Question
In this part, you will implement a resizable and generic Queue using an array. The API you must implement is described below. Do not change
In this part, you will implement a resizable and generic Queue using an array. The API you must implement is described below. Do not change the names or types of any of the methods below!
NOTE: You should implement the Queue using the loop-around method described during class. If you do not, you will likely not pass the test cases!
Queue
Queue | constructor; creates and empty Queue with initial capacity of 1 |
Queue(int n) | constructor; creates an empty Queue with initial capacity of n |
void enqueue(Item item) | 1) adds new item to the back of the Queue; 2) double the array capacity if the Queue becomes full |
Item dequeue() | 1) removes and returns front item from the Queue; 2) throws EmptyQueueException if the Queue is empty; 3) reduce the array capacity by half if the size of the Queue falls below 14 full but do not make the size below the initial capacity |
boolean isEmpty() | return true if the Queue is empty, false otherwise |
int size() | return the size of the Queue (i.e. the number of elements currently in the Queue) |
Item peek() | 1) return but do not remove the front item in the Queue; 2) throws an EmptyQueueException if the Queue is empty |
Item[] getArray()* | return the underlying array that contains the Queue |
Note: When you resize, the front of the queue should be reset to index 0. You should not reset it when the size goes to 0.
The Queue should use a loop-around method in order to reuse open space. Here is an example of how that works. Assume the initial capacity of the array is 5. The F stands for front and the B stands for back.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
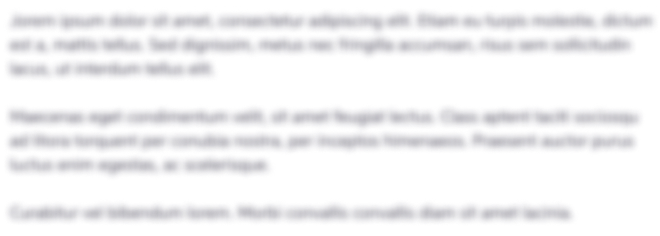
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started