Question
In this lab we will be working with the Numbers class. Start by downloading the files in the numbers directory. You will see that it
In this lab we will be working with the "Numbers" class. Start by downloading the files in the numbers directory. You will see that it contains the class definition in numbers.h, the implementation of class methods in numbers.cpp, and a small main in numbers.cpp program that calls these methods to test their operation.
Create a NetBeans "C++ Application" project called "Numbers" with an automatically generated "main.cpp" file. Create a "numbers" class, and then replace the NetBeans versions of numbers.h, numbers.cpp and main.cpp with the files you downloaded above. When you compile and run the project, you should be prompted for an input file.
Download the file numbers.txt and save this in the same directory as your NetBeans code. If you look inside this file, you will see it has 100 two digit numbers (which are actually the first 200 digits of PI). Now when you run your program, enter "numbers.txt" as the name of the input file.
Copy and paste your program output into the text box below:
In probability theory and statistics, the variance and standard deviation are measures of how "dispersed" or "spread out" a set of numbers are. The standard formula for calculating variance is: where the mean is given by
The standard deviation is simply the square root of the variance.
If you look at the Numbers class definition you will see that it has methods for calculating the min, max, and mean values of the array of numbers. Your task in this section is to add two new methods called "findVariance" and "findStandardDeviation" that calculate the variance and standard deviation of the array of numbers using the formulas above.
Start by adding two methods to the class definition, and two empty method implementations. Then add calls to these two methods to the main program and print out the variance and standard deviation. Finally add the body of the two methods and run the program again.
Copy and paste your findVariance and findStandardDeviation methods below:
Copy and paste your program output into the text box below:
As you now know, the Numbers class reads and manipulates an array of integers. In this section, we will use "typedef" to generalize the Numbers class so it can work with any numeric data type. Start by making a backup copy of numbers.cpp so you do not lose the original file.
Add the line "typedef int DataType;" above the Numbers class definition and go through the class definition and replace all of the "int" with "DataType" where the variable or return type is associated with the Data itself. Do NOT change index variables or counters. You should end up with seven occurrences of "DataType" in the class definition.
Next go through all of the method implementations and the main program and replace all of the "int" to "DataType" using the same approach. Remember, we only want to change variables associated with the Data array. When you compile and run your program it should produce exactly the same results as before.
Finally, edit the typedef line to be "typedef float DataType;" and compile and run the program. You should see that the mean, variance and standard deviation are all output as float values. Congratulations, you just generalized your first C++ class to support multiple data types.
Copy and paste your typedef line and class definition below:
Copy and paste your program output into the text box below:
The typedef approach is great because we only have to change one line to change a class from using "int" data to using "float" data. The problem is that we can not easily have a main program that works with BOTH kinds of classes at the same time. This is why templates were added to C++. By using a template class, we can supply the data type we want to use when we declare the objects in the main program.
Start by making a backup copy of the typedef version of "numbers.cpp" so you do not lose this work. Next, edit the class definition to remove the typedef line and add the following "template
Next, copy/paste this line just before each of your method definitions to tell the compiler that these methods are templated. Then change the "Numbers::" to be "Numbers
templateint Numbers ::getCount() { return Count; }
Next, replace the original main program with the following code and compile and run your program. If you look at this code, you will see that we used "Numbers
// For templates we MUST compile the numbers.h and numbers.cpp code // at the same time as main.cpp in order for the compiler to resolve // what data types you are using in Numbers objects. This is done // by including "numbers.cpp" below. // When compiling on the Linux command line use g++ -Wall main.cpp // When compiling using an IDE, you may need to rename "numbers.cpp" // to "numbers.cpp.txt" and change the include line below accordingly // If you do not rename the file, it may be compiled twice causing // a large number of confusing errors. #include "numbers.cpp" int main() { string filename; cout > filename; // Process int numbers Numbersnum; num.readFile(filename); cout num2; num2.readFile(filename); cout Finally, edit your program and see what happens when you use the following data types (short, long, double, char, string) when creating Numbers objects. Which work fine? Which have problems?
Describe your experiments with your Numbers class below:
Copy and paste your final "numbers.cpp" file below:
main.cpp
#include "numbers.h" //---------------------------------------------- int main() { Numbers num; string filename; cout > filename; num.readFile(filename); coutnumbers.cpp
#include "numbers.h" //---------------------------------------------- Numbers::Numbers() { // Initialize variables Count = 0; for (int i = 0; i > num; while (!din.eof() && Count > num; } din.close(); } //---------------------------------------------- int Numbers::getCount() { return Count; } //---------------------------------------------- int Numbers::getValue(int index) { // Return specified value if ((index >= 0) && (index Data[index]) min = Data[index]; return min; } //---------------------------------------------- int Numbers::findMax() { // Search array for max int max = Data[0]; for (int index = 0; index 0) return total / Count; else return 0; }numbers.h
#ifndef NUMBERS_H #define NUMBERS_H //---------------------------------------------- // Purpose: Numbers class with basic operations // Author: John Gauch //---------------------------------------------- #include#include using namespace std; //---------------------------------------------- class Numbers { public: // Constructors Numbers(); ~Numbers(); // Methods void readFile(string filename); int getCount(); int getValue(int index); int findMin(); int findMax(); int findMean(); private: static const int SIZE = 100; int Count; int Data[SIZE]; }; #endif numbers.txt
14 15 92 65 35 89 79 32 38 46 26 43 38 32 79 50 28 84 19 71 69 39 93 75 10 58 20 97 49 44 59 23 07 81 64 06 28 62 08 99 86 28 03 48 25 34 21 17 06 79 82 14 80 86 51 32 82 30 66 47 09 38 44 60 95 50 58 22 31 72 53 59 40 81 28 48 11 17 45 02 84 10 27 01 93 85 21 10 55 59 64 46 22 94 89 54 93 03 81 962=N1i=1N(xi)2 =N1i=1Nxi
Step by Step Solution
There are 3 Steps involved in it
Step: 1
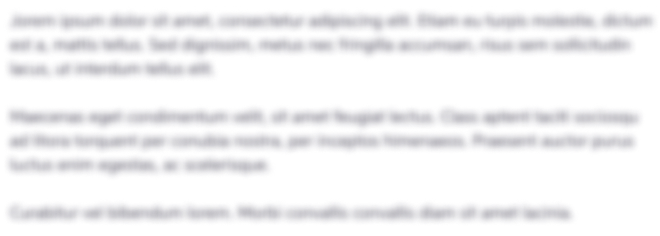
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started