Question
In this problem set, you will practice how to formulate optimization problems and solve them with Pyomo. The total points of this problem set is
In this problem set, you will practice how to formulate optimization problems and solve them with Pyomo. The total points of this problem set is 60 points. You need to show all your working and reasoning to obtain full credit.
Instructions:
Please add your answers in the provided cells. Double click on them so that you can edit. If you insert additional cells for testing during development, please delete them before submitting your homework.
There are provided cells of Python code that can help you check your answers. For example:
print('*** Solution *** ') print('x1:', value(model.x1)) print('x2:', value(model.x2))
You shouldn't need to modify those cells.
If you have code that prints a lot of text, please remove them before submitting because large amounts of printed outputs could obscure your answers.
Question 1 (12 points)
An oil company must determine how many barrels of oil to extract during each of the next two years. If the company extracts ????1�1 million barrels during year 1, each barrel can be sold for 80−????180−�1 dollars. If the company extracts ????2�2 million barrels during year 2, each barrel can be sold for 85−????285−�2 dollars. The cost of extracting ????1�1 million barrels in year 1 is 2????212�12 million dollars, and the cost of extracting ????2�2 million barrels in year 2 is 3????223�22 million dollars. A total of 20 million barrels of oil are available, and at most $250 million can be spent on extraction. The company wants to maximize its profit (revenue - cost) for the next two years.
Part (a) Formulate the optimization problem (6 pts)
As a first step, formulate an optimization problem from the description.
Your formulation should clearly specify:
- Decision variables
- Objective function
- Constraints
Note that you can write math expressions with LaTex in Jupyter Notebook by wrapping them with the dollar sign($).
For example: ∑????????=0????2????+????????????????−????????????∑�=0���2+����−���
Your answer here:
Part (b) Solve for the optimal solution using Pyomo (6 pts)
You want to first define the model with Pyomo and then find the optimal solution with the IPOPT solver.
Use the skeleton code to get you started.
In [ ]:
from pyomo.environ import *from pyomo.opt import SolverFactorysolver = SolverFactory('ipopt')
In [ ]:
model = ConcreteModel()# Define the decision variables:model.x1 = '''your code here'''model.x2 = '''your code here''''''add your constraints and objective'''solver.solve(model)
In [ ]:
print('*** Solution *** ')print('x1:', value(model.x1))print('x2:', value(model.x2))
Question 2 (26 points)
A company has the following historical data on the number of ad exposures and the corresponding number of units sold of one of its products:
Ads | 0 | 20 | 40 | 60 | 80 | 100 | 120 | 140 | 160 | 180 | 200 | 220 | 240 | 260 | 280 | 300 | 320 | 340 | 360 | 380 | 400 |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Units | 3 | 18 | 34 | 46 | 56 | 65 | 72 | 78 | 82 | 85 | 90 | 90 | 93 | 94 | 95 | 101 | 98 | 100 | 98 | 101 | 105 |
Part (a) Power fit (12 pts)
Formulate a nonlinear optimization model to find the parameters of a function of the form ????(????)=????????????�(�)=��� to model the number of units sold (y) for its product as a function of ad exposures (x). Solve for the optimal solution using Pyomo.
Note that it's common to perform similar "curve-fitting" tasks, for example, with Excel.
Formulate the optimization problem (6 pts)
As a first step, formulate an optimization problem from the description.
Your formulation should clearly specify:
- Decision variables
- Objective function
- Constraints
Your answer here:
Solve for the optimal solution using Pyomo (6 pts)
You want to first define the model with Pyomo and then find the optimal solution with the IPOPT solver.
Use the skeleton code to get you started.
