Question
In this lab, we will practice: using the modulo operator % to get the rightmost digits of a number using the integer division // to
In this lab, we will practice:
using the modulo operator % to get the rightmost digits of a number
using the integer division // to get the desired leftmost digits by "shifting the number right"
developing an algorithm and writing pseudocode to outline the steps of the solution
using string formatting (f-strings) to produce a string with the requested format
writing a function with one input parameter
writing the main program and calling the function with a correct argument
producing the requested output as part of the main program
Instructions
Write a function convert_phone_number() that takes an integer representing a 10-digit phone number. The function should return a string containing the phone number in the following format:
(NNN) NNN-NNNN
In your main program, get the input from the user, convert it into an integer, and then call the function to produce the requested output.
Assumptions / Simplifications
For simplicity, assume the input number always has 10 digits and starts with a non-zero digit. So 0119998888 is not allowed. You can also assume that none of the three pieces of the phone number would start with a 0. For example, you do not need to worry about 512 014 2012 or 512 919 0234 as inputs.
Example
If the input into the function is an integer:
8005551212
the output of the function is a string that contains:
(800) 555-1212
Algorithm / Pseudocode
Look at the example above and write down the steps for how to solve this problem.
You might have something like:
Get the leftmost 3 digits and store them in an area_code variable
Get the rightmost 4 digits and store them in a variable
Get the middle 3 digits by
What should you do once you have the first 2 steps done? Work it out before you begin coding it.
Hints
Use the modulo operator % to get the desired rightmost digits.
For example: The rightmost 2 digits of 572 can be obtained using 572 % 100, which is 72.
For example: The last digit of 572 can be obtained using 572 % 10, which is 2.
Use the integer division // to get the desired leftmost digits by "shifting the number right" by the desired amount.
Shifting 572 right by 2 digits is done by 572 // 100, which yields 5. (Recall that the integer division discards the fraction).
Shifting 572 right by 1 digit is done by 572 // 10, which yields 57.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
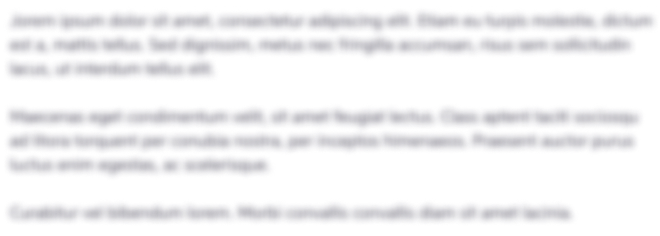
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started