Question
In this introductory major assignment, you will write a complete C program that will implement a set of operations supported by a menu that will
In this introductory major assignment, you will write a complete C program that will implement a set of operations supported by a menu that will prompt for the operation (Power of 2, Reverse Bits, Replace Bit Position from Mask, and Palindrome) along with a positive integer less than 2 billion, and then perform that operation on that integer (based on possible additional input) to produce the result. More details about the operations are found below. The twist in this assignment is that each operation must be performed using bitwise operators rather than the traditional operations. That said, relational operators and the like can be used in branching and loops, but data manipulation must be done using bitwise operators. This project will be organized in a header file called major1.h, a source code file called major1.c, which will contain the main() function, and four individual source code files called power.c, reverse.c, replace.c, and palindrome.c that will contain the function definition for their respective arithmetic operations. The entire group will be responsible in general for non-operation specific functionality in the major1.h and major1.c source code files while each individual group member is responsible for his/her own functional operation in the appropriate files (including the function declaration in the header file and the function call inside the main() function). In particular, you are expected to have the following functionality for each file:
major1.h
This file is the overall header file for the project and will contain any preprocessor directives, such as include and define directives, and function prototypes. While the include directives are general for the team, each member is expected to add their own function prototype (i.e., function declaration) for the operation that he/she is responsible for. major1.c
This is the code file with the main() function that will do the following: (1) display the menu, (2) read in the users response for the menu selection, (3) prompt for and read in a positive integer less than two billion, and then, based on the menu selection, (4) call the appropriate function call for the specified operation, passing the integer operand as a parameter to that function. This functionality will be contained in a loop that will continue to iterate until the user selects the option to end the program. If the user enters a valid outside of the 1 5 range, you will print a meaningful error message and re-display the menu. Additionally, you will continue to prompt for and read in the integer operand until the user enters an acceptable value (no error message is needed here). While the code to display the menu as well as prompt for and read in the integer operand are considered to be part of the group component, each group member is expected to add the function call for the operation he/she is responsible for. power.c This code will contain a single function that accepts a single positive integer less than two billion (and the include directive to your header file) to perform the following functionality: determine if the passed-in integer parameter is a power of two (i.e., is there some integral value N for which the positive integer 2N less than two billion exists, such as 32, which is 25 ) and if it is not a power of two, calculate the next integer higher than the passed-in integer parameter that is a power of two. For example, if the user enters the positive integer 12 (which is not a power of two), the next higher integer that is a power of two is 16. The operations to determine whether or not the positive integer is a power of two, plus the calculation of the next higher integer that is a power of two, must be done using bitwise operators.
reverse.c
This code will contain a single function that accepts a single positive integer less than two billion (and the include directive to your header file) to perform the following functionality: reverse the bits (all 32 of them) and then print out the decimal value of the new integer (i.e., the one with the bits reversed). For example, if the user enters the positive integer 2 (which is 000010), this function would reverse the digits to 010000, which is 1073741824. The operations to reverse the bits must be done using bitwise operators.
replace.c
This code will contain a single function that accepts a single positive integer less than two billion (and the include directive to your header file) to perform the following functionality: (1) prompt for and read in a positive integer mask less than three billion and continue to prompt for and read in the positive integer mask until the user enters and acceptable value (no error message is needed here), (2) prompt for and read in the bit replacement position from the mask and continue to prompt for and read in the bit replacement position from the mask until the user enters an acceptable value (no error message is needed here, but note that since we are working with 32-bit unsigned integers, the value should be between 0 and 31, inclusively), and finally (3) replace the single bit in the original positive integer less than two billion passed to the function with the single bit from the positive integer mask specified by the user (i.e., in the bit replacement position from the mask). For example, if the user initially enters 7 (in binary, 0000111) as the positive integer less than two billion, then enters 8 (in binary, 0001000) for the positive integer mask and 3 for the bit replacement position, you will replace the third bit from the positive integer 7 (a 0) with the third bit from the positive integer mask (a 1), resulting in the new value 15 (in binary, 0001111). The operations to replace the bit must be done using bitwise operators. Additionally, no loops may be used (except in error checking when prompting for and reading in the positive integer mask and bit replacement position from the mask) to achieve this functionality.
SAMPLE OUTPUT (user input shown in bold):
$ ./binops
Enter the menu option for the operation to perform:
(1) POWER OF 2
(2) REVERSE BITS (3)
REPLACE BIT POSITION FROM MASK
(4) PALINDROME
(5) EXIT
--> 0
Error: Invalid option. Please try again.
Enter the menu option for the operation to perform:
(1) POWER OF 2
(2) REVERSE BITS
(3) REPLACE BIT POSITION FROM MASK
(4) PALINDROME
(5) EXIT
--> 6
Error: Invalid option. Please try again.