In [ ]:
ads = [i*20 for i in range(21)]units = [3,18,34,46,56,65,72,78,82,85,90,90,93,94,95,101,98,100,98,101,105]
In [ ]:
model = '''Your code here'''model.a = '''Your code here'''model.b = '''Your code here'''def objective(model): '''Your code here'''model.obj = Objective(rule=objective, sense='''Your code here''')solver.solve(model)
In [ ]:
print('*** Solution *** :')print('a:', value(model.a))print('b:', value(model.b))print('sum of squared error:', model.obj())
Part (b) Exponential fit (12 pts)
Formulate a nonlinear optimization model to find the parameters of a function of the form ????(????)=????(1−????−????????)�(�)=�(1−�−��) to model the number of units sold (y) for its product as a function of ad exposures (x). Solve for the optimal solution using Pyomo.
Formulate the optimization problem (6 pts)
As a first step, formulate an optimization problem from the description.
Your formulation should clearly specify:
- Decision variables
- Objective function
- Constraints
Your answer here:
Solve for the optimal solution using Pyomo (6 pts)
You want to first define the model with Pyomo and then find the optimal solution with the IPOPT solver.
Use the skeleton code to get you started.
In [ ]:
model = '''Your code here'''model.a = '''Your code here'''model.b = '''Your code here'''def objective(model): '''Your code here'''model.obj = Objective(rule=objective, sense='''Your code here''')solver.solve(model)
In [ ]:
print('*** Solution *** :')print('a:', value(model.a))print('b:', value(model.b))print('sum of squared error:', model.obj())
Part (c) Discussion (2 pts)
In terms of fit, which of the two models is better? Explain your answer.
Your answer here:
Question 3 (22 points)
Alex is a high school student who scored 75 out of 100 points in the math midterm exam, 20 out of 100 in the Spanish midterm exam, and 65 out of 100 in the physics midterm exam. Alex has the option to hire private tutors to improve his exam scores. The table below estimates the score Alex will get in the final exams after attending a certain number of hours of tutor sessions and the rate of tutors for the three subjects respectively. The highest score one can get for each subject is 100 points. We assume that Alex will get the same grade in the final exams, i.e., 75 for math, 20 for Spanish and 65 for physics, if he does not hire tutors at all. Alex is able to spend at most 40 hours on tutoring sessions before the final exam.
Part (a) Formulate the optimization problem (6 pts)
Alex wants to maximize the total score of the three subjects in the final exams and minimize the tuition fees (cost of hiring private tutors). Formulate the problem as a single-objective optimization problem.
Your formulation should clearly specify:
- Decision variables
- Objective function
- Constraints
Your answer here:
Part (b) Re-formulate the optimization problem (10 pts)
Now suppose that Alex wants to maximize the total score of the three subjects and is no longer concerned by tuition fees. Reformulate the problem and approximate the problem by linearizing the problem using piece-wise linear functions.
Your formulation should clearly specify:
- Decision variables
- Objective function
- Constraints
Your answer here:
Part (c) Solve for the optimal solution (4 pts)
Solve the linear programming problem you formulated in Part B. Feel free to use Pyomo and the IPOPT solver or other solvers (e.g. Excel). If you choose to use your own platform or solver, please attach the code or the excel spreadsheet to the end of your .pdf submission.
In [ ]:
'''your code here (if using Python)'''
Part (d) Discussion (2 pts)
Based on your results in the previous section, when would a budget constraint be an obstacle to achieving Alex's goal of maximizing his final exam scores?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Ad Serving Systems Programmatic Advertising This involves using algorithms to automate the buying and selling of ad space You could explore programmin...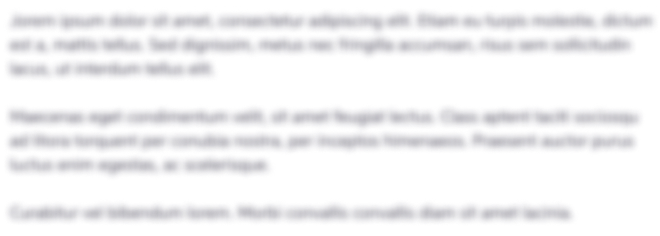
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started