Question
In this lab, you will work with two java files: Pokemon.java - defines the Pokemon class PokemonField.java - contains main() method, where you may create
In this lab, you will work with two java files:
-
Pokemon.java - defines the Pokemon class
-
PokemonField.java - contains main() method, where you may create objects of the Pokemon class.
Note: In the Pokemon game, hp is a term that means hit points (or life). This value decreases when the Pokemon is attacked. When this value reaches 0, the Pokemon loses the battle. The field maxHp refers to the number of hit points the Pokemon has at the beginning of the battle.The field hitPoints refers to the current amount of hitPoints remaining for the Pokemon. At the beginning of the battle, these two values will be the same, but hitPoints will decrease over time when the Pokemon is attacked. The field attackPoints refers to the amount of damage that the Pokemon creates when it attacks another Pokemon.
-
Complete the Pokemon class with the following specifications:
(1) add the following four private fields
* String name * int maxHp * int hitPoints * int attackPoints
(2) add a constructor, which takes three parameters, the first one is used to initialize name, the second one is used to initialize both maxHP and hitPoints, and the third one is used to initialize attackPoints.
(3) add public methods (mutators): setHitPoints, setAttackPoints
(4) add public methods (accessors): getName, getHitPoints, getAttackPoints
-
Complete the fight method in PokemonField.java
import java.util.Random; public class PokemonField { public static void main(String[] args) { /*FIXME(1) Create an object of Pokemon class, p1, with the following arguments, "Pikachu", 10, 2 */ /*FIXME(2) Create another object of Pokemon class, p2, with the following arguments, "Absol", 10, 1 */ p1.printStat(); p2.printStat(); System.out.println(); Random random = new Random(); fight(random, p1, p2); p1.printStat(); p2.printStat(); } /*In this method, two Pokemon objects fight until one of them get a 0 hitPoints. */ public static void fight(Random random, Pokemon p1, Pokemon p2) { double prob = (double)p1.getAttackPoints() / (p1.getAttackPoints() + p2.getAttackPoints()); while(p1.getHitPoints() > 0 && p2.getHitPoints() > 0) { if(random.nextDouble() < prob) { /*FIXME(3) p1 won, deduct p2's hitPoints by the amount of p1's attackPoints. The minimum hitPoints for any Pokemon is 0, so it cannot be set to a negative number.*/ } else{ /*FIXME(4) p2 won, deduct p1's hitPoints by the amount of p2's attackPoints. The minimum hitPoints for any Pokemon is 0, so it cannot be set to a negative number.*/ } } if(p1.getHitPoints() > 0) System.out.println(p1.getName() + " won!"); else System.out.println(p2.getName() + " won!"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
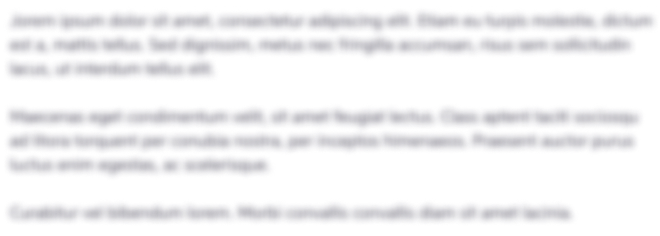
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started