Enter the menu option for the operation to perform:
(1) POWER OF 2
(2) REVERSE BITS
(3) REPLACE BIT POSITION FROM MASK
(4) PALINDROME
(5) EXIT
--> 1
Enter a positive integer less than 2 billion: 0
Enter a positive integer less than 2 billion: 2000000000
Enter a positive integer less than 2 billion: 32
32 is a power of 2
Enter the menu option for the operation to perform:
(1) POWER OF 2
(2) REVERSE BITS
(3) REPLACE BIT POSITION FROM MASK
(4) PALINDROME
(5) EXIT
--> 1
Enter a positive integer less than 2 billion: 72346
72346 is not a power of 2
Next higher integer that is power of 2 is: 131072
Enter the menu option for the operation to perform:
(1) POWER OF 2
(2) REVERSE BITS
(3) REPLACE BIT POSITION FROM MASK
(4) PALINDROME
(5) EXIT
--> 2
Enter a positive integer less than 2 billion: 0
Enter a positive integer less than 2 billion: 2000000000
Enter a positive integer less than 2 billion: 2
2 with bits reversed is 1073741824
Enter the menu option for the operation to perform:
(1) POWER OF 2
(2) REVERSE BITS
(3) REPLACE BIT POSITION FROM MASK
(4) PALINDROME
(5) EXIT
--> 2
Enter a positive integer less than 2 billion: 1073741824
1073741824 with bits reversed is 2
Enter the menu option for the operation to perform:
(1) POWER OF 2
(2) REVERSE BITS
(3) REPLACE BIT POSITION FROM MASK
(4) PALINDROME
(5) EXIT
--> 2
Enter a positive integer less than 2 billion: 237834
237834 with bits reversed is 1350942720
Enter the menu option for the operation to perform:
(1) POWER OF 2
(2) REVERSE BITS
(3) REPLACE BIT POSITION FROM MASK
(4) PALINDROME
(5) EXIT
--> 3
Enter a positive integer less than 2 billion: 0
Enter a positive integer less than 2 billion: 2000000000
Enter a positive integer less than 2 billion: 7
Enter a positive integer mask up to 3 billion: 0
Enter a positive integer mask up to 3 billion: 3000000000
Enter a positive integer mask up to 3 billion: 8
Enter the bit replacement position from mask (0-indexed): -1
Enter the bit replacement position from mask (0-indexed): 32
Enter the bit replacement position from mask (0-indexed): 3
New integer with bit 3 from mask 8 is 15
Enter the menu option for the operation to perform:
(1) POWER OF 2
(2) REVERSE BITS
(3) REPLACE BIT POSITION FROM MASK
(4) PALINDROME
(5) EXIT
--> 3
Enter a positive integer less than 2 billion: 238475
Enter a positive integer mask up to 3 billion: 2983434345
Enter the bit replacement position from mask (0-indexed): 18
New integer with bit 18 from mask 2983434345 is 238475
Enter the menu option for the operation to perform:
(1) POWER OF 2
(2) REVERSE BITS
(3) REPLACE BIT POSITION FROM MASK
(4) PALINDROME
(5) EXIT
6 of 7
--> 3
Enter a positive integer less than 2 billion: 238475
Enter a positive integer mask up to 3 billion: 2983434345
Enter the bit replacement position from mask (0-indexed): 22
New integer with bit 22 from mask 2983434345 is 4432779
Enter the menu option for the operation to perform:
(1) POWER OF 2
(2) REVERSE BITS
(3) REPLACE BIT POSITION FROM MASK
(4) PALINDROME
(5) EXIT
--> 4
Enter a positive integer less than 2 billion: 0
Enter a positive integer less than 2 billion: 2000000000
Enter a positive integer less than 2 billion: 1073741826
The binary representation is: 01000000000000000000000000000010
1073741826 is a palindrome
Enter the menu option for the operation to perform:
(1) POWER OF 2
(2) REVERSE BITS
(3) REPLACE BIT POSITION FROM MASK
(4) PALINDROME
(5) EXIT
--> 4
Enter a positive integer less than 2 billion: 298554990
The binary representation is: 00010001110010111001011001101110
298554990 is not a palindrome
Enter the menu option for the operation to perform:
(1) POWER OF 2
(2) REVERSE BITS
(3) REPLACE BIT POSITION FROM MASK
(4) PALINDROME
(5) EXIT
--> 5
Program terminating. Goodbye.
Program terminating. Goodbye.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
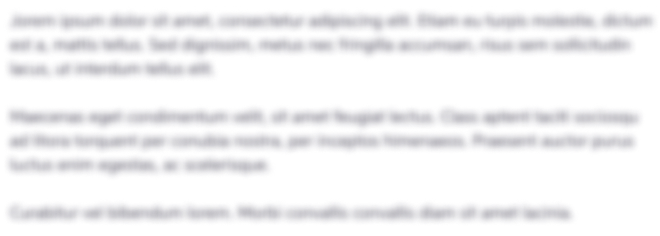
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started