Question
In this lab, you will be writing a program to solve mazes that are provided as input files. This lab will provide practice on 2D
In this lab, you will be writing a program to solve mazes that are provided as input files.
This lab will provide practice on 2D arrays, as well as classes and objects
Below is an example of a maze, as provided in an input file:
H H H H H H H H H H H H H H X H H X X X X X X H H D H H H X H H H H H H X X H H H H H H X H X H H X X X X X X H H X H H H H H H
The Hs in the file represent walls in the maze.
The Xs in the file represent open spaces that can be traversed
Note that there is one opening in the wall, which will always be located *somewhere* at the bottom of the maze.
You will need to locate this opening to determine where to begin
It will be the only X in the bottom row of the maze.
There is also a single D in each maze, which represents the cheese. It is the goal of your program to find a path through the maze to this cheese.
You will work with a Rat class, where the Rat object will move through the mazes, always using the same algorithm:
If the rat can move up, it will move up
If the rat can't move up, but can move left, it will move left.
If the rat can't move up or left, it will move right
If the rat can't move up, left or right, it will move down
As the rat traverses the maze, it leaves a * everywhere it has been (rat droppings!) so that it is easy to see the path it takes through the maze.
When it locates the cheese, you should display the message "Munch, munch, munch!" and then print out the maze, including the rat's path
The final location of the rat should also be displayed using a & (rat is sitting down after the exploring the maze)
The program should begin by prompting the user for the name of an input file, and then reading the contents of the input file into a 2D array (required).
Note that you are required to use a 2D array to store the maze and are not permitted to use ArrayLists.
Note that the first line of each input file begins with the a number that indicates the length of the rows and columns in your 2D array.
Below are 3 input files with sample output.
Your program should work with these files, and any other similar maze files the instructor chooses to run with your submitted lab.
Input file: maze1.txt:
8 H H H H H H H H H H H H H H X H H X X X X X X H H D H H H X H H H H H H X X H H H H H H X H X H H X X X X X X H H X H H H H H H
Sample Output for maze1.txt:
Welcome to the Maze Runner! Please enter the name of the file: maze1.txt Munch! Munch! Munch! Maze: H H H H H H H H H H H H H H X H H * * * * * X H H & H H H * H H H H H H * * H H H H H H * H X H H * * * * X X H H * H H H H H H Input file: maze2.txt:
15 H H H H H H H H H H H H H H H H X X X H H X X X X D H X X H H H H X H H X H H H H H H X H H H H X H X X X X X H H X X H H X X X H X H H H H X H H X H H X H X X X X X X X X H X X H H H H X H H H X H H H H H X H H X X X H X H X X X X X H X H H X H H H H H X H H H H H X H H X H X H X X X X X H H H X H H X X X H X H H H X H X X X H H H H X H X H X H X H X H X H H H H X H X X X X X X X H X H H X X X H H H X H X H X H X H H H H H H H H H H X H H H H H
Sample Output for maze2.txt:
Welcome to the Maze Runner! Please enter the name of the file: maze2.txt Munch! Munch! Munch! Maze: H H H H H H H H H H H H H H H H X X X H H * * * * & H X X H H H H X H H * H H H H H H X H H H H X H * * X X X H H X X H H X X X H * H H H H X H H X H H X H X X * * * X X X H X X H H H H X H H H * H H H H H X H H X X X H X H * X X X X H X H H X H H H H H * H H H H H X H H X H X H X X * * * H H H X H H X X X H X H H H * H X X X H H H H X H X H X H * H X H X H H H H X H X X X X * X X H X H H X X X H H H X H * H X H X H H H H H H H H H H * H H H H H Input file: maze3.txt:
7 H H H H H H H H X X X X X H H X H H H X H H X X X H X H H X H X H D H H X H X H H H H H H X H H H
Sample Output for maze3.txt:
Welcome to the Maze Runner! Please enter the name of the file: maze3.txt Munch! Munch! Munch! Maze: H H H H H H H H * * * * * H H * H H H * H H * * * H * H H X H * H & H H X H * H H H H H H * H H H
Required Starter Code
Your starter code for this assignment will be in two separate files.
You will have one file called Rat.java, a class which defines the behavior of the Rat who will traverse your maze.
You must complete the implementation of the methods who signatures are provided.
The other file must be named Maze.java and it will contain a main method to define the behavior of your maze program.
Place both files in the same Lab3 project folder
Rat.java Starter Code:
public class Rat { private int posX; private int posY; /** * Sets the x (column) location of the rat * @param x the x position of the rat in the x-y plane */ public void setXPos(int x) { posX = x; } /** * Sets the y (row) location of the rat * @param y the y position of the rat in the x-y plane */ public void setYPos(int y) {
//fill in here
} /** * Returns the x position of the rat * @return the x position of the rat in the x-y plane */ public int getXPos() { return posX; } /** * Returns the y position of the rat * @return the y position of the rat in the x-y plane */ public int getYPos() {
//fill in here
} /** * Moves the rat one space to the left * by subtracting one from its x position */ public void moveLeft() {
//fill in here
} /** * Moves the rat one space to the right * by adding one to its x position */ public void moveRight() {
//fill in here
} /** * Moves the rat one space down * by adding one to its y position */ public void moveDown() {
//fill in here
} /** * Moves the rat one space up * by subtracting one from its y position */ public void moveUp() { fill in here } }
A Note on Positioning the Rat:
The upper left corner of the maze has an (x,y) position of (0,0).
The upper right corner of the maze has an (x,y) position of (length - 1, 0)
The bottom left corner of the maze has an (x,y) position of (0, length - 1)
The bottom right corner of the maze has an (x,y) position of (length - 1, length - 1)
Therefore in a 5X5 maze here would be the coordinate positions of the rat at each possible location:
(0,0) | (1,0) | (2,0) | (3,0) | (4,0) |
(0,1) | (1,1) | (2,1) | (3,1) | (4,1) |
(0,2) | (1,2) | (2,2) | (3,2) | (4,2) |
(0,3) | (1,3) | (2,3) | (3,3) | (4,3) |
(0,4) | (1,4) | (2,4) | (3,4) | (4,4) |
Maze.java Starter Code:
import java.io.PrintWriter; import java.io.File; import java.io.IOException; import java.util.Scanner; public class Maze { public static void main(String[] args) throws IOException{ String maze[][];
//declare your Rat object here
Scanner input = new Scanner(System.in); System.out.println("Welcome to the Maze Runner! ");
//rest of main method
input.close();
} //end of main
/** * Prints out the 2D maze to the console * @param maze the 2D array representing the maze */ public static void printMaze(String maze[][]) { System.out.println(" Maze:");
//rest of printMaze method
} //end of printMaze
} //end of class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
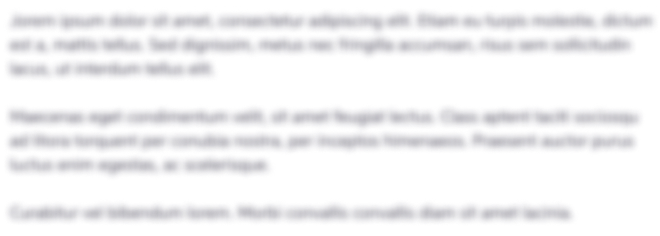
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started