Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In this lab we will be creating a C++ class, and an object of that class. Classes can be used to represent anything: cars, gradebooks,
Step by Step Solution
There are 3 Steps involved in it
Step: 1
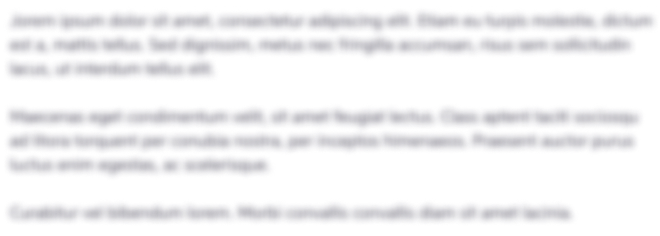
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started