//invalid range for month
else if (month < 1 || month > 12) {
return "invalid month";
}
//invalid range for day
else if (day < 1 || day > 31) {
return "invalid day";
}
//if month and day contain a single digit, add "0" in front of it
else if ((month >= 1 && month <= 9) && (day >= 1 && day <= 9)) {
return "0" + month + "/0" + day + "/" + year;
}
else if (month >= 1 && month <= 9) {
return "0" + month + "/" + day + "/" + year;
}
else if (day >= 1 && day <= 9) {
return month + "/" + "0" + day + "/" + year;
}
//if no single digit for month and day, then simply output as it is
else {
return month + "/" + day + "/" + year;
}
}
}
/**
* Date.java
*
*
*/
public class Date {
//instance variables for dates
private int year;
private int month;
private int day;
public Date paidDate;
//Constructor that sets month, day and year
public Date(int month, int day, int year) {
this.month = month;
this.day = day;
this.year = year;
}
//Copy constructor that copies month, day, and year
public Date(Date other) {
this.month = other.month;
this.day = other.month;
this.year = other.month;
}
//get the value of year
public int getYear() {
return this.year;
}
//get the value of month
public int getMonth() {
return this.month;
}
//get the value of day
public int getDay() {
return this.day;
}
//set the valid range for year
public void setYear(int year) {
//case when year is greater than or equal to 2014 and less than or equal to 2024
if (year >= 2014 && year <= 2024) {
this.year = year;//set the class year equal to the parameter year
} else {
System.out.println("invalid year");
}
}
//set the valid range for month
public void setMonth(int month) {
//case when month is greater than 0 and less than or equal to 12
if (month > 0 && month <= 12) {
this.month = month;//set the value of month as the parameter month
} else {
System.out.println("invalid month");
}
}
//set the valid range for day
public void setDay(int day) {
//case when day is greater than 0 and less than or equal to 31
if (day > 0 && day <= 31) {
this.day = day;//set the class day equal to the parameter day
} else {
System.out.println("invalid day");
}
}
//check whether the date is after the other date
public boolean isAfter(Date compareTo) {
//case when all year, month, and day values are the same
if (this.year < compareTo.year) {
return true;
} if(this.year == compareTo.year && this.month < compareTo.month) {
return true;
} if(this.year == compareTo.year && this.month == compareTo.month
&& this.day < compareTo.day) {
return true;
}else {
return false;
}
}
//check whether the two dates are the same
public boolean equals(Object date) {
Date d = (Date) date;//make an another object
if (this.day == d.day && this.month == d.month && this.year == d.year) {
return true;
} else {
return false;
}
}
//make a String to print the value of dates
public String toString() {
//invalid range for year
if (year < 2014 || year > 2024) {
return "invalid year";
}
//invalid range for month
else if (month < 1 || month > 12) {
return "invalid month";
}
//invalid range for day
else if (day < 1 || day > 31) {
return "invalid day";
}
//if month and day contain a single digit, add "0" in front of it
else if ((month >= 1 && month <= 9) && (day >= 1 && day <= 9)) {
return "0" + month + "/0" + day + "/" + year;
}
else if (month >= 1 && month <= 9) {
return "0" + month + "/" + day + "/" + year;
}
else if (day >= 1 && day <= 9) {
return month + "/" + "0" + day + "/" + year;
}
//if no single digit for month and day, then simply output as it is
else {
return month + "/" + day + "/" + year;
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
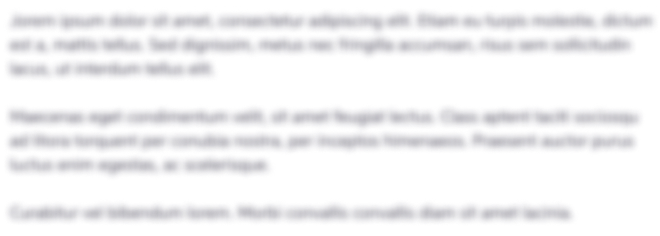
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started