Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In this assignment, you will write a C program for working with bank accounts. Your program will use pointers, structs, arrays, and null - terminated
In this assignment, you will write a C program for working with bank accounts. Your program will use pointers, structs, arrays, and nullterminated strings. You should have experience working with these things from CS You might need to review some of these topics, and look some things up but you should write this program without help from the teacher, tutors, IAs, other students, or anyone else. If you are not able to write this program on your own, without getting help from anyone, you are not ready to take this class and you should not stay in the class this term. As with every programming assignment in this class, make sure that your program compiles and runs on the class server. You won't get any credit for programs that don't run on the server. Part In a file named account.c implement all of the functions declared in the account.h file. It's important that you understand everything in account.h so if there is anything in it that you don't understand, look it up Make sure that your account.c file works with the following file mainc and produces the output shown below. All amounts and balances should be positive. Amounts for withdrawals should not be greater than the account balance, and amounts for transfers should not be greater than the balance of the source account. If any of these constraints are violated then the transaction should not be executed and the function should return a negative integer as its return value. The only function that should print anything is accountprint. Use a command line like this to compile your program: gcc o main mainc account.c That command line tells the compiler to compile mainc and account.c and to make an executable file named main account.h #include #define MAXNAMELEN typedef struct int id; int balance; char ownerMAXNAMELEN; USED FOR A STRING Account; int accountinitAccount acctp int id int balance, char owner; int accountdepositAccount acctp int amount; int accountwithdrawAccount acctp int amount; int accounttransferAccount fromp Account top int amount; int accountprintAccount acctp; Link to download file: account.hDownload account.h mainc #include #include "account.h int mainint argc, char argv Account a a; accountinit&a "Hermione Granger"; accountinit&a "Jack Swallow"; accountdeposit&a; accountwithdraw&a; accountwithdraw&a; accounttransfer&a &a; accountprint&a; accountprint&a; Link to download file: maincDownload mainc Output In the output below, the dollar sign represents the shell prompt and the name of the executable file is main $ main Hermione Granger $ Jack Swallow $ Part Write a program that reads account transactions from the standard input stream and then executes those transactions. The main source file that includes a function named main for this program should be mainc and your Makefile for this program should produce an executable named main Make an array of Account structs to keep track of the accounts. Define a constant using #define or the const keyword named MAXACCOUNTS that specifies the maximum number of accounts that can be created. Format of input lines Each line of input will have a transaction or a command as the first thing on the line. Items on each input line will be separated by one or more spaces or tabs Here is a list of account transactions and commands: new For a new transaction, your program should create or initialize an account. If the maximum number of accounts has already been created then your program should print an error message and not create an account. To create an account, initialize an Account struct in the array, using the accountinit function. Your program should assign an account number to the account and pass that number to accountinit. Input lines for new transactions will have the word new, followed by the initial balance of the new account, followed by the first and last names of the account owner. The initial balance will always be an integer. There will always be exactly two names for the account owner. Here is an example of an input line for a new account: new Hermione Granger The initial balance for the account is $ The first name is Hermione and the last name is Granger. Those two names should be combined into one nullterminated string which is passed to accountinit. deposit For a deposit transaction, your program should get the account number and the deposit amount from the input line, use the account number to get the address of the correct Account struct, and then call accountdeposit. Example: deposit withdraw For a withdraw transaction, your program should get the account number and the withdrawal amou
In this assignment, you will write a C program for working with bank accounts. Your program will use pointers, structs, arrays, and nullterminated strings. You should have experience working with these things from CS
You might need to review some of these topics, and look some things up but you should write this program without help from the teacher, tutors, IAs, other students, or anyone else. If you are not able to write this program on your own, without getting help from anyone, you are not ready to take this class and you should not stay in the class this term.
As with every programming assignment in this class, make sure that your program compiles and runs on the class server. You won't get any credit for programs that don't run on the server.
Part
In a file named account.c implement all of the functions declared in the account.h file. It's important that you understand everything in account.h so if there is anything in it that you don't understand, look it up Make sure that your account.c file works with the following file mainc and produces the output shown below.
All amounts and balances should be positive. Amounts for withdrawals should not be greater than the account balance, and amounts for transfers should not be greater than the balance of the source account. If any of these constraints are violated then the transaction should not be executed and the function should return a negative integer as its return value.
The only function that should print anything is accountprint.
Use a command line like this to compile your program:
gcc o main mainc account.c
That command line tells the compiler to compile mainc and account.c and to make an executable file named main
account.h
#include
#define MAXNAMELEN
typedef struct
int id;
int balance;
char ownerMAXNAMELEN; USED FOR A STRING
Account;
int accountinitAccount acctp int id int balance, char owner;
int accountdepositAccount acctp int amount;
int accountwithdrawAccount acctp int amount;
int accounttransferAccount fromp Account top int amount;
int accountprintAccount acctp;
Link to download file: account.hDownload account.h
mainc
#include
#include "account.h
int mainint argc, char argv
Account a a;
accountinit&a "Hermione Granger";
accountinit&a "Jack Swallow";
accountdeposit&a;
accountwithdraw&a;
accountwithdraw&a;
accounttransfer&a &a;
accountprint&a;
accountprint&a;
Link to download file: maincDownload mainc
Output
In the output below, the dollar sign represents the shell prompt and the name of the executable file is main
$ main
Hermione Granger $
Jack Swallow $
Part
Write a program that reads account transactions from the standard input stream and then executes those transactions. The main source file that includes a function named main for this program should be mainc and your Makefile for this program should produce an executable named main
Make an array of Account structs to keep track of the accounts. Define a constant using #define or the const keyword named MAXACCOUNTS that specifies the maximum number of accounts that can be created.
Format of input lines
Each line of input will have a transaction or a command as the first thing on the line. Items on each input line will be separated by one or more spaces or tabs Here is a list of account transactions and commands:
new
For a new transaction, your program should create or initialize an account. If the maximum number of accounts has already been created then your program should print an error message and not create an account. To create an account, initialize an Account struct in the array, using the accountinit function. Your program should assign an account number to the account and pass that number to accountinit.
Input lines for new transactions will have the word new, followed by the initial balance of the new account, followed by the first and last names of the account owner. The initial balance will always be an integer. There will always be exactly two names for the account owner.
Here is an example of an input line for a new account:
new Hermione Granger
The initial balance for the account is $ The first name is Hermione and the last name is Granger. Those two names should be combined into one nullterminated string which is passed to accountinit.
deposit
For a deposit transaction, your program should get the account number and the deposit amount from the input line, use the account number to get the address of the correct Account struct, and then call accountdeposit.
Example:
deposit
withdraw
For a withdraw transaction, your program should get the account number and the withdrawal amou
Step by Step Solution
There are 3 Steps involved in it
Step: 1
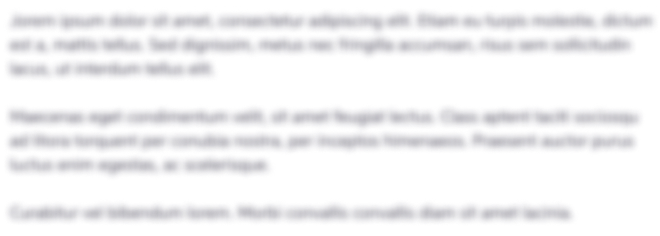
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started