Question
In this problem, you are asked to implement a function to get the player's shots data. In particular, your function should read in data/shot_logs.csv and
In this problem, you are asked to implement a function to get the player's shots data. In particular, your function should read in "data/shot_logs.csv" and filter the dataset to return the player's data.
In [1]:
import pandas as pd
def GetPlayerData(player):
df_shots_player = None
# YOUR CODE HERE
raise NotImplementedError()
return df_shots_player
In [2]:
df_shots_player = GetPlayerData("stephen curry")
assert df_shots_player.shape == (968, 21)
df_shots_player = GetPlayerData("brian roberts")
assert df_shots_player.shape == (372, 21)
Problem 2
You need to implement a function to add a Month column to the existing data, which contains the month from the MATCHUP column.
In [ ]:
def AddMonth(df_shots_player):
d = {'JAN':1, 'FEB':2, 'MAR':3, 'APR':4, 'MAY':5, 'JUN': 6, 'JUL':7,'AUG':8,'SEP':9,'OCT':10,'NOV':11,'DEC':12 }
# YOUR CODE HERE
return df_shots_player
In [ ]:
df_shots_player = GetPlayerData("stephen curry")
df_shots_player = AddMonth(df_shots_player)
assert round(df_shots_player["Month"].describe()["mean"], 2) == 6.65
df_shots_player = AddMonth(GetPlayerData("brian roberts"))
assert round(df_shots_player["Month"].describe()["mean"], 2) == 6.69
Problem 3
You need to implement a function to add a column called "Short_Type" to df_shots_player that identifies the shot type as one of the three that we are interested in: "short" (
In [ ]:
def GetShotType(row):
"""
This function takes a row of the dataset and returns
"3-pointer", "short" or "mid-range" depending on the shot
type.
"""
return_type = "3-pointer"
# YOUR CODE HERE
raise NotImplementedError()
return return_type
def AddShotType(df_shots_player):
# YOUR CODE HERE
raise NotImplementedError()
return df_shots_player
In [ ]:
import pandas as pd
df_shots_player = GetPlayerData("stephen curry")
df_shots_player = AddMonth(df_shots_player)
df_shots_player = AddShotType(df_shots_player)
assert df_shots_player[df_shots_player["Shot_Type"] == "3-pointer"].shape[0] == 456
assert df_shots_player[df_shots_player["Shot_Type"] == "short"].shape[0] == 224
assert df_shots_player[df_shots_player["Shot_Type"] == "mid-range"].shape[0] == 288
Problem 4
You need to implement a function to compute the player's field goal percentage for each month and for each type of shot. Only look at months where he took at least 30 shots in each category. The function should return a dataframe for "stephen curry" as the follows:
In [ ]:
def ComputeGoalType(df_shots_player):
FG_perc_by_month = None
# YOUR CODE HERE
return FG_perc_by_month
In [ ]:
df_shots_player = GetPlayerData("stephen curry")
df_shots_player = AddMonth(df_shots_player)
df_shots_player = AddShotType(df_shots_player)
FG_perc_by_month = ComputeGoalType(df_shots_player)
assert [round(x, 2) for x in FG_perc_by_month["3-pointer"].tolist()] == [0.41, 0.47, 0.43, 0.35]
assert [round(x, 2) for x in FG_perc_by_month["short"].tolist()] == [0.65, 0.65, 0.65, 0.70]
The CSV File is in the shared link
https://drive.google.com/file/d/1scO0JF15YX0K3r17T6oNRBd1VV7wLojz/view?usp=sharing
Problem 1 In this problem, you are asked to implement a function to get the player's shots data. In particular, your function should read in "data/shot_logs.csv" and filter the dataset to return the player's data. In [1]: import pandas as pd def GetPlayerData(player): df_shots_player = None \# YOUR CODE HERE raise NotImplementedError() return df_shots_player In [2]: df_shots_player = GetPlayerData("stephen curry") assert df_shots_player.shape ==(968,21) df_shots_player = GetPlayerData("brian roberts") assert df _shots_player.shape ==(372,21) Problem 2 You need to implement a function to add a Month column to the existing data, which contains the month from the MATCHUP column. You need to implement a function to add a column called "Short_Type" to df_shots_player that identifies the shot type as one of the three that we are interested in: "short" (
Step by Step Solution
There are 3 Steps involved in it
Step: 1
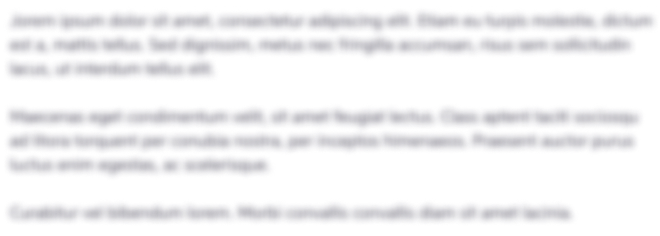
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started